Key Points of JavaScript Observer Pattern
- Observer pattern in JavaScript allows one-to-many data binding between elements, which is especially useful for keeping multiple elements synchronized with the same data.
- Observer pattern contains three main methods:
subscribe
(add new observable events),unsubscribe
(remove all events using bound data).broadcast
Using ES6 features such as classes, arrow functions, and constants can effectively implement observer patterns, making the code simpler and easier to reuse. - Observer mode can be used to solve practical problems in JavaScript, such as updating word counts in blog posts at each keystroke and can be further enhanced to build new features.
Event Observer
The advanced view of this mode is as follows:
<code>EventObserver │ ├── subscribe: 添加新的可观察事件 │ ├── unsubscribe: 移除可观察事件 │ └── broadcast: 使用绑定数据执行所有事件</code>After elaborating on the Observer mode, I will add a word counting feature that uses it. The Word Count component will use this observer and integrate everything together. To initialize
, do the following: EventObserver
class EventObserver { constructor() { this.observers = []; } }Start with an empty list of observation events, and each new instance does so. From now on, let's add more ways to perfect the design pattern in
. EventObserver
subscribe method
To add a new event, do the following:
subscribe(fn) { this.observers.push(fn); }Get the list of observation events and push new items into the array. The event list is a callback function list. One way to test this method in pure JavaScript is as follows:
<code>EventObserver │ ├── subscribe: 添加新的可观察事件 │ ├── unsubscribe: 移除可观察事件 │ └── broadcast: 使用绑定数据执行所有事件</code>
I use Node assertion to test this component in Node. The exact same assertion also exists in the Chai assertion. Note that the observation event list consists of simple callbacks. We then check the length of the list and assert that the callback is in the list.
unsubscribe method
To remove an event, do the following:
class EventObserver { constructor() { this.observers = []; } }
Filter out anything that matches the callback function from the list. If there is no match, the callback will remain in the list. The filter returns a new list and reassigns the observer list. To test this good method, do the following:
subscribe(fn) { this.observers.push(fn); }
The callback must match the same function on the list. If a match exists, the unsubscribe
method removes it from the list. Note that the test uses function reference to add and remove it.
broadcast method
To call all events, do the following:
// Arrange const observer = new EventObserver(); const fn = () => {}; // Act observer.subscribe(fn); // Assert assert.strictEqual(observer.observers.length, 1);
This will iterate over the list of observation events and execute all callbacks. With this you can get the one-to-many relationship you need to subscribe to events. You can pass in the data
parameter, which makes the callback data binding. ES6 uses arrow functions to make the code more efficient. Note that the (subscriber) => subscriber(data)
function does most of the work. This single-line arrow function benefits from this short ES6 syntax. This is a significant improvement in the JavaScript programming language. To test this broadcast
method:
unsubscribe(fn) { this.observers = this.observers.filter((subscriber) => subscriber !== fn); }
Use let
instead of const
so that we can change the value of the variable. This makes the variable mutable, allowing me to reassign its value in the callback. Use let
in your code to signal to other programmers that the variable is changing at some point. This increases the readability and clarity of JavaScript code. This test gives me enough confidence to make sure the observer works as expected. With TDD, it's all about building reusable code in pure JavaScript. There are many benefits to writing testable code in pure JavaScript. Test everything and keep what is beneficial for code reuse. With this, we have perfected it. The question is, what can you build with it? EventObserver
Practical application of observer mode: blog word count demonstration
For the demo, it's time to create a blog post that keeps word count for you. Each keystroke you enter will be synchronized by the Observer Design Pattern. Think of it as free text input, where each event triggers an update to where you need it to go. To get word count from free text input, you can do the following:
// Arrange const observer = new EventObserver(); const fn = () => {}; observer.subscribe(fn); // Act observer.unsubscribe(fn); // Assert assert.strictEqual(observer.observers.length, 0);Completed! There is a lot of content in this seemingly simple pure function, so how about a simple unit test? In this way, my intentions are clear:
<code>EventObserver │ ├── subscribe: 添加新的可观察事件 │ ├── unsubscribe: 移除可观察事件 │ └── broadcast: 使用绑定数据执行所有事件</code>
Please note the slightly weird input string in blogPost
. My goal is to have this function cover as many edge cases as possible. As long as it gives me a correct word count, we go in the right direction. By the way, this is the real power of TDD. This implementation can be iterated and covers as many use cases as possible. Unit tests tell you how I expect it to behave. If the behavior is flawed, it is easy to iterate and adjust it for whatever reason. By testing, leave enough evidence for others to make the changes. It's time to connect these reusable components to the DOM. This is the part you take pure JavaScript and solder it into your browser. One way is to use the following HTML on the page:
class EventObserver { constructor() { this.observers = []; } }
Then there is the following JavaScript:
subscribe(fn) { this.observers.push(fn); }
Get all reusable code and set observer design mode. This will track changes in the text area and give you word count below it. I'm using body.appendChild()
in the DOM API to add this new element. Then, an event listener is attached to bring it to life. Note that using arrow functions can connect a single line of events. In fact, you can use it to broadcast event-driven changes to all subscribers. () => blogObserver.broadcast()
Most of the work was done here. It even passes the latest changes to the text area directly into the callback function. Yes, client scripts are pretty cool. None of the demos you can touch and adjust is complete, here is the CodePen: (The CodePen link should be inserted here, which cannot be provided due to the inability to access external websites)
Now, I won't call this feature a full feature. But this is just the starting point for the observer's design pattern. The question in my mind is, how far are you willing to go?
Looking forward
You can develop this idea further. You can use the Observer Design Pattern to build many new features. You can enhance the demo using the following methods:
- Another component calculates the number of paragraphs
- Another component displays a preview of the input text
- Enhanced previews with markdown support, e.g.
These are just some ideas you can dig into. The above enhancements will challenge your programming capabilities.
Conclusion
Observer design pattern can help you solve practical problems in JavaScript. This solves the long-term problem of keeping a bunch of elements in sync with the same data. Typically, when the browser triggers a specific event. I believe most of you have had this problem now and have turned to tools and third-party dependencies. This design pattern allows you to go as far as possible. In programming, you abstract the solution into patterns and build reusable code. The benefits this will bring to you are endless. I hope you can see how much work you can do in pure JavaScript with just a little discipline and effort. New features in the language, such as ES6, can help you write some concise and reusable code.
(The FAQ for JavaScript Observer Pattern should be included here, but due to space limitations, it has been omitted.)
The above is the detailed content of JavaScript Design Patterns: The Observer Pattern. For more information, please follow other related articles on the PHP Chinese website!
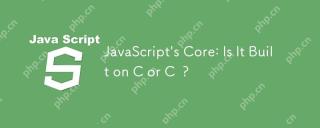
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
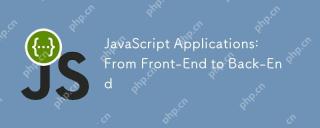
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
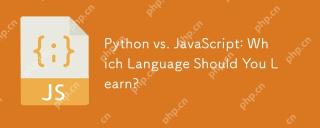
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
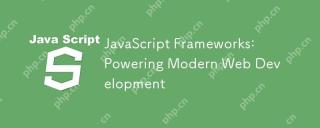
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
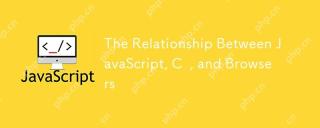
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
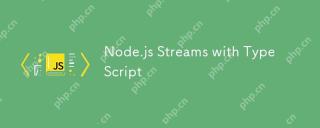
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
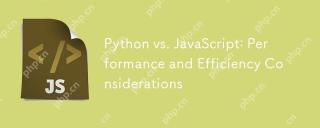
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
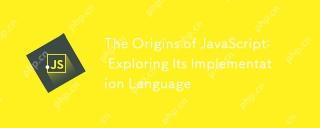
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
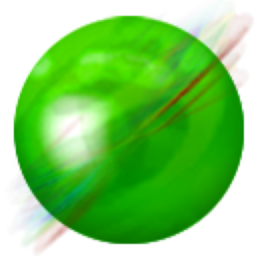
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
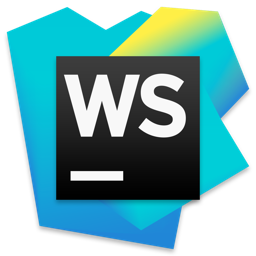
WebStorm Mac version
Useful JavaScript development tools
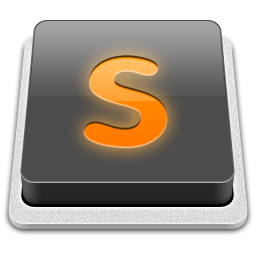
SublimeText3 Mac version
God-level code editing software (SublimeText3)
