Coding Challenges in Technical Interview: Full Preparation and Effective Response
The coding process in technical interviews has always been controversial, especially in the front-end field, where some questions are weakly related to daily work. However, many companies still stick to this kind of screening method, and they value candidates' logical thinking, problem-solving ability and creativity rather than simply technical proficiency. This article will explore how to effectively deal with five common JavaScript/front-end junior engineer interview coding challenges.
Interview preparation strategy:
- Full preparation: Prioritize to learn unfamiliar knowledge points, and conduct a lot of handwritten code exercises to simulate the whiteboard interview environment. Platforms such as GeeksforGeeks and Pramp are good practice resources.
- Clearly understanding the problem: Be sure to fully understand the problem before coding, considering the boundary situation and input/output type, which helps to form the right solution.
- Think loudly during the interview: During the interview, clearly express your ideas, demonstrate your problem solving methods and communication skills, and let the interviewer follow up on your ideas and provide necessary help.
- Common challenges of practicing: Master common coding challenges such as palindrome, FizzBuzz, and riddles, and thoroughly understand their logic and solutions.
- Code Review and Testing: Always test your code with various cases, ensure its accuracy, and discuss potential alternatives or optimizations to demonstrate your in-depth understanding and flexibility of coding.
Common JavaScript coding challenges:
The following are five common challenges and provide problem-solving ideas and sample code:
1. Palindrome (Palindrome)
Judge whether a string is palindrome (both and back are read the same).
Problem solution: Convert the string to lowercase, then reverse it, and then compare it with the original string.
Sample code:
const palindrome = str => str.toLowerCase() === str.toLowerCase().split('').reverse().join('');
2. FizzBuzz
Print numbers from 1 to n, multiples of 3 print "fizz", multiples of 5 print "buzz", multiples of 3 and multiples of 5 print "fizzbuzz".
Problem solution: Use the modular operator (%) to judge the multiple relationship and print the corresponding results according to the conditions.
Sample code:
const fizzBuzz = n => { for (let i = 1; i <= n; i++) { if (i % 15 === 0) console.log('fizzbuzz'); else if (i % 3 === 0) console.log('fizz'); else if (i % 5 === 0) console.log('buzz'); else console.log(i); } };
3. Anagram (Anagram)
Judge whether the two strings are riddles (the letters are the same, the number is the same, and the order is different).
Problem solution: Create a character count object, count the number of occurrences of each character in the two strings, and then compare whether the two objects are the same.
Sample code:
const anagram = (str1, str2) => { const charCount = str => [...str.toLowerCase()].reduce((acc, char) => { acc[char] = (acc[char] || 0) + 1; return acc; }, {}); return Object.keys(charCount(str1)).length === Object.keys(charCount(str2)).length && Object.keys(charCount(str1)).every(key => charCount(str1)[key] === charCount(str2)[key]); };
4. Find the Vowels
Statistics the number of vowel letters (a, e, i, o, u) in a string.
Problem solution: Use regular expressions to match vowel letters and return the length of the matching result.
Sample code:
const palindrome = str => str.toLowerCase() === str.toLowerCase().split('').reverse().join('');
5. Fibonacci Sequence (Fibonacci)
Returns the nth number of the Fibonacci sequence.
Problem solution: Iterative or recursive methods can be used. Iterative methods are more efficient.
Sample code (iteration):
const fizzBuzz = n => { for (let i = 1; i <= n; i++) { if (i % 15 === 0) console.log('fizzbuzz'); else if (i % 3 === 0) console.log('fizz'); else if (i % 5 === 0) console.log('buzz'); else console.log(i); } };
Summary:
Proficiency in these common coding challenges and understanding the logic and data structures behind them will greatly improve your success rate in technical interviews. Remember, clear communication and problem-solving ideas are equally important.
FAQs:
(The FAQ part in the original text is omitted here because this part of the content is highly overlapped with the generated text, and to avoid redundancy, it will not be repeated.)
The above is the detailed content of How to Beat 5 Common JavaScript Interview Challenges. For more information, please follow other related articles on the PHP Chinese website!
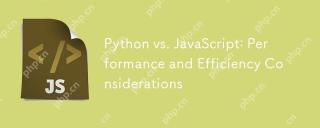
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
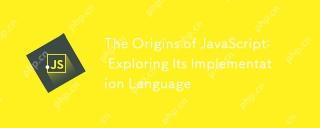
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
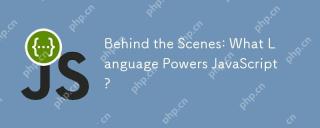
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
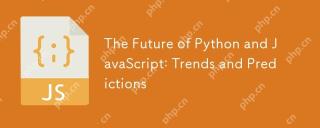
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
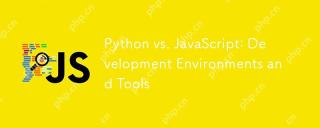
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
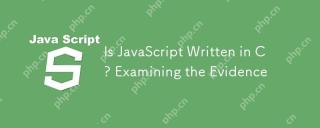
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
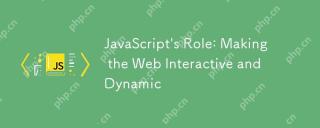
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
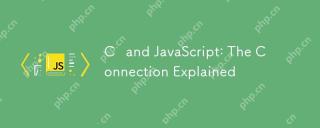
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
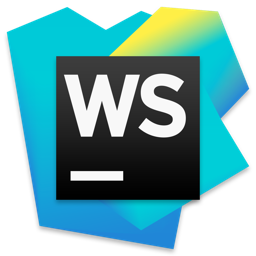
WebStorm Mac version
Useful JavaScript development tools
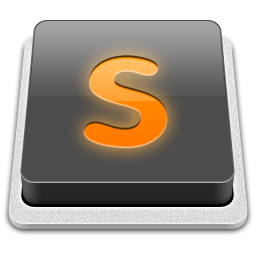
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
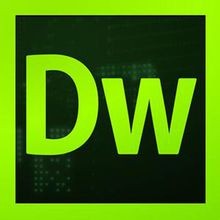
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
