Use JavaScript to dynamically add and delete CSS classes to easily manipulate web elements! This article will explain how to use the className
and classList
attributes of JavaScript to efficiently manage CSS classes, and realize dynamic modification of web page elements, such as displaying/hiding menus, highlighting form errors or element animations.
Core points:
- JavaScript can be used to dynamically modify page elements based on user interactions (such as showing/hiding menus, highlighting form errors, or animation effects).
-
className
andclassList
properties are powerful tools for operating CSS classes;className
have wider compatibility, whileclassList
is more modern and convenient. - can create functions and add or delete classes to elements using
className
orclassList
attributes. These functions can take a string selector or element itself as parameters, loop through the element and add or delete the specified class.
The -
classList
attribute (supported by browsers in IE10 and above) provides a series of methods to operate classes, includingadd
,remove
andtoggle
, simplifying the process of adding or deleting classes.
Compatibility scheme: Use className
Properties
className
attribute allows access to the class attribute of the HTML element. Through string operations, we can add and delete classes. We will use the querySelectorAll()
method to select HTML elements (compatible with IE8 and above browsers).
Add class:
function addClass(elements, myClass) { if (!elements) return; if (typeof elements === 'string') elements = document.querySelectorAll(elements); else if (elements.tagName) elements = [elements]; for (let i = 0; i < elements.length; i++) { if (!(' ' + elements[i].className + ' ').includes(' ' + myClass + ' ')) { elements[i].className += ' ' + myClass; } } }
Delete class:
function removeClass(elements, myClass) { if (!elements) return; if (typeof elements === 'string') elements = document.querySelectorAll(elements); else if (elements.tagName) elements = [elements]; const reg = new RegExp('(^| )' + myClass + '($| )', 'g'); for (let i = 0; i < elements.length; i++) { elements[i].className = elements[i].className.replace(reg, ' '); } }
Modernization Solution: Use classList
Properties
IE10 and above browsers support classList
attributes, simplifying the operation of classes.
Add class:
function addClass(selector, myClass) { const elements = document.querySelectorAll(selector); elements.forEach(element => element.classList.add(myClass)); }
Delete class:
function removeClass(selector, myClass) { const elements = document.querySelectorAll(selector); elements.forEach(element => element.classList.remove(myClass)); }
Summary
This article introduces methods to add and delete CSS classes using className
and classList
properties. By mastering these skills, you can easily achieve dynamic effects of web elements and improve user experience.
FAQs (FAQs)
-
Purpose of adding or removing CSS classes using Vanilla JS? Dynamically controls the appearance and behavior of HTML elements, such as changing styles in response to user operations, showing/hiding elements, creating animation effects, etc.
-
How to add multiple classes using
classList.add()
? Pass the class name as an independent parameter:element.classList.add("class1", "class2", "class3");
-
Can you delete classes that do not exist on the element? Yes,
classList.remove()
will not report an error, but will not perform any operation. -
How to check if an element contains a specific class? Use the
classList.contains()
method to return a boolean value. -
How to manipulate classes with multiple elements at the same time? Use
querySelectorAll()
to select elements, and then loop through and manipulate the classes of each element. -
How to switch the class of an element? Use
classList.toggle()
method. -
The difference between
className
andclassList
?className
is a string attribute;classList
is a read-only attribute that returns theDOMTokenList
object, providing a more convenient class operation method. -
classList
Does SVG elements be supported? Support. -
How is the browser compatibility of
classList
? Modern browsers are widely supported, and IE9 and below are not supported. -
Can the
classList
method be called in a chain? Yes.
I hope this article will be helpful to you!
The above is the detailed content of Quick Tip: Add or Remove a CSS Class with Vanilla JavaScript. For more information, please follow other related articles on the PHP Chinese website!
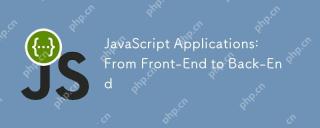
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
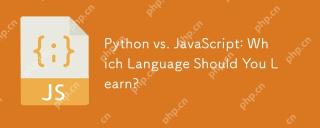
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
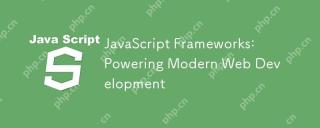
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
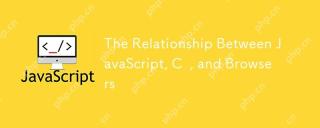
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
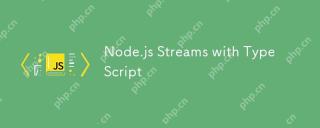
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
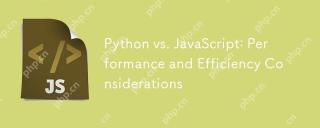
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
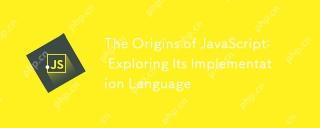
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
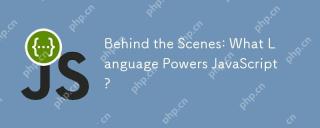
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
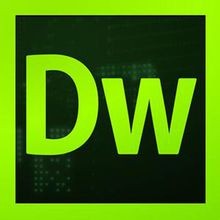
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
