This tutorial explores web workers and their application in resolving performance bottlenecks. We'll cover both browser and server-side implementations, highlighting their capabilities and limitations.
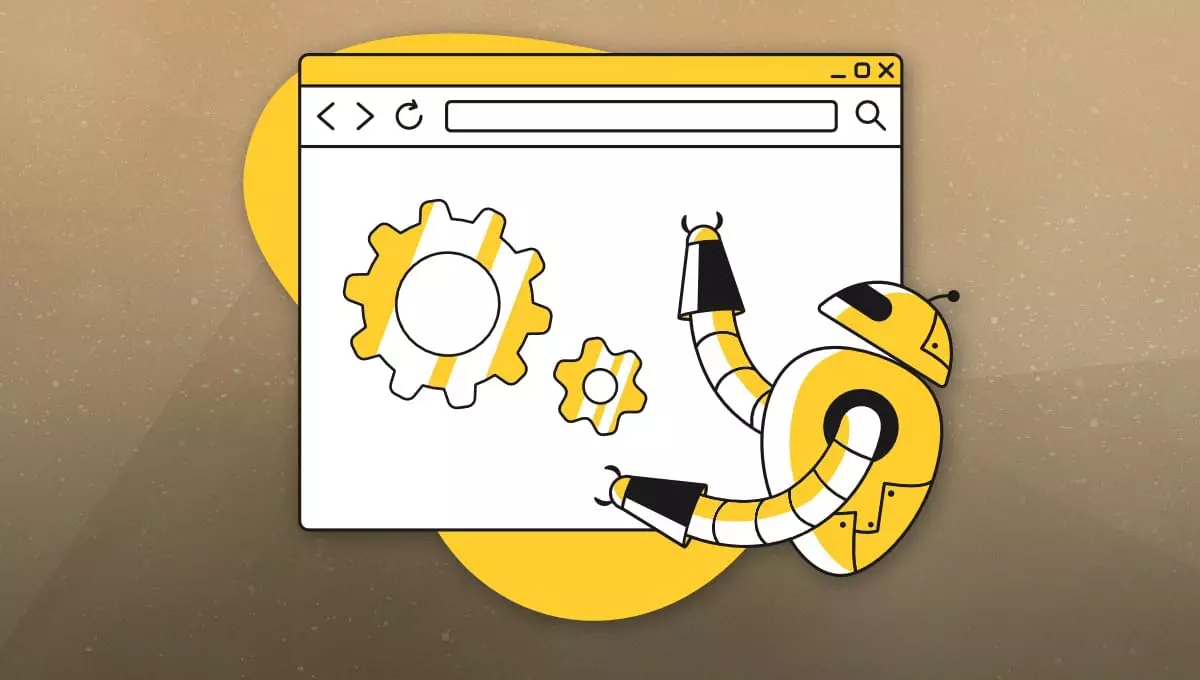
Key Concepts:
-
JavaScript's Single-Threaded Nature: JavaScript, in browsers and Node.js, traditionally runs on a single thread. This limits parallel processing, potentially causing performance issues with long-running tasks.
-
The Event Loop: JavaScript's asynchronous nature uses an event loop to handle I/O operations without blocking the main thread. However, CPU-bound tasks still block execution.
-
Web Workers: The Solution: Web workers provide a mechanism for running scripts concurrently in background threads, preventing UI freezes in browsers and improving server-side responsiveness.
Browser-Side Web Workers:
The tutorial demonstrates a browser-based application featuring a clock and a dice-rolling simulator. The example showcases how web workers enable the clock to continue running even while a computationally intensive dice-rolling simulation is in progress.
-
Dedicated vs. Shared Workers: The differences between dedicated (one-to-one communication) and shared workers (multiple threads accessing a single worker) are explained, noting the limited browser support for shared workers.
-
Limitations: Web workers operate in isolation, lacking direct access to the DOM and certain global objects. This is a crucial security measure to prevent conflicts. They are most effective for CPU-bound tasks, not I/O-bound ones.
-
Implementation: The tutorial details how to create and use web workers, including message passing, error handling (
onmessageerror
, onerror
), and the use of importScripts()
for importing external scripts. The complexities of using ES modules with workers are also addressed.
-
Service Workers: A brief overview of service workers, their distinct functionality, and their differences from web workers is provided.
Server-Side Web Workers (Node.js):
The tutorial expands on the use of web workers in Node.js (version 10 and above), showcasing a similar dice-rolling example.
-
Implementation: The example demonstrates how to create and manage workers in Node.js, using
workerData
for initial data transfer and parentPort.postMessage()
for communication. Event handlers (message
, exit
, error
) are used for managing worker lifecycle and errors.
-
Inline Workers: The possibility of embedding both main and worker code within a single file is discussed.
-
Limitations: Similar to browser workers, server-side workers have limitations regarding data sharing. Techniques for sharing data using
SharedArrayBuffer
are explored, along with their caveats.
-
Alternatives: The tutorial covers alternatives to web workers in Node.js, including:
-
Child Processes: For launching external processes.
-
Clustering: For creating multiple instances of a Node.js application to handle increased load.
-
Process Managers (e.g., PM2): For simplifying the management of multiple Node.js instances.
-
Container Managers (e.g., Docker, Kubernetes): For deploying and managing applications across multiple machines.
Conclusion:
Web workers offer a powerful approach to enhancing performance by enabling parallel processing. The tutorial provides a comprehensive guide to their usage, limitations, and alternatives, empowering developers to make informed decisions about their application of this technology. The FAQs section further clarifies key aspects of web workers and their relationship to other web technologies. The image illustrating the architecture of web workers remains in its original position.
The above is the detailed content of Develop Faster JS Apps: the Ultimate Guide to Web Workers. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn