This article will discuss several methods and application scenarios for removing the beginning and end spaces of strings in PHP.
Spaces in strings usually refer to spaces at the beginning or end. (The spaces between words are also spaces, but this article mainly focuses on the beginning and end spaces.)
In PHP string processing, the beginning and the end spaces often cause trouble. Removing spaces can effectively clean and standardize data.
The importance of removing spaces
Give an example the importance of removing spaces: Suppose that a user name is stored in the database, a user accidentally adds a space at the end of the user name. This can cause problems with search or sorting. Use the trim()
function to remove the beginning and end spaces, which ensures that all usernames in the database are consistent in format and are easier to handle.
When working with text-based data formats such as XML or JSON, it is also important to remove spaces. These formats have strict regulations on the use of spaces, and failure to comply with these regulations can lead to errors or unexpected behavior. Use the trim()
or preg_replace()
functions to remove unnecessary spaces to ensure the correct format of the data and improve processing efficiency.
Methods to remove spaces in PHP
The following are some ways to remove spaces in PHP:
trim()
The trim()
function removes spaces at the beginning and end of a string. For example:
$str = " Hello World! "; $str = trim($str); echo $str; // 输出: "Hello World!"
ltrim()
The ltrim()
function removes spaces at the beginning of a string. For example:
$str = " Hello World! "; $str = ltrim($str); echo $str; // 输出: "Hello World! "
rtrim()
The rtrim()
function removes spaces at the end of the string. For example:
$str = " Hello World! "; $str = rtrim($str); echo $str; // 输出: " Hello World!"
preg_replace()
The preg_replace()
function uses regular expressions to remove spaces in strings. For example:
$str = " Hello World! "; $str = preg_replace('/\s+/', '', $str); echo $str; // 输出: "HelloWorld!"
Especial care is required when using regular expressions, the results may not match expectations. In the above example, spaces between words are also removed.
If you need to remove spaces inside a string, you can use the str_replace()
function to replace all spaces with other characters or strings.
Summary
Removing spaces in PHP is essential to clean and standardize data, improve security, and ensure the correct format of text data. Whether you need to remove the beginning and end spaces or the spaces inside a string, PHP provides a variety of functions to complete these tasks. Using functions such as trim()
, ltrim()
, rtrim()
, and preg_replace()
can ensure that the data is formatted correctly and easier to process.
PHP space removal FAQ
What are spaces in PHP? Spaces in PHP refer to spaces, tabs, and line breaks in strings that are used for formatting but not displayed as visible characters.
Why do you need to remove spaces from PHP strings? Removing spaces ensures data consistency, prevents input errors, and ensures that the text does not contain unwanted head and tail spaces.
How to remove the beginning and end spaces of a string in PHP? You can use the trim()
function to remove the beginning and end spaces of a string.
Can the beginning and end spaces be removed separately? Yes, you can use the ltrim()
function to remove the beginning spaces and use the rtrim()
function to remove the end spaces.
Will removing spaces modify the original string, or will it return a new string? The space removal functions in PHP do not modify the original string; they return a new string with space removal.
The above is the detailed content of Quick Tip: How to Trim Whitespace with PHP. For more information, please follow other related articles on the PHP Chinese website!
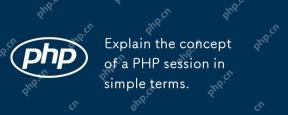
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
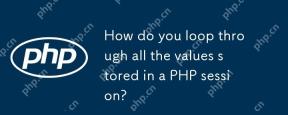
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
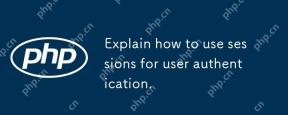
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
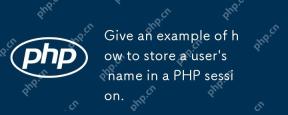
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
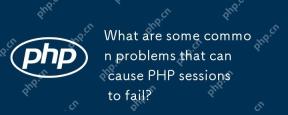
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
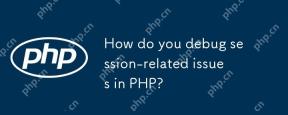
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
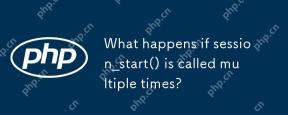
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
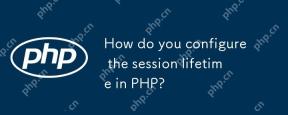
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
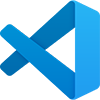
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
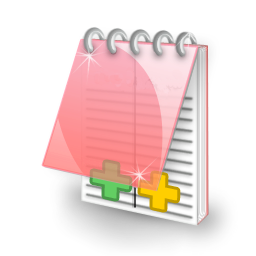
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
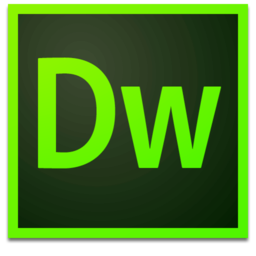
Dreamweaver Mac version
Visual web development tools
