Key Takeaways:
Refactoring JavaScript enhances code readability, maintainability, performance, and reusability, aiding bug detection and correction. Common techniques involve minimizing redundancy using variables, improving event handling, and refining class management for more flexible, adaptable code. Thorough unit testing before and after refactoring is crucial to prevent unintended consequences. While performance gains are possible, prioritizing readability and maintainability is paramount. Refactoring should be an iterative process integrated into the development lifecycle.
This article was peer-reviewed by Dan Prince. Thanks to SitePoint's peer reviewers for their contributions!
A SitePoint forum thread showcased code controlling dropdown visibility. While functional, the code lacked robustness and adaptability. This article demonstrates refactoring techniques for improved reusability and future-proofing.
Original CSS:
#second { display: none; } #second.show { display: block; }
Original JavaScript:
document.getElementById("location").onchange = function () { if (this[this.selectedIndex].value === "loc5") { document.getElementById("second").className = "show"; } else { document.getElementById("second").className = ""; } };
This article refactors this JavaScript code for better maintainability and reusability.
Choosing the Right Refactoring Path:
JavaScript offers multiple solutions; the goal is to improve the code to avoid future rework. Removing redundancy is a starting point, progressing towards more generic, adaptable code. Specific code is often brittle, while generic code handles a wider range of situations. The balance lies in achieving generality without sacrificing readability.
Refactoring: Specific to Generic:
Test-driven development (TDD) highlights a crucial principle: as tests become more specific, the code becomes more generic. This enhances the code's ability to handle diverse scenarios. For the example code, improvements include:
- Using variables to store strings, centralizing management.
- Replacing
onchange
withaddEventListener
for better event handling and preventing overwriting. - Utilizing
classList
instead ofclassName
to manage class names without overwriting existing classes.
These changes enhance resilience to future modifications and simplify updates.
Using Variables to Prevent Duplication:
Storing element IDs and trigger values in variables improves maintainability. Instead of multiple references to the same element, a single variable reference is used, simplifying future modifications.
Improved Code (with variable usage):
var source = document.getElementById("location"); var target = document.getElementById("second"); var triggerValue = "loc5"; source.onchange = function () { var selectedValue = this[this.selectedIndex].value; if (selectedValue === triggerValue) { target.className = "show"; } else { target.className = ""; } };
This refactoring centralizes identifier management.
Improving Event Handling:
Traditional event handlers can be overwritten. addEventListener
allows multiple handlers for the same event, preventing accidental overwriting.
Improved Code (with addEventListener):
#second { display: none; } #second.show { display: block; }
This ensures event handler persistence.
Improving Class Handling:
Using classList.add()
and classList.remove()
prevents overwriting existing classes, maintaining the element's original styling.
Final Refactored Code:
document.getElementById("location").onchange = function () { if (this[this.selectedIndex].value === "loc5") { document.getElementById("second").className = "show"; } else { document.getElementById("second").className = ""; } };
This code is more robust and adaptable.
Conclusion:
Refactoring is a straightforward process. The "specific to generic" principle, often a byproduct of TDD, enhances code flexibility. These techniques reduce the need for repeated code fixes, resulting in more maintainable and reusable JavaScript.
Frequently Asked Questions (FAQs) on JavaScript Refactoring Techniques: (This section remains largely unchanged from the input, as it provides valuable information and doesn't need significant rewriting for the purpose of paraphrasing.)
What are the key benefits of refactoring JavaScript code?
Refactoring JavaScript code offers numerous advantages: improved readability and maintainability, enhanced performance through code optimization, easier bug detection and correction, and increased code reusability.
How can I identify code that needs refactoring?
Signs of code needing refactoring include code duplication, excessively long methods or functions, overly large classes, high complexity, and poor performance. Code analysis tools can assist in identifying problematic areas.
What are some common JavaScript refactoring techniques?
Common JavaScript refactoring techniques encompass extracting methods, renaming variables or functions, removing dead code, simplifying conditional expressions, and replacing temporary variables with direct queries.
How can I ensure that refactoring does not introduce new bugs in the code?
Comprehensive unit testing is crucial. Run tests before and after refactoring to verify functionality remains unaffected.
Can refactoring improve the performance of JavaScript code?
Yes, refactoring can significantly boost performance by eliminating unnecessary code, optimizing functions, and simplifying complex expressions. However, readability and maintainability should remain primary objectives.
Is it necessary to refactor the entire code at once?
No, incremental refactoring, focusing on specific areas or functions, is generally recommended for better manageability and reduced risk.
How can I learn more about JavaScript refactoring techniques?
Numerous online resources are available, including tutorials, blogs, books, and video courses. Practical experience can be gained through coding challenges and open-source projects.
What tools can I use for refactoring JavaScript code?
IDEs like WebStorm and Visual Studio Code offer built-in refactoring features. Code analysis tools such as ESLint and JSHint help identify problematic code sections.
Can refactoring help in reducing the size of JavaScript code?
Yes, refactoring can lead to more concise code, reducing its size and potentially improving web page load times.
Is refactoring a one-time process?
No, refactoring is an ongoing process integrated into the development lifecycle to maintain code quality and efficiency.
The above is the detailed content of JavaScript Refactoring Techniques: Specific to Generic Code. For more information, please follow other related articles on the PHP Chinese website!
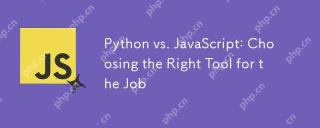
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
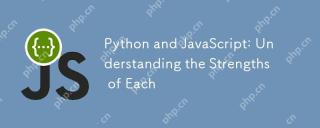
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
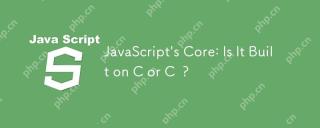
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
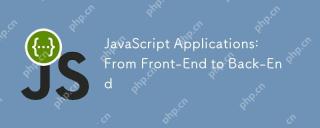
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
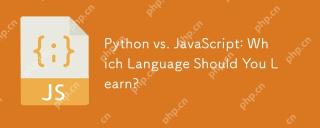
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
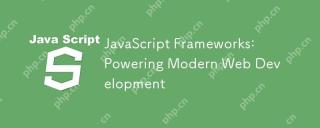
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
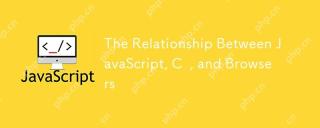
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
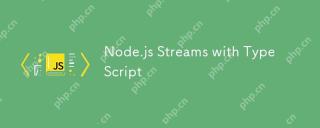
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
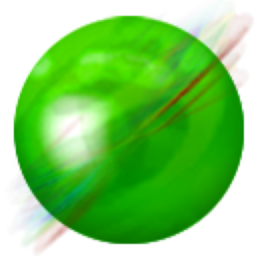
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
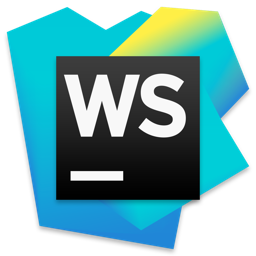
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
