JavaScript's equality comparisons can be tricky due to its loose typing. This article explores the nuances of double (==) and triple (===) equals operators, and the concept of truthy and falsy values.
Understanding these concepts leads to cleaner, more predictable code.
Key Takeaways:
- JavaScript's dynamic typing allows flexible value assignments but can cause unexpected comparison results. Loose equality (==) performs type coercion, often leading to surprising outcomes. Strict equality (===) directly compares values and types, resulting in more reliable comparisons.
- Every JavaScript value is either truthy or falsy. Falsy values are
false
,0
,-0
,0n
,""
,null
,undefined
, andNaN
. All other values are truthy, including'0'
,'false'
,[]
,{}
, and functions. - Loose equality comparisons with truthy/falsy values can be unpredictable. For example,
false == 0 == ''
is true, but[] == true
is false, and[] == false
is true! Strict equality avoids these ambiguities. - To avoid errors, avoid direct truthy/falsy comparisons. Use strict equality (
===
) and explicitly convert to Boolean values (Boolean()
or!!
) when necessary. This ensures predictable behavior.
JavaScript's Typing System:
JavaScript variables are loosely typed:
let x; x = 1; // x is a number x = '1'; // x is a string x = [1]; // x is an array
Loose equality (==) converts values to strings before comparison:
// all true 1 == '1'; 1 == [1]; '1' == [1];
Strict equality (===) considers type:
// all false 1 === '1'; 1 === [1]; '1' === [1];
JavaScript's primitive types are: undefined
, null
, boolean
, number
, bigint
, string
, and symbol
. Everything else is an object (including arrays).
Truthy vs. Falsy Values:
Each value has a Boolean equivalent:
-
Falsy:
false
,0
,-0
,0n
,""
,null
,undefined
,NaN
- Truthy: Everything else.
Example:
if (value) { // value is truthy } else { // value is falsy }
document.all
(deprecated) is also falsy.
Loose Equality (==) Comparisons:
Loose equality leads to unexpected results with truthy/falsy values:
Strict Equality (===) Comparisons:
Strict equality provides clearer results:
Note that NaN === NaN
is always false
.
Recommendations:
-
Avoid direct comparisons: Use
!x
instead ofx == false
. -
Use strict equality (
===
): Provides more predictable results. -
Convert to Boolean: Use
Boolean(x)
or!!x
for explicit Boolean conversion.
Conclusion:
Understanding truthy/falsy values and using strict equality improves code reliability. Avoid the pitfalls of loose comparisons to prevent debugging headaches.
FAQs (abbreviated for brevity):
- Truthy/Falsy Concept: Values treated as true/false in Boolean contexts.
- JavaScript's Handling: Type coercion converts values to Boolean.
- Examples: See above lists.
-
Checking Truthy/Falsy: Use in Boolean contexts or
Boolean()
function. -
==
vs===
: Loose vs. strict equality;===
avoids type coercion. -
Evaluating Expressions: Short-circuiting in logical AND (
&&
) and OR (||
). -
Non-Boolean Falsy Values:
0
,""
,null
,undefined
,NaN
,-0
. -
Converting to Boolean: Use
Boolean()
. - Falsy Values in Logical Operations: Affect operation results.
-
Special Cases:
0
vs'0'
, empty arrays/objects are truthy.
The above is the detailed content of Truthy and Falsy Values: When All is Not Equal in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
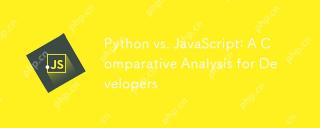
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
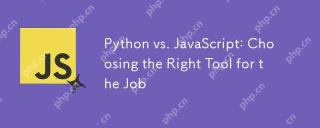
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
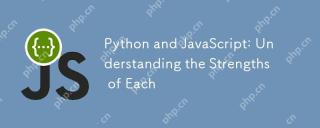
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
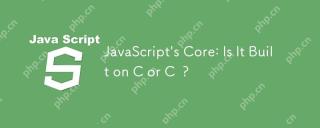
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
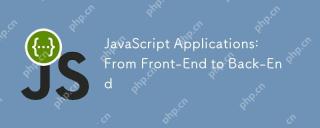
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
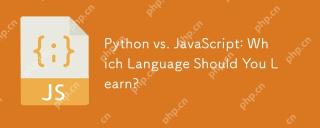
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
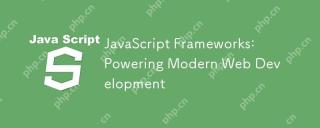
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
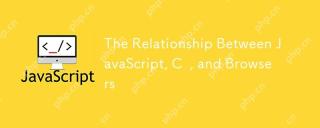
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
