This excerpt from PHP & MySQL: Novice to Ninja, 7th Edition introduces fundamental PHP concepts. We'll cover PHP's server-side nature, basic syntax, variables, operators, comments, and control structures.
PHP: Server-Side Scripting
Unlike client-side languages (HTML, CSS, JavaScript) executed by the browser, PHP runs on the server before sending the page to the browser. This allows for dynamic content generation, enhanced security, and reduced client load. PHP code, enclosed in <?php
and ?>
tags within .php
files, is processed by the server, and the resulting HTML is sent to the browser.
Example: Generating a Random Number
The following code generates a random number:
<?php echo rand(1, 10); ?>
The browser only sees the generated number, not the PHP code itself. This highlights key advantages: security (server-side generation prevents client-side manipulation), browser compatibility (server-side processing eliminates browser-specific issues), and access to server resources (databases, files, etc.).
Basic Syntax and Statements
PHP syntax resembles C-derived languages. Statements end with semicolons (;). The echo
statement outputs content (often HTML):
echo 'This is a <strong>test</strong>!';
Strings are enclosed in single (' ') or double (" ") quotes. Functions, identified by parentheses, perform specific tasks (e.g., rand()
generates a random number).
Variables, Operators, and Comments
Variables start with a dollar sign ($) (e.g., $testVariable = 3;
). PHP is loosely typed; variables can hold various data types. Operators perform mathematical operations ( , -, , /) and string concatenation (.). Comments (// for single-line, / */ for multi-line) explain code.
Control Structures
-
if
Statements: Execute code blocks conditionally. The==
operator checks for equality.
if ($roll == 6) { echo 'You win!'; }
-
else
Statements: Provide alternative code execution if theif
condition is false. -
Logical Operators:
||
(or),&&
(and) combine conditions. -
Loops: Repeat code blocks.
-
for
Loops: Iterate a known number of times.
<?php echo rand(1, 10); ?>
-
while
Loops: Repeat as long as a condition is true.
echo 'This is a <strong>test</strong>!';
-
do...while
Loops: Execute code at least once, then repeat based on a condition.
-
Arrays
Arrays store multiple values. Elements are accessed by index (starting at 0). Associative arrays use string keys.
if ($roll == 6) { echo 'You win!'; }
User Interaction and Forms
-
Passing Variables in Links: Use URL query strings (e.g.,
name.php?name=Alice
). PHP accesses these via$_GET
.htmlspecialchars()
sanitizes user input to prevent security vulnerabilities (cross-site scripting). -
Passing Variables in Forms: Forms submit data using GET or POST methods. GET appends data to the URL; POST sends data invisibly.
$_POST
array holds POST data.
Hiding the Seams: PHP Templates and Security
Separate PHP logic from HTML using include statements (include __DIR__ . '/../templates/file.html.php';
). This improves maintainability and security. Use absolute paths (with __DIR__
) to avoid issues with relative paths and the current working directory.
Many Templates, One Controller
Controllers manage multiple templates based on user interaction. This example uses a form (form.html.php) and a welcome message (welcome.html.php) template.
Frequently Asked Questions (FAQs)
This section provides answers to common beginner questions about PHP syntax, variable usage, arrays, error handling, form handling, file handling, database interaction, session management, and email sending.
The above is the detailed content of Introducing PHP: A Beginner's Guide. For more information, please follow other related articles on the PHP Chinese website!
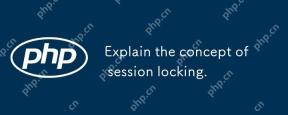
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
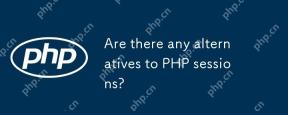
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
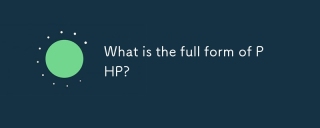
The article discusses PHP, detailing its full form, main uses in web development, comparison with Python and Java, and its ease of learning for beginners.
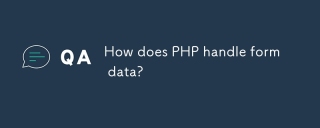
PHP handles form data using $\_POST and $\_GET superglobals, with security ensured through validation, sanitization, and secure database interactions.
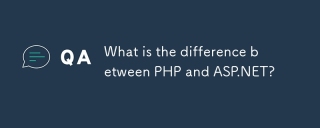
The article compares PHP and ASP.NET, focusing on their suitability for large-scale web applications, performance differences, and security features. Both are viable for large projects, but PHP is open-source and platform-independent, while ASP.NET,
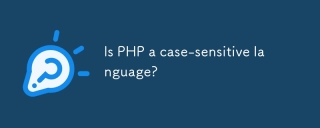
PHP's case sensitivity varies: functions are insensitive, while variables and classes are sensitive. Best practices include consistent naming and using case-insensitive functions for comparisons.
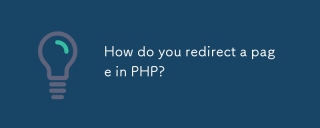
The article discusses various methods for page redirection in PHP, focusing on the header() function and addressing common issues like "headers already sent" errors.
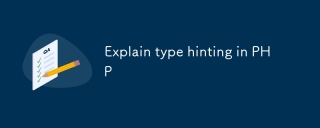
Article discusses type hinting in PHP, a feature for specifying expected data types in functions. Main issue is improving code quality and readability through type enforcement.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
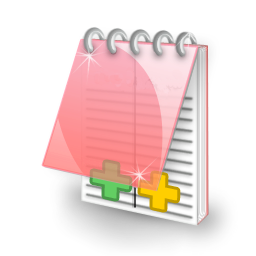
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
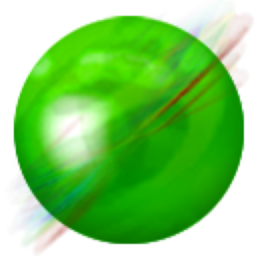
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
