<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript String Splitting: substring() vs. split()</title> </head> <body> <h1 id="JavaScript-String-Splitting-substring-vs-split">JavaScript String Splitting: `substring()` vs. `split()`</h1> <p>JavaScript offers two primary methods for dividing strings into substrings: `substring()` and `split()`. Each serves a distinct purpose.</p> <h2 id="substring">`substring()`</h2> <p>The `substring()` method extracts a portion of a string based on starting and ending indices. It's ideal for retrieving a single substring from a known position within the string.</p> <p><strong>Syntax:</strong> <code>string.substring(startIndex, endIndex)</code></p> <ul> <li><code>startIndex</code>: The index of the first character to include (0-based).</li> <li><code>endIndex</code> (optional): The index *after* the last character to include. If omitted, the substring extends to the end of the string.</li> </ul> <p><strong>Example:</strong></p> <pre class="brush:php;toolbar:false"><code> const str = "Hello, world!"; const sub = str.substring(7, 12); // Extracts "world" console.log(sub); // Output: "world"
Often, you'll use `indexOf()` to dynamically determine the start or end indices.
`split()`
The `split()` method divides a string into an array of substrings based on a specified separator. This is perfect for handling strings containing lists of items delimited by a character (e.g., comma-separated values).
Syntax: string.split(separator, limit)
-
separator
: The character or string used to divide the string. -
limit
(optional): The maximum number of substrings to return.
Example:
<code> const csv = "apple,banana,cherry"; const fruits = csv.split(","); // Splits at each comma console.log(fruits); // Output: ["apple", "banana", "cherry"] </code>
Key Differences
Feature | `substring()` | `split()` |
---|---|---|
Output | Substring (string) | Array of substrings |
Purpose | Extract a portion at specific indices | Split into multiple substrings based on a separator |
Index-based | Yes | No (separator-based) |
When to Use Each Method
- Use `substring()` when you need to extract a single, continuous piece of text from a known position within the string.
- Use `split()` when you need to break a string into multiple parts based on a delimiter, such as commas, spaces, or other characters.
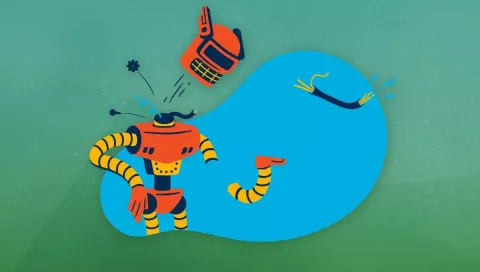
The above is the detailed content of How to Split a String into Substrings in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
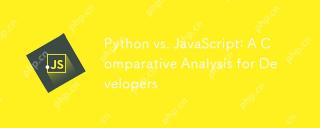
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
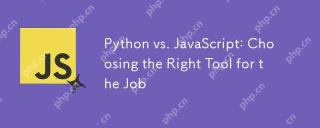
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
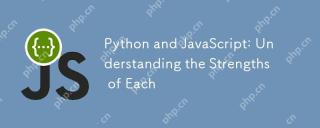
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
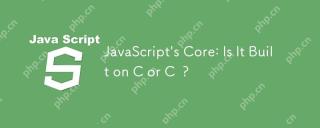
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
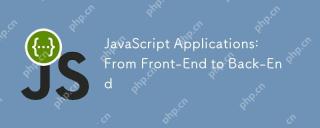
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
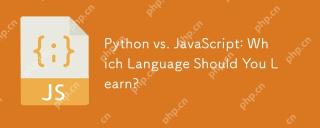
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
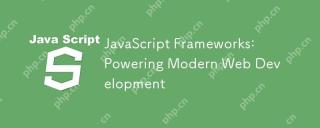
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
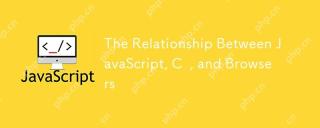
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
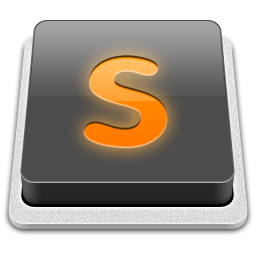
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
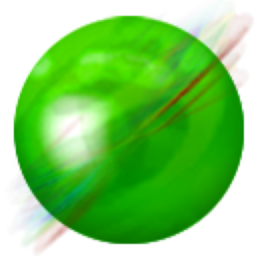
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
