This tutorial builds upon the previous introduction to the PHP Intl extension, focusing on localizing complex data like numbers, dates, and currencies. Let's dive in!
Key Concepts:
- The PHP Intl extension leverages the ICU library for robust locale-aware data handling, essential for multilingual applications.
-
NumberFormatter
handles number localization, addressing variations in decimal separators and formatting styles across different locales. - Currency formatting is easily achieved with
NumberFormatter
, specifying the currency code and using theformatCurrency
method. - The extension provides a comprehensive calendar API (
IntlCalendar
) for date manipulation and comparison, offering functionalities similar to popular date/time libraries.
Decimal Localization:
Inconsistencies in decimal separators across regions are a common challenge. The NumberFormatter
class elegantly solves this:
$numberFormatter = new NumberFormatter( 'de_DE', NumberFormatter::DECIMAL ); var_dump( $numberFormatter->format(123456789) ); // Output: string(11) "123.456.789" $numberFormatter = new NumberFormatter( 'en_US', NumberFormatter::DECIMAL ); var_dump( $numberFormatter->format(123456789) ); // Output: string(11) "123,456,789" $numberFormatter = new NumberFormatter( 'ar', NumberFormatter::DECIMAL ); var_dump( $numberFormatter->format(123456789) ); // Output: string(22) "١٢٣٬٤٥٦٬٧٨٩" $numberFormatter = new NumberFormatter( 'bn', NumberFormatter::DECIMAL ); var_dump( $numberFormatter->format(123456789) ); // Output: string(30) "১২,৩৪,৫৬,৭৮৯"
The locale code (e.g., 'de_DE', 'en_US') dictates the formatting style. Various formatting styles (decimal, currency, duration, etc.) are available.
Formatting Styles and Attributes:
We can customize number formatting using attributes:
$nf = new NumberFormatter( 'en_US', NumberFormatter::DECIMAL ); $nf->setAttribute(NumberFormatter::FRACTION_DIGITS, 2); var_dump( $nf->format(1234.56789) ); // Output: string(8) "1,234.57" var_dump( $nf->format(1234) ); // Output: string(8) "1,234.00"
Rounding behavior can be controlled:
$nf = new NumberFormatter( 'en_US', NumberFormatter::DECIMAL ); $nf->setAttribute(NumberFormatter::MAX_FRACTION_DIGITS, 2); $nf->setAttribute(NumberFormatter::ROUNDING_MODE, NumberFormatter::ROUND_CEILING); var_dump($nf->format(1234.5678) ); // Output: string(8) "1,234.57" $nf->setAttribute(NumberFormatter::ROUNDING_MODE, NumberFormatter::ROUND_DOWN); var_dump($nf->format(1234.5678) ); // Output: string(8) "1,234.56"
SPELLOUT
and DURATION
styles, introduced previously, also apply here. Parsing formatted strings back into numbers is supported via the parse
method.
Currency Localization:
Formatting numbers as currencies is straightforward:
$nf = new NumberFormatter( 'en_US', NumberFormatter::CURRENCY ); var_dump( $nf->formatCurrency(1234.56789, "USD" ) ); // Output: string(9) ",234.57"
The getSymbol
method simplifies currency symbol retrieval:
$nf = new NumberFormatter( 'en_US', NumberFormatter::CURRENCY ); var_dump( $nf->formatCurrency(1234.56789, $nf->getSymbol(NumberFormatter::INTL_CURRENCY_SYMBOL)) ); // Output: string(9) ",234.57" $nf = new NumberFormatter( 'fr_FR', NumberFormatter::CURRENCY ); var_dump( $nf->formatCurrency(1234.56789, $nf->getSymbol(NumberFormatter::INTL_CURRENCY_SYMBOL)) ); // Output: string(14) "1 234,57 €"
Time Zones and Calendars:
IntlTimeZone
manages time zones, mirroring functionalities of DateTimeZone
. IntlCalendar
provides a rich API for calendar operations:
$calendar = IntlCalendar::createInstance(); var_dump($calendar->getTimeZone()->getId()); // Output: Time zone ID (e.g., "UTC") $calendar = IntlCalendar::fromDateTime(new DateTime()); // Create from DateTime object // Comparisons $calendar1 = IntlCalendar::fromDateTime( DateTime::createFromFormat('j-M-Y', '11-Apr-2016') ); $calendar2 = IntlCalendar::createInstance(); $diff = $calendar1->fieldDifference($calendar2->getTime(), IntlCalendar::FIELD_MILLISECOND); // ... (comparison and date navigation examples as before)
Date navigation is intuitive:
$calendar = IntlCalendar::createInstance(); $calendar->add(IntlCalendar::FIELD_MONTH, 1); // Add a month $calendar->add(IntlCalendar::FIELD_DAY_OF_WEEK, 1); // Add a day of the week // ...
Conclusion:
The PHP Intl extension, powered by ICU, offers a powerful and comprehensive solution for internationalizing your PHP applications. This two-part series has covered message localization and now complex data localization. Future articles will explore additional functionalities within the Intl extension.
The above is the detailed content of Localizing Dates, Currency, and Numbers with Php-Intl. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
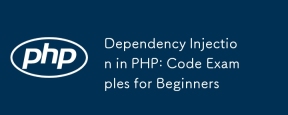
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
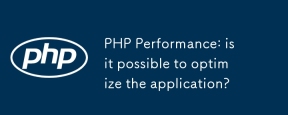
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
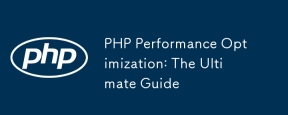
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
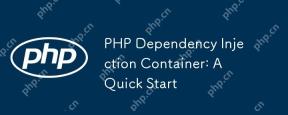
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
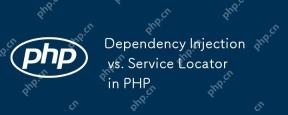
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
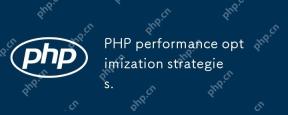
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
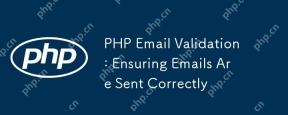
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
