This tutorial provides a practical guide to using CouchDB, a NoSQL database, via its HTTP API with PHP. We'll skip the theoretical aspects and dive straight into performing database operations. We assume you have CouchDB and Futon (its web admin console) already set up.
Note: We use localhost
for simplicity. If using a VM, adjust accordingly (e.g., using a custom vhost and forwarded ports).
Key Concepts:
- CouchDB Basics: A NoSQL database using JSON for storage, JavaScript for queries, and an HTTP API.
- Document Structure: Data is stored in individual JSON documents; no fixed schema or tables.
- HTTP API: Use HTTP requests (GET, POST, PUT, DELETE) to interact with the database.
- PHP Integration: We'll demonstrate using Guzzle and Doctrine's CouchDB Client.
- Advanced Features (briefly mentioned): MapReduce, conflict resolution, change feeds.
Creating a Database:
Use Futon (https://www.php.cn/link/3bbbc60ff463969b78a091ff51ac6566) to create a database. Give it a name (e.g., test_couch
).
After creation, you'll see an interface to add documents. Remember, in CouchDB, documents are the fundamental unit; there are no tables. You can store different data structures within the same database. For organization, include a type
field to categorize documents (e.g., "type": "users"
).
Example Document Structures:
-
User:
{"id": 123, "fname": "doppo", "lname": "kunikida", "pw": "secret", "hobbies": ["reading", "sleeping"], "type": "users"}
-
Blog Post:
{"title": "The big brown fox", "author": "fox", "text": "...", "publish_date": "2016-07-07", "type": "blog_posts"}
HTTP API Interaction:
You can use curl
or tools like Postman to interact directly with the CouchDB HTTP API.
-
Create Database (curl):
curl -X PUT http://localhost:5984/<database_name></database_name>
(returns{"ok":true}
) -
Create Document (Postman): Send a POST request to
http://localhost:5984/test_couch
, withContent-Type: application/json
and your JSON document in the body.
Bulk Insert:
Use a POST request to http://localhost:5984/test_couch/_bulk_docs
with an array of documents in the JSON body.
Retrieving Documents:
-
All Documents:
http://localhost:5984/test_couch/_all_docs?include_docs=true
-
Specific Document:
http://localhost:5984/test_couch/<document_id>?include_docs=true</document_id>
-
Specific Revision: Add
&rev=<revision_id></revision_id>
to the URL above.
CouchDB tracks revisions; each update creates a new revision. Futon allows browsing through these revisions.
Views (MapReduce):
Views provide querying capabilities. Create views in Futon using map and reduce functions (JavaScript). These functions are saved within design documents.
- Example Map Function (filter by trainer):
function(doc) { emit(doc.trainer, doc.name); }
Access views via URLs like: http://localhost:5984/test_couch/_design/<design_doc>/_view/<view_name>?key="Ash"</view_name></design_doc>
Updating Documents:
Send a PUT request to the document URL, including the _rev
field with the latest revision ID. You must provide the entire updated document.
Deleting Documents:
Send a DELETE request to the document URL, including the _rev
field with the latest revision ID.
http://localhost:5984/test_couch/<document_id>?rev=<revision_id></revision_id></document_id>
PHP Integration (Guzzle):
Guzzle simplifies HTTP requests in PHP. The following examples demonstrate basic operations: (Remember to install Guzzle: composer require guzzlehttp/guzzle
)
<?php require 'vendor/autoload.php'; use GuzzleHttp\Client; $client = new Client(['base_uri' => 'http://localhost:5984']); // ... (GET, POST, PUT, DELETE examples using Guzzle similar to the curl and Postman examples above) ... ?>
PHP Integration (Doctrine CouchDB Client):
Doctrine's CouchDB client provides a higher-level abstraction. (Install with: composer require doctrine/couchdb
)
<?php require 'vendor/autoload.php'; $client = \Doctrine\CouchDB\CouchDBClient::create(['dbname' => 'test_couch']); // ... (Examples for creating, retrieving, updating, and deleting documents using the Doctrine client) ... ?>
Conclusion:
This tutorial offers a practical introduction to CouchDB using its HTTP API and PHP. Explore the official documentation and the Definitive Guide for more advanced features. Future posts will delve deeper into building applications with CouchDB.
The above is the detailed content of A Pokemon Crash Course on CouchDB. For more information, please follow other related articles on the PHP Chinese website!
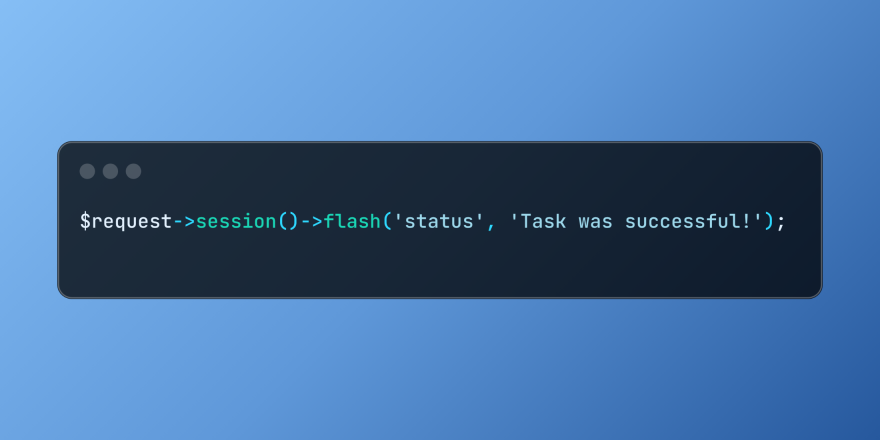
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
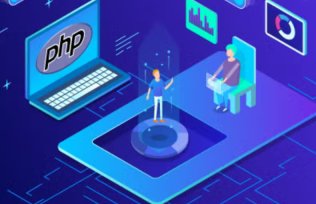
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
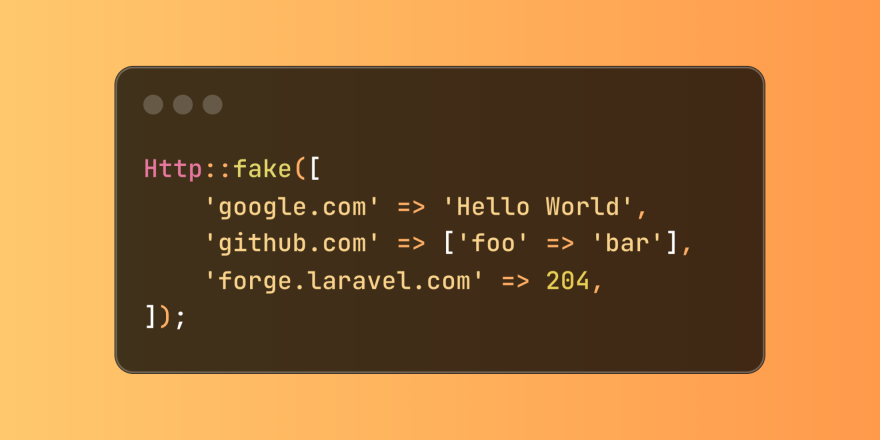
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
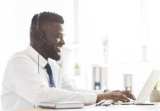
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
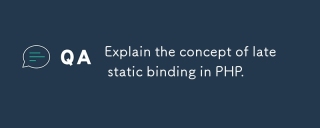
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
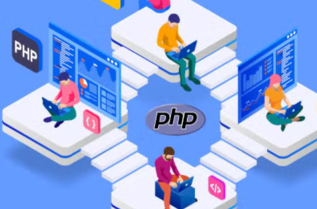
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
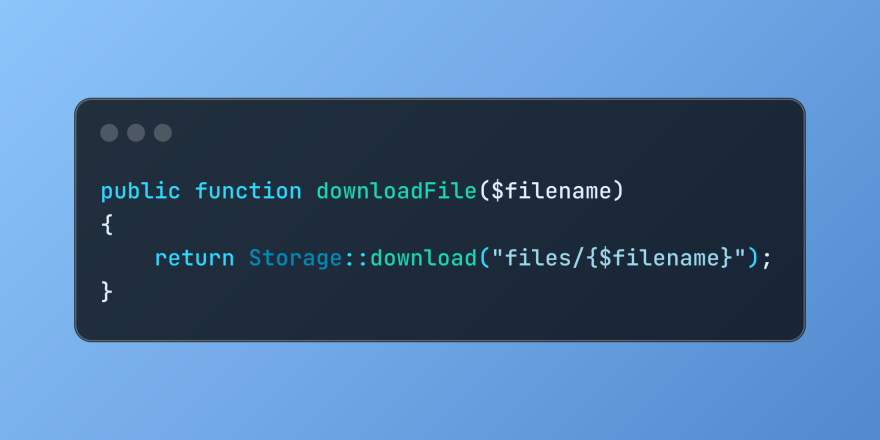
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
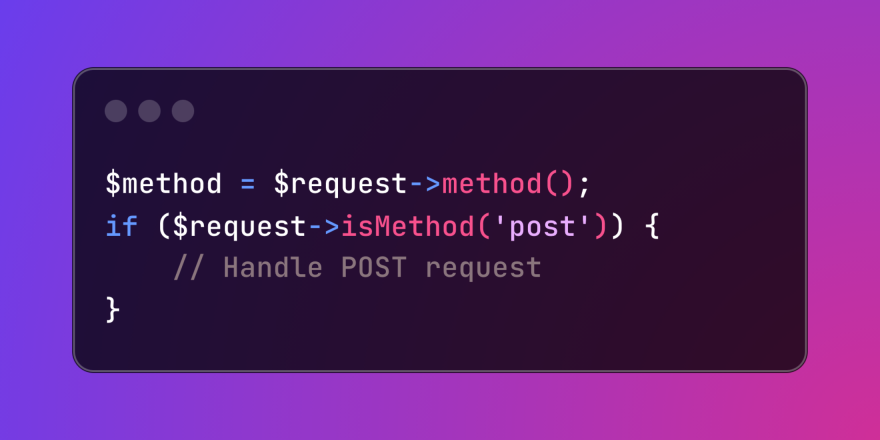
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
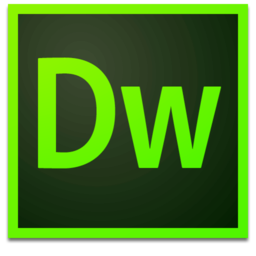
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
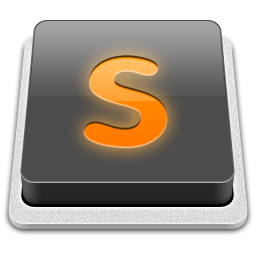
SublimeText3 Mac version
God-level code editing software (SublimeText3)
