WP_Error class for WordPress: elegant error handling mechanism
WordPress's WP_Error
class provides a simple and powerful error handling mechanism for managing and modifying errors in applications. It is easy to integrate into your application, effectively handles errors returned by WordPress core functions, and creates custom error messages to improve the user experience. In addition, practical functions such as is_wp_error
can check whether the variable or function return value is an instance of WP_Error
, thereby debugging problems efficiently.
No matter how skillful and meticulous your code is written, errors cannot be avoided in any development process. As a WordPress developer, it is your responsibility to make sure all code errors are handled correctly without affecting the end user. WordPress comes with a basic error handling class WP_Error
, which can be integrated into your code for basic error handling.
This tutorial will explore the basic structure and working principles of WP_Error
classes, and most importantly, we will introduce how to integrate WP_Error
classes in your application.
WP_Error
Detailed explanation of the category
WP_Error
The class has a simple structure but powerful function, which is enough to be used as an error handling mechanism for plug-ins. Its source code is located in the wp-includes/class-wp-error.php
file. Let's take a look at its properties and methods.
Properties
WP_Error
There are only two private attributes: $errors
and $error_data
. $errors
is used to store related error messages, while $error_data
is optionally used to store relevant data you want to access later. WP_Error
Use simple key-value pairs to store related errors and data into an object, so the keys defined in WP_Error
must be unique to avoid overwriting previously defined keys.
Method
WP_Error
Provides several ways to modify the two properties it contains. Let's look at a few:
-
get_error_codes()
: Returns all available error codes for a specificWP_Error
instance. If only the first error code is needed, another separate function is available.get_error_code()
- : If
get_error_messages( $code )
is not provided, the function will simply return all messages stored in the specific$code
instance. Similarly, if you only need to return a message with a specific error code, just useWP_Error
.get_error_message( $code )
- : This function is especially useful when you want to modify errors stored in instantiated
add( $code, $message, $data )
objects. Note that even ifWP_Error
and$message
are not required, the$data
variables will still be filled.$errors
- : If you only want to modify the
add_data( $data, $code)
property, you can use this function. Note that the$error_data
parameter is in the second position, opposite to the$code
method. Ifadd
is not provided, the error data will be added to the first error code.$code
- : This is a new method recently added in WordPress 4.1, which removes all error messages and data associated with a specific key.
remove( $code )
How do you know if the specific variable or data returned by a function is an instance of
? You can check using a utility function WP_Error
which returns true or false based on the given variable. is_wp_error()
- : Return true if
is_wp_error( $thing )
is an instance of$thing
, otherwise return false.WP_Error
WP_Error
Just just understand how works internally, you also need to learn how to implement it well in your own application. Let's take a few examples to better understand how it works. WP_Error
WordPress provides many practical functions that can be used to speed up our development process. Most functions are also equipped with basic error handling functions that we can use.
For example,
is a very useful function that we can use to make a remote POST request to a specific URL. However, we cannot expect the remote URL to be always accessible, or our requests are always successful. We know from the manual page that this function will return wp_remote_post
when it fails. This knowledge will help us to correctly implement error handling in our application. WP_Error
// 向远程 URL $url 发出请求 $response = wp_remote_post( $url, array( 'timeout' => 30, 'body' => array( 'foo' => 'bar' ) ) ); if ( is_wp_error( $response ) ) { echo 'ERROR: ' . $response->get_error_message(); } else { // 执行某些操作 }As you can see, we are performing a remote POST request to
. However, instead of simply getting the $url
data as is, we do some checks using the convenient $response
function introduced earlier. If everything works, we can continue to do what we want to do with is_wp_error
. $response
Return custom error in your application
Suppose you have a custom function that handles the submission of a contact form, named handle_form_submission
. Suppose we set up a custom form somewhere, let's see how we can improve the function by implementing our own error handling capabilities.
// 向远程 URL $url 发出请求 $response = wp_remote_post( $url, array( 'timeout' => 30, 'body' => array( 'foo' => 'bar' ) ) ); if ( is_wp_error( $response ) ) { echo 'ERROR: ' . $response->get_error_message(); } else { // 执行某些操作 }
Of course, you also need to implement your own cleaning and verification in these functions, but this is not within the scope of this tutorial. Now, knowing that we correctly return the WP_Error
instance when we are error-based, we can use it to provide more meaningful error messages to the end user.
Suppose there is a specific part in your application that displays form submission errors, you can do this:
function handle_form_submission() { // 在此处执行你的验证、nonce 等操作 // 实例化 WP_Error 对象 $error = new WP_Error(); // 确保用户提供名字 if ( empty( $_POST['first_name'] ) ) { $error->add( 'empty', 'First name is required' ); } // 也需要姓氏 if ( empty( $_POST['last_name'] ) ) { $error->add( 'empty', 'Last name is required' ); } // 检查电子邮件地址 if ( empty( $_POST['email'] ) ) { $error->add( 'empty', 'Email is required' ); } elseif ( ! is_email( $_POST['email'] ) ) { $error->add( 'invalid', 'Email address must be valid' ); } // 最后,检查消息 if ( empty( $_POST['message'] ) ) { $error->add( 'empty', 'Your message is required' ); } // 发送结果 if ( empty( $error->get_error_codes() ) ) { return true; // 没有错误 } // 有错误 return $error; }
Summary
Striving to excel in software development also means knowing what to do when your code cannot do what it should do, and making sure your application can handle it gracefully.
For WordPress, using the included WP_Error
class provides a fairly simple but powerful error handling implementation that you can integrate into your application.
(The external references and FAQ sections in the original text are omitted here because they are direct copies of the original text and do not meet the requirements of pseudo-originality.)
The above is the detailed content of An Introduction to the WP_Error Class. For more information, please follow other related articles on the PHP Chinese website!

WordPressisapowerfulCMSwithsignificantadvantagesandchallenges.1)It'suser-friendlyandcustomizable,idealforbeginners.2)Itsflexibilitycanleadtositebloatandsecurityissuesifnotmanagedproperly.3)Regularupdatesandperformanceoptimizationsarenecessarytomainta

WordPressexcelsineaseofuseandadaptability,makingitidealforbeginnersandsmalltomedium-sizedbusinesses.1)EaseofUse:WordPressisuser-friendly.2)Security:Drupalleadswithstrongsecurityfeatures.3)Performance:GhostoffersexcellentperformanceduetoNode.js.4)Scal

Yes,youcanuseWordPresstobuildamembershipsite.Here'show:1)UsepluginslikeMemberPress,PaidMemberSubscriptions,orWooCommerceforusermanagement,contentaccesscontrol,andpaymenthandling.2)Ensurecontentprotectionwithupdatedpluginsandadditionalsecuritymeasures

You don't need programming knowledge to use WordPress, but mastering programming can improve the experience. 1) Use CSS and HTML to adjust the theme style. 2) PHP knowledge can edit topic files and add functions. 3) Custom plug-ins and meta tags can optimize SEO. 4) Pay attention to backup and use of sub-topics to prevent update issues.

TosecureaWordPresssite,followthesesteps:1)RegularlyupdateWordPresscore,themes,andpluginstopatchvulnerabilities.2)Usestrong,uniquepasswordsandenabletwo-factorauthentication.3)OptformanagedWordPresshostingorsecuresharedhostingwithawebapplicationfirewal

WordPressexcelsoverotherwebsitebuildersduetoitsflexibility,scalability,andopen-sourcenature.1)It'saversatileCMSwithextensivecustomizationoptionsviathemesandplugins.2)Itslearningcurveissteeperbutofferspowerfulcontroloncemastered.3)Performancecanbeopti
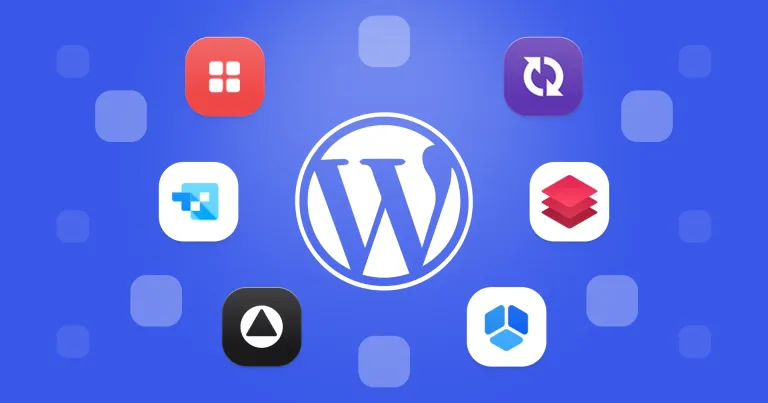
Seven Must-Have WordPress Plugins for 2025 Website Development Building a top-tier WordPress website in 2025 demands speed, responsiveness, and scalability. Achieving this efficiently often hinges on strategic plugin selection. This article highlig

WordPresscanbeusedforvariouspurposesbeyondblogging.1)E-commerce:WithWooCommerce,itcanbecomeafullonlinestore.2)Membershipsites:PluginslikeMemberPressenableexclusivecontentareas.3)Portfoliosites:ThemeslikeAstraallowstunninglayouts.Ensuretomanageplugins


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
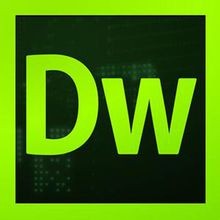
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
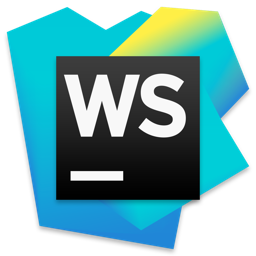
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
