This article provides a practical introduction to object-oriented programming (OOP) in Python. We'll focus on demonstrating OOP concepts rather than delving into complex theoretical details. Python's popularity, ranking fourth among developers according to Statista, highlights its versatility and simplified syntax, making it an excellent choice for learning OOP.
Key Concepts:
- Python's OOP Strengths: Python's straightforward syntax and adaptability make it ideal for implementing OOP principles. This tutorial emphasizes practical application.
- Classes and Objects: Classes serve as blueprints, defining the structure and behavior of objects. Objects are instances of classes, possessing attributes (data) and methods (functions).
- Advanced OOP Features: We'll explore inheritance, polymorphism, method overloading, and overriding – crucial for building efficient and reusable code.
Fundamentals of OOP:
OOP is a programming paradigm—a set of guidelines for structuring code. It models systems using objects, each with specific functions and behaviors. Objects contain data and methods (procedures acting on data, potentially using parameters). Languages like Java, C , C#, Go, and Swift utilize OOP, each with its own implementation.
Classes and Objects:
Consider two dogs, Max and Pax. They are both instances (objects) of the "dog" concept. The "dog" concept itself is modeled using a class. A class defines the template (attributes and methods) for creating objects.
Here's Python code illustrating this:
class Dog: def __init__(self, name, breed): self.name = name self.breed = breed def __repr__(self): return f"Dog(name={self.name}, breed={self.breed})" max = Dog("Max", "Golden Retriever") pax = Dog("Pax", "Labrador") print(max) print(pax)
The __init__
method (constructor) initializes the object's state. self
refers to the current object instance. The __repr__
method provides a string representation of the object.
Defining New Methods:
To add functionality, define methods within the class. For example, a get_nickname
method:
class Dog: # ... (previous code) ... def get_nickname(self): return f"{self.name}, the {self.breed}" # ... (rest of the code) ...
Access Modifiers:
Python uses naming conventions (single underscore _
for protected, double underscore __
for private) to suggest access restrictions, but doesn't enforce them strictly like some other languages. It's best practice to use getter and setter methods for controlled access to attributes.
Inheritance:
Inheritance promotes code reuse. A subclass inherits attributes and methods from a superclass (parent class).
Example: Person
(parent) and Student
, Professor
(children):
class Dog: def __init__(self, name, breed): self.name = name self.breed = breed def __repr__(self): return f"Dog(name={self.name}, breed={self.breed})" max = Dog("Max", "Golden Retriever") pax = Dog("Pax", "Labrador") print(max) print(pax)
The super().__init__
call in subclasses invokes the parent class's constructor.
Polymorphism:
Polymorphism allows objects of different classes to respond to the same method call in their own specific way.
Method Overloading and Overriding:
Method overloading (having multiple methods with the same name but different parameters) is not directly supported in Python in the same way as in some other languages. Method overriding, where a subclass provides a different implementation of a method from its superclass, is supported.
Conclusion:
This article provided a practical overview of OOP in Python. Understanding classes, objects, inheritance, and polymorphism is crucial for writing well-structured, reusable, and maintainable Python code. Further exploration of advanced OOP concepts and design patterns will enhance your programming skills.
(FAQs section omitted for brevity, as it's a repetition of information already covered in the article.)
The above is the detailed content of Object-oriented Programming in Python: An Introduction. For more information, please follow other related articles on the PHP Chinese website!
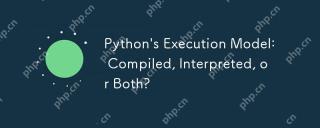
Pythonisbothcompiledandinterpreted.WhenyourunaPythonscript,itisfirstcompiledintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).Thishybridapproachallowsforplatform-independentcodebutcanbeslowerthannativemachinecodeexecution.
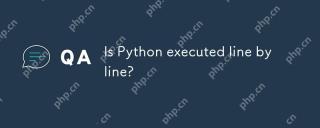
Python is not strictly line-by-line execution, but is optimized and conditional execution based on the interpreter mechanism. The interpreter converts the code to bytecode, executed by the PVM, and may precompile constant expressions or optimize loops. Understanding these mechanisms helps optimize code and improve efficiency.
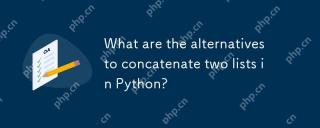
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
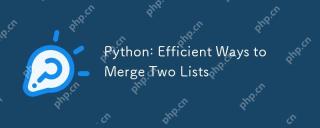
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
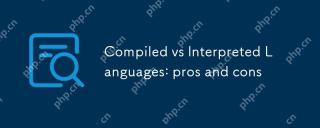
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
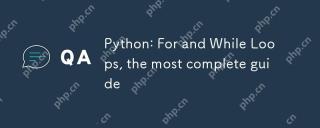
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
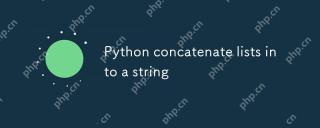
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
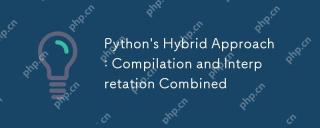
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
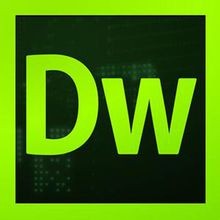
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
