Real-time form verification: subtle improvements to enhance user experience
Core points:
- JavaScript can be used to implement real-time form verification, which provides users with instant feedback on input validity, thereby improving user experience and maintaining data integrity, ensuring that only valid data is submitted.
- HTML5 attributes
pattern
andrequired
can be used to define the valid input range of form elements. If the browser does not support these properties, its values can be used as the basis for JavaScript compatibility populators.
The -
aria-invalid
attribute can be used to indicate whether the field is invalid. This property provides accessibility information and can be used as a CSS hook to visually indicate an invalid field. - JavaScript function
instantValidation()
Test the field and perform actual verification, controlling thearia-invalid
attribute to indicate the status of the field. This function can be bound to theonchange
event to provide real-time form validation.
HTML5 introduces several new properties for implementing browser-based form validation. The pattern
property is a regular expression that defines valid input ranges for text area elements and most input types. required
attribute specifies whether the field is required. For older browsers that do not support these properties, we can use their values as the basis for compatibility fillers. We can also use them to provide more interesting enhancements – real-time form validation.
It should be noted that you do not overuse verification, so as not to disrupt normal browsing behavior and hinder user operations. For example, I have seen some forms that cannot leave invalid fields using the Tab key - JavaScript is used (more precisely, abused) to force focus to stay within the field until it is valid. This is very unfavorable to the user experience and directly violates the accessibility guidelines.
This article will introduce a less invasive implementation method. It's not even full client validation - it's just a slight user experience enhancement implemented in an accessible way, and when I tested the script it found it was almost the same as Firefox's current native implementation!
Basic Concept
In the latest version of Firefox, if the required field is empty or its value does not match the pattern, the field will display a red border as shown in the following figure:
Of course, this won't happen immediately. If this happens, the border will be displayed by default for each required field. Instead, these borders are displayed only after you interact with the field, which is basically (although not exactly) similar to the onchange
event.
Therefore, we will use onchange
as the trigger event. Alternatively, we can use the oninput
event, which will fire as long as we type or paste any value in the field. But this is really too "instant" because it can easily trigger repeatedly while typing in quick succession, resulting in a flickering effect, which can get bored or distracted by some users. And, anyway, oninput
does not trigger from the programming input, and onchange
will trigger, we may need it to handle operations such as autocomplete from third-party plugins.
Define HTML and CSS
Let's take a look at our implementation, starting with the HTML it is based on:
<form action="#" method="post"> <fieldset> <legend><strong>Add your comment</strong></legend> <p> <label for="author">Name <abbr title="Required">*</abbr></label> <input aria-required="true" id="author" name="author" pattern="^([- \w\d\u00c0-\u024f]+)$" required="required" size="20" spellcheck="false" title="Your name (no special characters, diacritics are okay)" type="text" value="" > </p> <p> <label for="email">Email <abbr title="Required">*</abbr></label> <input aria-required="true" id="email" name="email" pattern="^(([-\w\d]+)(\.[-\w\d]+)*@([-\w\d]+)(\.[-\w\d]+)*(\.([a-zA-Z]{2,5}|[\d]{1,3})){1,2})$" required="required" size="30" spellcheck="false" title="Your email address" type="email" value="" > </p> <p> <label for="website">Website</label> <input id="website" name="website" pattern="^(http[s]?:\/\/)?([-\w\d]+)(\.[-\w\d]+)*(\.([a-zA-Z]{2,5}|[\d]{1,3})){1,2}(\/([-~%\.\(\)\w\d]*\/*)*(#[-\w\d]+)?)?$" size="30" spellcheck="false" title="Your website address" type="url" value="" > </p> <p> <label for="text">Comment <abbr title="Required">*</abbr></label> <textarea aria-required="true" cols="40" id="text" name="text" required="required" rows="10" spellcheck="true" title="Your comment" ></textarea> </p> </fieldset> <fieldset> <input name="preview" type="submit" value="Preview"> <input name="save" type="submit" value="Submit Comment"> </fieldset> </form>
This example is a simple comment form where some fields are required, some fields are verified, and some fields satisfy both conditions. Fields with required
attributes also have aria-required
attributes to provide fallback semantics for assistive technologies that do not support new input types.
ARIA specification also defines the aria-invalid
attribute, which we will use to indicate if the field is invalid (there is no equivalent attribute in HTML5). The aria-invalid
property obviously provides accessibility information, but it can also be used as a CSS hook to apply red borders:
input[aria-invalid="true"], textarea[aria-invalid="true"] { border: 1px solid #f00; box-shadow: 0 0 4px 0 #f00; }
We can just use box-shadow
without worrying about borders. To be honest, this will look better, but in this way, there will be no indication in browsers that do not support box-shadow
(such as IE8).
Add JavaScript
Now that we have static code, we can add scripts. First, we need a basic addEvent()
function:
function addEvent(node, type, callback) { if (node.addEventListener) { node.addEventListener(type, function(e) { callback(e, e.target); }, false); } else if (node.attachEvent) { node.attachEvent('on' + type, function(e) { callback(e, e.srcElement); }); } }
Next, we need a function to determine whether a given field should be validated, which simply tests that it is neither disabled nor read-only, and that it has a pattern
or required
attribute:
function shouldBeValidated(field) { return ( !(field.getAttribute("readonly") || field.readonly) && !(field.getAttribute("disabled") || field.disabled) && (field.getAttribute("pattern") || field.getAttribute("required")) ); }The first two conditions may seem verbose, but they are necessary because the
and disabled
properties of an element do not necessarily reflect their attribute state. For example, in Opera, fields with hardcoded attribute readonly
still return readonly="readonly"
for their readonly
attribute (the point attribute only matches the state set through the script). undefined
function instantValidation(field) { if (shouldBeValidated(field)) { var invalid = (field.getAttribute("required") && !field.value) || (field.getAttribute("pattern") && field.value && !new RegExp(field.getAttribute("pattern")).test(field.value)); if (!invalid && field.getAttribute("aria-invalid")) { field.removeAttribute("aria-invalid"); } else if (invalid && !field.getAttribute("aria-invalid")) { field.setAttribute("aria-invalid", "true"); } } }Therefore, if the field is required but has no value, or it has pattern and value, but the value does not match the pattern, the field is invalid.
Since the pattern already defines the string form of the regular expression, we just need to pass the string to the
constructor, and it creates a regular expression object that we can test for that value. However, we have to pretest the value to make sure it is not empty so that the regex itself does not have to consider the empty string. RegExp
Once we have determined whether the field is invalid, we can control its aria-invalid
property to indicate the status - add it to an invalid field that does not yet have the property, or from a valid field that has the property deleted in. Very simple! Finally, in order for this to work, we need to bind the verification function to the onchange
event. It should be simple like this:
<form action="#" method="post"> <fieldset> <legend><strong>Add your comment</strong></legend> <p> <label for="author">Name <abbr title="Required">*</abbr></label> <input aria-required="true" id="author" name="author" pattern="^([- \w\d\u00c0-\u024f]+)$" required="required" size="20" spellcheck="false" title="Your name (no special characters, diacritics are okay)" type="text" value="" > </p> <p> <label for="email">Email <abbr title="Required">*</abbr></label> <input aria-required="true" id="email" name="email" pattern="^(([-\w\d]+)(\.[-\w\d]+)*@([-\w\d]+)(\.[-\w\d]+)*(\.([a-zA-Z]{2,5}|[\d]{1,3})){1,2})$" required="required" size="30" spellcheck="false" title="Your email address" type="email" value="" > </p> <p> <label for="website">Website</label> <input id="website" name="website" pattern="^(http[s]?:\/\/)?([-\w\d]+)(\.[-\w\d]+)*(\.([a-zA-Z]{2,5}|[\d]{1,3})){1,2}(\/([-~%\.\(\)\w\d]*\/*)*(#[-\w\d]+)?)?$" size="30" spellcheck="false" title="Your website address" type="url" value="" > </p> <p> <label for="text">Comment <abbr title="Required">*</abbr></label> <textarea aria-required="true" cols="40" id="text" name="text" required="required" rows="10" spellcheck="true" title="Your comment" ></textarea> </p> </fieldset> <fieldset> <input name="preview" type="submit" value="Preview"> <input name="save" type="submit" value="Submit Comment"> </fieldset> </form>event must bubble up (using a technology commonly known as event delegate), but in Internet Explorer 8 and earlier, the
event onchange
does not occur Bubbleonchange
. We can choose to ignore these browsers, but I think it would be a shame, especially if the problem is so easy to solve. It just means that the code is a little more complex - we have to get a collection of input and text area elements, iterate through them and bind the event to each field separately:
onchange
input[aria-invalid="true"], textarea[aria-invalid="true"] { border: 1px solid #f00; box-shadow: 0 0 4px 0 #f00; }Conclusion and Prospect
It's that - a simple and non-invasive real-time form verification enhancement that provides accessible and intuitive tips to help users complete forms.
After this script is implemented, we can actually complete a complete compatibility filler program in just a few steps. Such a script is beyond the scope of this article, but if you want to develop it further, all the basic modules are here-test whether the fields should be validated, the fields should be validated according to the schema and/or
, and the binding trigger event.
required
I must admit, I'm not sure if it's really worth it! If you already have this enhancement (which works in IE7 and all modern browsers), and consider that you have no choice but to implement server-side verification, and consider that you have support for
's browsers have used them for pre-submission verification - with all this in mind, is it really necessary to add another compatibility filler?
pattern
(The FAQ section about real-time verification can be added here, the content is the same as the FAQs section in the original document) required
The above is the detailed content of Instant Form Validation Using JavaScript. For more information, please follow other related articles on the PHP Chinese website!
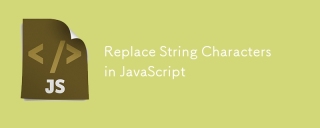
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
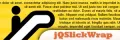
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
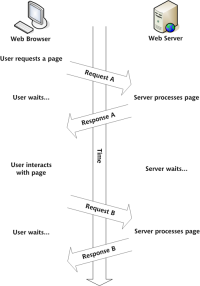
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
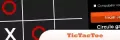
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
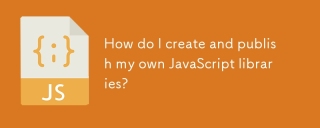
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
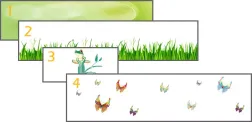
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
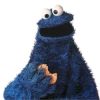
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session
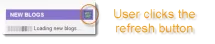
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
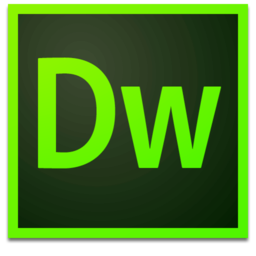
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
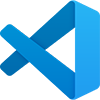
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
