Streamline Your Laravel Workflow with Elixir: A Comprehensive Guide
Many web developers utilize various tools to enhance their workflow and maintain lean codebases. However, tools requiring compilation, such as CSS and JavaScript preprocessors, can sometimes slow down the process. This is where task runners like Gulp and Elixir shine. This tutorial explores Elixir, a user-friendly Node.js tool built by Jeffrey Way, designed to simplify Gulp task management, particularly within the Laravel framework.
This guide focuses on asset compilation in Laravel, but also touches on its broader applicability.
Key Concepts:
- Elixir streamlines asset compilation by wrapping Gulp tasks within a clean Node.js interface.
- Installation necessitates Node.js, Gulp, and the
laravel-elixir
package (via npm). - Elixir uses default source and output paths, but these are customizable via method arguments or a configuration object.
- Built-in support includes CSS preprocessor compilation, JavaScript processing, and asset versioning.
- Custom tasks are easily created using Elixir's API, and it integrates seamlessly with Laravel's Blade templating.
- Elixir automates and streamlines the workflow, supporting various CSS and JavaScript preprocessors, boosting Laravel development efficiency.
Prerequisites and Installation:
- Node.js: Essential as Gulp runs within Node.js. Homestead Improved users already have this.
-
Gulp: Elixir relies on Gulp. Install globally using npm:
npm install --global gulp
(unless already installed via Homestead Improved). -
Laravel Elixir: Laravel projects typically include
laravel-elixir
inpackage.json
. Install usingnpm install
within your project's root directory. For non-Laravel environments:npm install laravel-elixir --save
Before You Begin:
Elixir assumes source files (.less
, .sass
, .coffee
, etc.) reside in ./resources/assets/
, with output to ./public/
by default.
File Type | Source Path | Output Path |
---|---|---|
Less | ./resources/assets/less |
./public/css |
Sass | ./resources/assets/sass |
./public/css |
CoffeeScript | ./resources/assets/coffee |
./public/js |
Elixir tasks are defined within your gulpfile.js
using the elixir
function, which takes a callback with a mix
object (providing all available methods).
elixir(function(mix) { mix.less('styles.less'); });
Passing arrays or wildcards compiles and concatenates multiple files into app.css
or app.js
. Single filenames result in identically named output files. These defaults are customizable.
Practical Examples:
- Compiling Less:
elixir(function(mix) { mix.less("styles.less"); });
Compiles resources/assets/less/styles.less
to public/css/styles.css
. Sass compilation uses mix.sass()
. Elixir handles vendor prefixes.
- Compiling CoffeeScript:
elixir(function(mix) { mix.coffee(['controllers.coffee', 'services.coffee']); });
Compiles CoffeeScript files from resources/assets/coffee/
to public/js/app.js
.
Advanced Techniques:
-
Multiple Files:
sass()
,less()
,coffee()
accept single files, wildcards, arrays, or no arguments (for all files in the default directory). -
Custom Source/Output Paths:
- Arguments: A second argument specifies the output directory:
elixir(function(mix) { mix.less(['file1.less', 'file2.less'], 'custom/path'); });
-
Full Paths: Prefix with
./
to specify paths relative to the project root. -
Config Object: The preferred method is modifying
css.output
andjs.output
in Elixir'sconfig
object (discussed later).
-
Concatenation: Use
scripts()
for JavaScript andstyles()
for CSS. These accept arguments for source and output paths, similar to compilation methods.scriptsIn()
andstylesIn()
concatenate all files within a specified directory. -
Jade to Blade: Requires
laravel-elixir-jade
(npm install laravel-elixir-jade@0.1.8 --save-dev
). Thejade()
method compiles Jade to Blade templates. -
File Versioning:
mix.version()
appends a hash to filenames, preventing caching issues. Use theelixir()
helper in Blade templates to reference the versioned files. -
Configuration: Elixir's behavior is controlled via the
config
object innode_modules/laravel-elixir/Config.js
. Override defaults by modifying theelixir.config
object ingulpfile.js
or creating anelixir.json
file in your project root. -
Custom Tasks: Extend Elixir's functionality using the
extend
method to create custom Gulp tasks.
A Real-World Scenario (Laravel/Angular):
This section details a complete example demonstrating Elixir's capabilities in a Laravel/Angular project. It covers configuring Elixir, compiling Less and CoffeeScript, compiling Jade to Blade, and versioning assets. The complete gulpfile.js
is provided, showcasing method chaining.
Running Tasks:
Run gulp
to execute all Elixir tasks. gulp watch
monitors files for changes and automatically runs the tasks. Individual tasks can be run (e.g., gulp less
). Use gulp --production
for minification.
Conclusion:
Elixir simplifies Gulp task management, providing a user-friendly interface for common asset compilation tasks. Its flexibility, customizability, and integration with Laravel make it a valuable tool for streamlining development workflows. This guide provides a comprehensive overview, but further exploration of Elixir's documentation is encouraged for advanced functionalities.
The above is the detailed content of Meet Elixir, the Laravel Way of Compiling Assets. For more information, please follow other related articles on the PHP Chinese website!
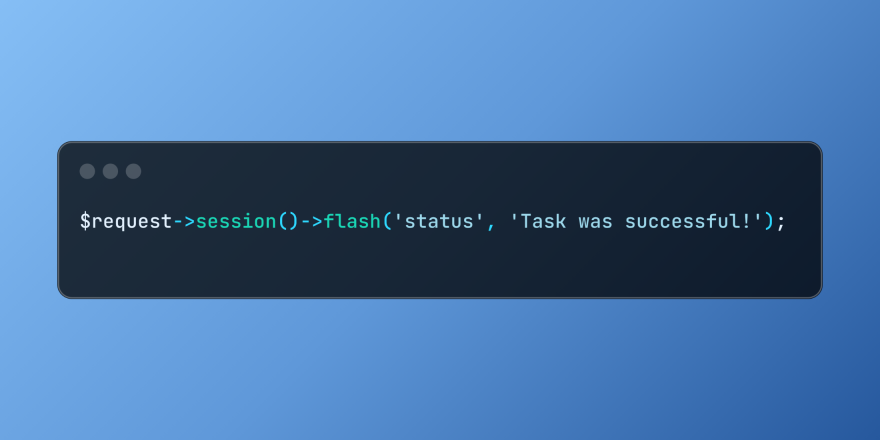
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
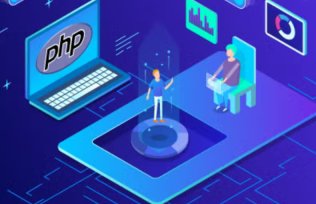
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
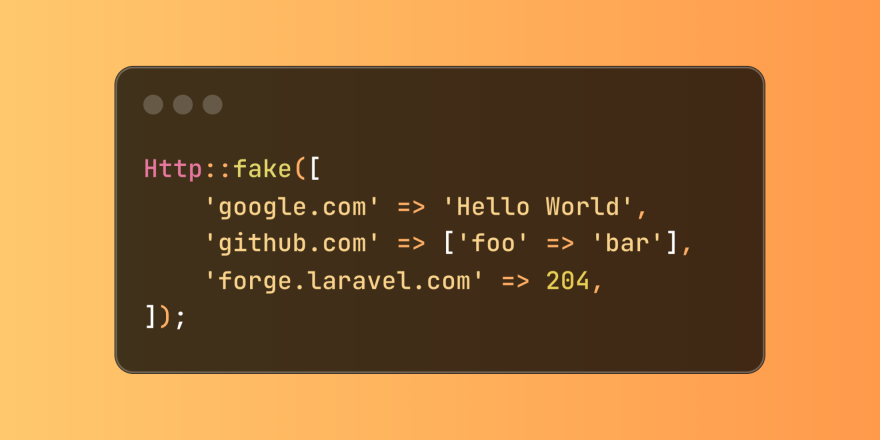
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
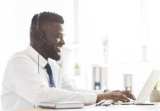
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
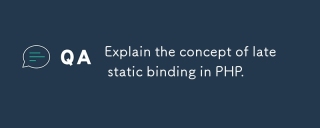
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
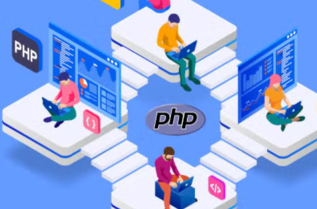
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
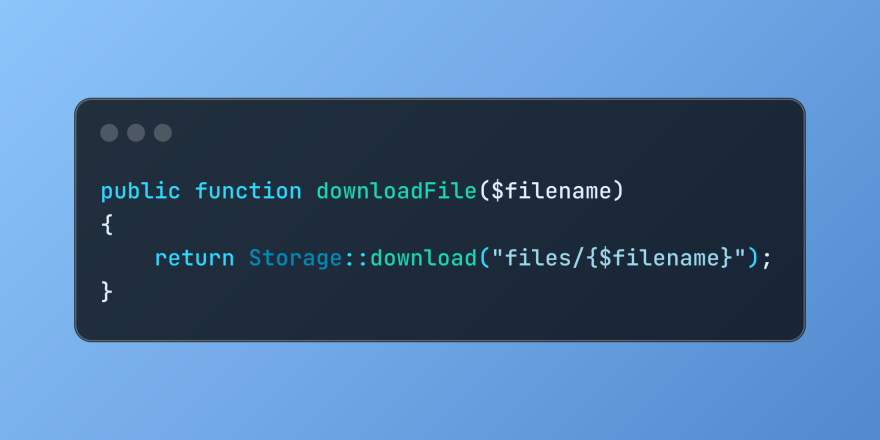
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
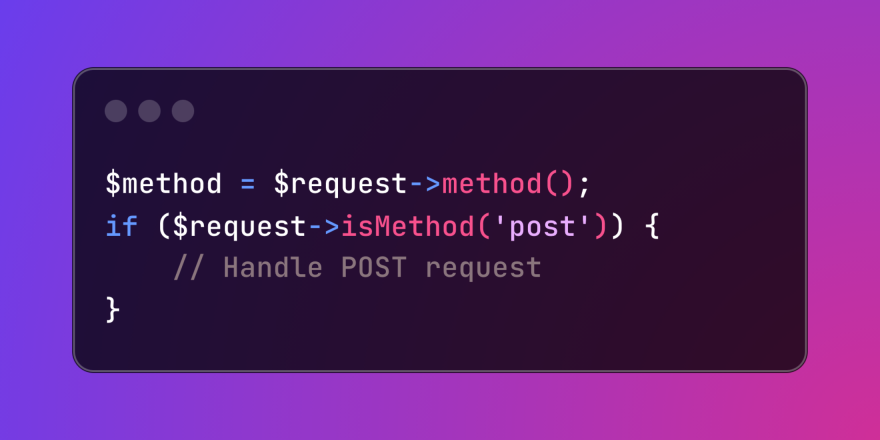
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
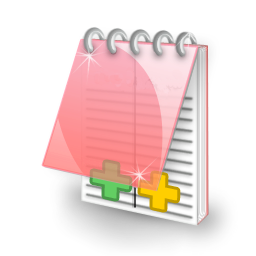
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
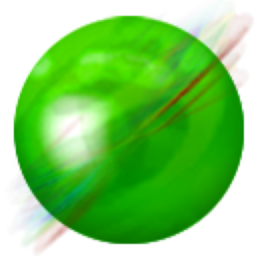
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
