When building complex applications, architecture design is as important as code quality. Even if the code is well written, but lacks a good organizational structure, maintaining and scaling becomes extremely difficult as complexity increases. Therefore, the best practice is to plan the architecture before the project starts, guide the project goals and make informed choices.
Unlike frameworks such as Ruby on Rails, Node.js does not have a de facto standard framework to enforce specific architectures and code organization. This makes architecture choice relatively free when building a complete Node.js web application, but also adds challenges.
This tutorial will use the MVC architecture to build a basic note-taking application, demonstrating how to use Node.js' Hapi.js framework, SQLite database (operating through Sequelize.js), and the Pug template engine to achieve efficient development.
Core points:
- Efficiently organize Node.js applications using MVC (Model-View-Controller) architecture to improve maintainability and scalability.
- Initialize the project with npm and create
package.json
files to manage dependencies. - Build a server using the Hapi.js framework and manage data using SQLite database and Sequelize.js.
- Define Node.js routes, clarify the functionality of the application, and ensure that each route is associated with the correct HTTP method and handler.
- Define the model using Sequelize.js to ensure data integrity and provide an abstraction layer.
- Implement the controller to process business logic, connect models and views, obtain data and determine the output format.
- Create views using the Pug template engine to make HTTP responses more readable and structured.
- Use the Inert plugin of Hapi.js to provide static file services and efficiently manage CSS and JavaScript files.
What is MVC?
Model-View-Controller (MVC) is probably one of the most popular application architectures. The MVC pattern was originally designed in PARC for the Smalltalk language to solve the organizational problems of graphical user interface applications. Although originally used in desktop applications, the concept has been successfully applied to web development.
Simply put, the MVC architecture includes:
- Model: Parts of processing database or any data-related functions.
- View (View): Everything the user sees - the page sent to the client.
- Controller: The logic of the website, and the bridge between the model and the view. The controller calls the model to get the data, then passes the data to the view, and finally sends it to the user.
This application will allow the creation, viewing, editing and deletion of plain text notes. While the features are simple, the solid architecture will make it easy to add new features in the future.
This tutorial assumes that you have installed the latest version of Node.js on your machine. If not, please refer to the relevant tutorial for installation.
The final application code has been uploaded to the GitHub repository, and you can view the complete project structure.
Land the foundation
The first step in building any Node.js application is to create a package.json
file with all dependencies and scripts. This file can be automatically generated using npm's init
command:
mkdir notes-board cd notes-board npm init -yOnce
is finished, you will have an available package.json
file.
Note: If you are not familiar with these commands, please check out our npm Getting Started Guide.
Next, install the Hapi.js framework:
npm install @hapi/hapi@18.4.0
This command will download Hapi.js and add it to the dependencies in the package.json
file.
Note: We specified the v18.4.0 version of Hapi.js because it is compatible with Node 8, 10 and 12 versions. If you are using Node 12, you can choose to install the latest version (Hapi v19.1.0).
Now, create the entry file - start the web server with everything. Create a server.js
file in the application directory and add the following code:
"use strict"; const Hapi = require("@hapi/hapi"); const Settings = require("./settings"); const init = async () => { const server = new Hapi.Server({ port: Settings.port }); server.route({ method: "GET", path: "/", handler: (request, h) => { return "Hello, world!"; } }); await server.start(); console.log(`Server running at: ${server.info.uri}`); }; process.on("unhandledRejection", err => { console.log(err); process.exit(1); }); init();
This is the basis of the application. First, we use strict mode; then, we introduce dependencies and instantiate a new server object, setting the connection port to 3000 (the port can be any number between 1023 and 65535); our first route will be Test, so the "Hello, world!" message is enough; finally, we start the server using the server.start()
method.
Storage settings
Best practice is to store configuration variables in a dedicated file. This file exports a JSON object containing data, where each key is assigned an independent environment variable, but don't forget the alternate values.
In this file, we can also have different settings depending on the environment (such as development or production). For example, we can use memory instances of SQLite for development purposes, but use real SQLite database files in production environments.
Selecting settings based on the current environment is very simple. Since we also have an env variable in the file that will contain development or production, we can do the following to get the database settings:
const dbSettings = Settings[Settings.env].db;
Therefore, when the env variable is development, dbSettings will contain the settings of the in-memory database; when the env variable is production, the path to the database file will be included.
In addition, we can add support for .env
files where local environment variables can be stored for development purposes. This can be done through a Node.js package like dotenv, which will read the .env
file in the root of the project and automatically add the found values to the environment.
Note: If you decide to use the .env
file as well, make sure to install the package using npm install dotenv
and add it to .gitignore
to avoid posting any sensitive information.
Our settings.js
File is as follows:
// This will load our .env file and add the values to process.env, // IMPORTANT: Omit this line if you don't want to use this functionality require("dotenv").config({ silent: true }); module.exports = { port: process.env.PORT || 3000, env: process.env.NODE_ENV || "development", // Environment-dependent settings development: { db: { dialect: "sqlite", storage: ":memory:" } }, production: { db: { dialect: "sqlite", storage: "db/database.sqlite" } } };
Now, we can start the application by executing the following command and navigating to http://localhost:3000
in our web browser:
mkdir notes-board cd notes-board npm init -y
Note: This project was tested on Node v12.15.0. If you encounter any errors, make sure you have an updated version installed.
(The following steps, due to space limitations, the following content will be briefly summarized. Please refer to the original text for specific implementation details)
Define routes: Define routes for applications in lib/routes.js
files, including GET, POST, PUT, and DELETE methods to handle the creation, reading, updating and deletion of notes.
Build a model: Create a Note
using Sequelize.js and SQLite to define the data structure of the notes (date, title, content, etc.). Define the model in lib/models/note.js
.
Build the controller: Create the controller to handle routing requests. lib/controllers/home.js
Processing home page, lib/controllers/note.js
Processing CRUD operations related to notes.
Build views: Create views using the Pug template engine. lib/views/home.pug
Show the list of notes, lib/views/note.pug
Show individual notes details. lib/views/components/note.pug
Define the note component.
Service static files: Use the Inert plugin of Hapi.js to serve static files (JavaScript and CSS). Register the Inert plugin in server.js
and add static file routes in routes.js
.
Client JavaScript: Write client JavaScript code to handle display/hide modal windows, and submit forms using Ajax.
Summary
This tutorial builds a basic note-taking application based on Hapi.js and MVC architecture. While there are still many details to be refined (such as input validation, error handling, etc.), this provides a foundation for learning and building Node.js applications. You can add user authentication, more complex UI and other functions on this basis.
The above is the detailed content of How to Build and Structure a Node.js MVC Application. For more information, please follow other related articles on the PHP Chinese website!
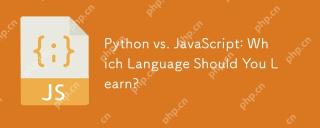
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
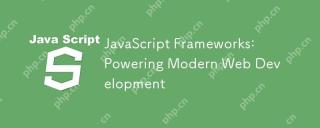
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
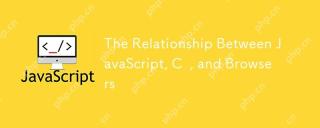
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
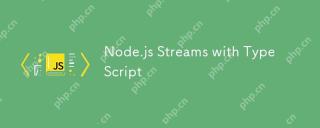
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
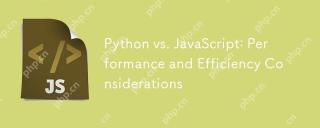
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
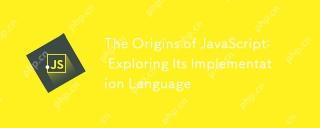
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
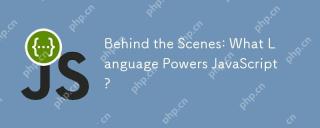
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
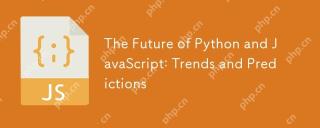
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
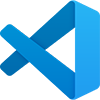
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
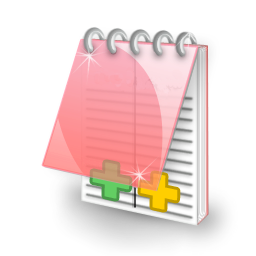
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
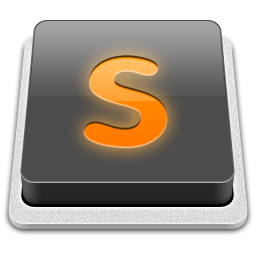
SublimeText3 Mac version
God-level code editing software (SublimeText3)
