jQuery DOM traversal: Easily control web elements
This article will explore the jQuery DOM traversal method in depth, showing how to use jQuery to easily select web page elements and operate based on their relationship with other elements in the page.
Core points:
- jQuery DOM traversal allows developers to easily navigate and manipulate web page elements and operate relative to the initial selection, including replacing the original selection or adding and removing elements to it.
- jQuery provides multiple methods to filter elements based on the position of elements relative to other elements and whether they have specific classes, etc. Methods include
eq
,first
,last
,slice
,filter
,map
and . -
children
jQuery also provides DOM traversal methods for accessing parent, child, or sibling elements. These methods includefind
,parent
,parents
,closest
,siblings
,prev
,prevAll
,next
,nextAll
, , - ,
add
,addBack
andend
.contents
not
Other jQuery methods related to DOM traversal include ,
,
,and
. These methods help to add more elements to the selection, restore to the previous set of elements, or exclude certain elements from the selection.Element Filtering Let's start with how to filter the selection into a more specific element. You can filter elements based on many conditions, such as their position relative to other elements and whether they have specific classes. In most cases, you will end up selecting fewer elements than you start selecting. The following is a list of different filtering methods:
-
eq
— This method reduces the matching element set to elements located at the index you specified. The index starts from scratch. Therefore, to select the first element, you must use$("selector").eq(0)
. Starting with version 1.4, you can provide a negative integer that counts elements from the end rather than the beginning. -
first
andlast
—first
methods will return only the first element in the matching element set, whilelast
will return the last element in the matching element set. Neither method accepts any parameters. -
slice
— If you are looking for all elements in a collection whose index is within a given range, you can useslice()
. This method accepts two parameters. The first parameter specifies the starting index of where the method should start the slice, and the second parameter specifies the index to which the selection should end. The second parameter is optional, and if omitted, all elements whose index is greater than or equal to the starting index are selected. -
filter
— This method reduces your element set to elements that match the selector or through the conditions set in the function you pass to this method. Here is an example of using a selector:
$("li").filter(":even").css( "font-weight", "bold" );
You can also use the function to select the same element:
$("li") .filter(function( index ) { return index % 2 === 0; }) .css( "font-weight", "bold" );
You can also use this function to perform more complex selections, such as:
.filter(function( index ) { return $( "span", this ).length >= 2; })
This will only select elements with at least two <span></span>
tags.
-
map
— You can use this method to pass each element in the current selection through a function, and finally create a new jQuery object with the return value. The returned jQuery object itself contains an array that you can use theget
method to handle the basic array.
DOM traversal
Consider a situation where you know the selector that you can use to access various elements, but you need to use the parent element of all of them. Furthermore, the parent element does not have any specific class or tags that are common to them. The only thing they have in common is that they are both parent elements of elements you can access. I've encountered similar situations many times.
jQuery provides many useful methods to access parent, child, or sibling elements. Let's introduce them one by one:
-
children
— This method allows us to get child elements of each element in the element set. These child elements can be optionally filtered by selectors. -
find
— This method will get all descendants of each element in the matching element set, which are filtered by selectors or elements. In this case, the selector parameter passed tofind()
is not optional. If you want to get all the descendants, you can pass the universal selector (*
) as a parameter to this method. -
parent
— This method will get the parent element of each element in the current set. The parent element can be optionally filtered using selectors. -
parents
— This method will get all ancestors of each element in the collection. It also accepts an optional selector parameter to filter ancestors. The difference betweenparent()
andparents()
is that the former only traverses one level upwards, whileparents()
traverses upwards to the root element of the document. -
closest
— This method gets the first element that matches the given selector by testing the element itself and then traversing the DOM tree upwards. There are two significant differences betweenparents()
andclosest()
.parents()
starts traversal from the element's parent element, andclosest()
starts traversal from the element itself. Another difference is thatclosest()
only goes through the DOM tree until a match is found, whileparents()
will move up until it reaches the root element of the document. -
siblings
— This method gets the sibling elements of each element in the matching element set. You can optionally provide a selector as a parameter to get only sibling elements with matching selectors. -
prev
— This method will get the exact same element of each element in our collection. If you provide a selector, the method will select the element only if it matches the selector. -
prevAll
— This method will get all precedent elements of each element in our collection. Like other methods, you can provide selectors to filter returned elements. -
next
— This method only gets the same element immediately following the matching element. If a selector is provided, it will only get matching selectors. -
nextAll
— This method will get all subsequent sibling elements of each element in the collection. The sibling elements can be selectively filtered by providing a selector.
More functions related to DOM traversal
When traversing the DOM, you may encounter situations where you need to add more elements that are not related to the original collection to the selection, or you need to restore to the previous set of elements. jQuery provides some functions that you can use to perform all of these tasks.
-
add
— This method will create a new jQuery object that will contain new elements added to the list of existing elements. Remember that there is no guarantee that new elements will be added to the existing collection in the order they are passed to theadd
method. -
addBack
— jQuery maintains an internal stack that tracks changes to element sets. Calling any traversal method will push a new set of elements onto the stack. If you want to use both previous and new element sets, you can use theaddBack
method. -
end
— This method ends the most recent filtering operation and restores your element set to its previous state. It is useful in situations where you want to manipulate certain elements related to the current set of elements, restore to the original set, and then manipulate different sets of elements. -
contents
— If you want to get all elements of all child elements, including text and comment nodes, you can use thecontents
method. You can also use this method to get the content of<iframe></iframe>
(if<iframe></iframe>
is in the same domain as your page). -
not
— If you have a large set of elements and want to select only those subsets of elements that are not that match a given selector, you can use . Starting with version 1.4, the method can also accept a function as an argument to test each element based on certain conditions. Any element that meets these conditions will be excluded from the filtered set.not()
All of these methods in jQuery provide us with an easy way to traverse from one set of elements to another. Since some of these methods are very similar to each other, I suggest you pay special attention to them. Understanding the difference between
and parents()
or closest()
and next("selector")
can save you hours of trouble in some cases. nextAll("selector").eq(0)
jQuery DOM traversal FAQ
What is the meaning of jQuery DOM traversal?
jQuery DOM traversal is a key aspect of web development, which allows developers to easily navigate and manipulate document object models (DOMs). It provides a set of methods that can be used to traverse elements in a web page, making it easier to select specific elements and perform various operations on them. This may include changing the content, style, and even structure of a web page. The ability to traverse the DOM makes jQuery a powerful tool for dynamic web development.
How is jQuery DOM traversal different from traditional JavaScript DOM operations?
While JavaScript provides its own DOM operation method, jQuery DOM traversal simplifies the process and makes it more efficient. It provides a more intuitive and concise syntax that makes the code easier to write and understand. Additionally, jQuery handles many JavaScript-related cross-browser compatibility issues, making your code more robust and reliable.
Can you explain the .parent()
and .children()
methods in jQuery DOM traversal?
The method in jQuery is used to select the direct parent element of an element. For example, if you have a .parent()
element inside the element, using
<div> on <code><div> will select <code>.parent()
. On the other hand, the method is used to select all direct child elements of an element. If you used
.children()
for the element in the previous example, it will select
.children()
. <div>
What is the difference between and <h3> methods in <code>.find()
jQuery? .children()
Although both
and .find()
methods are used to select descendant elements, they work slightly differently. The .children()
method only traverses downwards one level, which means it only selects direct child elements. However, the .children()
method can traverse down multiple levels of the DOM tree, which means it can select all descendants of the element, not just direct child elements. .find()
method in jQuery DOM traversal? .siblings()
The
method in jQuery is used to select all sibling elements of the selected element. A simultaneous element refers to an element that shares the same parent element. For example, if you have multiple elements inside a .siblings()
element, using on one will select all other
<div> elements. <code><div>
<code>.siblings()
What is the purpose of the <div> method in jQuery DOM traversal?
<h3 id="The-code-eq-code-method-in-jQuery-is-used-to-select-elements-with-a-specific-index-number-It-is-especially-useful-when-you-have-multiple-elements-of-the-same-type-and-want-to-select-one-of-them-based-on-their-position-in-the-DOM-The-index-number-starts-at-so"> The <code>.eq()
method in jQuery is used to select elements with a specific index number. It is especially useful when you have multiple elements of the same type and want to select one of them based on their position in the DOM. The index number starts at 0, so will select the first element,
will select the second element, and so on. .eq()
.eq(0)
Can you explain the .eq(1)
and
The .first()
and .last()
methods in
jQuery are used to select the first and last elements of the group, respectively. For example, if you have a set of elements, using .first()
will select the first .last()
in the group, and <div> will select the last <code>.first()
. <div>
<code>.last()
How do I use the <div> method in jQuery DOM traversal?
<h3 id="The-code-filter-code-method-in-jQuery-is-used-to-select-elements-that-meet-certain-conditions-You-can-pass-a-function-to-the">The <code>.filter()
method in jQuery is used to select elements that meet certain conditions. You can pass a function to the method, which will select only the elements that the function returns
. This allows you to create more complex selection criteria and select elements based on the attributes or content of the element. .filter()
What is the purpose of the .not()
method in jQuery DOM traversal?
The .not()
method in jQuery is used to delete elements from a collection. It is the opposite of the .filter()
method. You can pass a selector, function, or jQuery object to the .not()
method, which will remove all elements that match the parameters from the collection.
Can you explain the .has()
method in jQuery DOM traversal?
The method in jQuery is used to select elements with specific descendants. You can pass a selector or jQuery object to the .has()
method, which will select all elements that contain at least one element that matches the parameter. This is useful when you want to select elements based on what the elements are. .has()
The above is the detailed content of A Comprehensive Look at jQuery DOM Traversal. For more information, please follow other related articles on the PHP Chinese website!
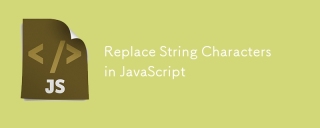
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
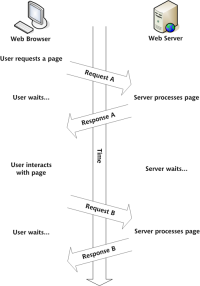
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
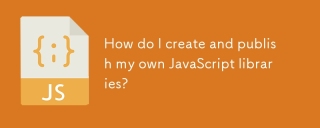
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
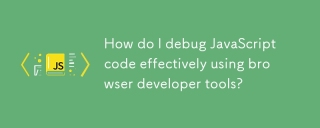
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
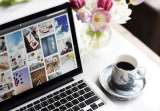
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
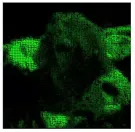
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
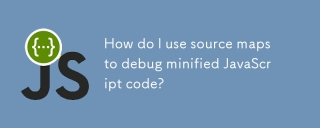
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
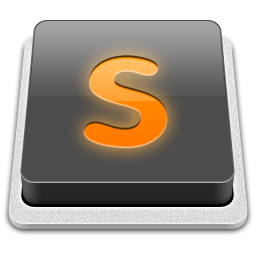
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
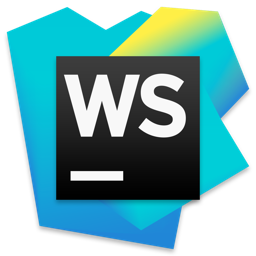
WebStorm Mac version
Useful JavaScript development tools
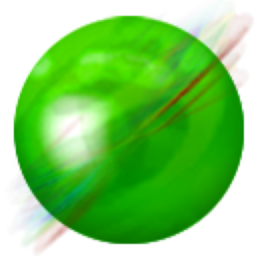
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.