A stack is a data structure that follows the LIFO (Last In, First Out) principle. In other words, The last element we add to a stack is the first one to be removed. When we add (or push) elements to a stack, they are placed on top; i.e. above all the previously-added elements.
There may be certain scenarios where we need to add an element at the bottom of the stack. There are multiple ways to add an element to the bottom of the stack. they are −
- Using Auxiliary Stack
- Using Recursion
- Using temporary variable
- Using a Queue
Using Auxiliary Stack
We can insert an element at the bottom of a stack using an auxiliary stack (a secondary stack using which we will perform operations) in Java. Here, we will use two stacks (a main stack and an auxiliary stack) to insert an element at the bottom of the main stack.
The main stack will have the original elements, while the auxiliary stack will help us to rearrange the elements. This method is easy to understand.
Steps
Following are the steps to insert an element at the bottom of a stack using an auxiliary stack:
- Initialize Two Stacks: Create a main stack push some elements in it and then create an auxiliary stack.
- Pop All Elements: Then remove all elements from the main stack and push them into the second auxiliary stack. This will help us to reverse the order of elements.
- Push the New Element: Once the main stack is empty, we need to push the new element into the main stack or you can also push the element on top of the auxiliary stack if you want.
- Restore the Original Order: Pop all elements from the auxiliary stack and push them back into the main stack. This will restore the original order of elements.
Example
Following is an example of how we can use an auxiliary stack to add an element at the bottom −
import java.util.Stack; public class InsertAtBottomUsingTwoStacks { public static void insertElementAtBottom(Stack<integer> mainStack, int x) { // Create an extra auxiliary stack Stack<integer> St2 = new Stack(); /* Step 1: Pop all elements from the main stack and push them into the auxiliary stack */ while (!mainStack.isEmpty()) { St2.push(mainStack.pop()); } // Step 2: Push the new element into the main stack mainStack.push(x); /* Step 3: Restore the original order by popping each element from the auxiliary stack and push back to main stack */ while (!St2.isEmpty()) { mainStack.push(St2.pop()); } } public static void main(String[] args) { Stack<integer> stack1 = new Stack(); stack1.push(1); stack1.push(2); stack1.push(3); stack1.push(4); System.out.println("Original Stack: " + stack1); insertElementAtBottom(stack1, 0); System.out.println("Stack after inserting 0 at the bottom: " + stack1); } } </integer></integer></integer>
In the program above, we start by pushing the elements 1, 2, 3, and 4 into the stack. Then, we transfer these elements to another stack. After that, we insert the target element into the main stack. Finally, we retrieve all the elements back from the auxiliary stack.
Using Recursion
Recursion is one other way to insert an element at the bottom of a stack. In this approach, we will use a recursive function to pop all the elements from our stack until it becomes empty, and once it becomes empty we will insert the new element into the stack, and then push the elements back into the stack.
Steps
Here are the steps to insert an element at the bottom of a stack using recursion:
- Base Case: Check if the stack is empty. If it is empty, we will push the new element into the stack.
- Recursive Case: If the stack is not empty, we will pop the top element and call the function recursively.
- Restore Elements: After we are done with inserting the new element, we need to push the previously popped elements back into the stack.
Example
import java.util.Stack; public class InsertAtBottomUsingTwoStacks { public static void insertElementAtBottom(Stack<integer> mainStack, int x) { // Create an extra auxiliary stack Stack<integer> St2 = new Stack(); /* Step 1: Pop all elements from the main stack and push them into the auxiliary stack */ while (!mainStack.isEmpty()) { St2.push(mainStack.pop()); } // Step 2: Push the new element into the main stack mainStack.push(x); /* Step 3: Restore the original order by popping each element from the auxiliary stack and push back to main stack */ while (!St2.isEmpty()) { mainStack.push(St2.pop()); } } public static void main(String[] args) { Stack<integer> stack1 = new Stack(); stack1.push(1); stack1.push(2); stack1.push(3); stack1.push(4); System.out.println("Original Stack: " + stack1); insertElementAtBottom(stack1, 0); System.out.println("Stack after inserting 0 at the bottom: " + stack1); } } </integer></integer></integer>
In the above program, we defined a recursive function that inserts a new element at the bottom of the stack, we then continued to pop the elements from the stack until the stack became empty, then we inserted the new element and after that, we restored the previous elements into the stack.
Using Temporary Variable
We can also achieve the given task using a temporary variable. We use this variable to store the elements while we manipulate the stack. This method is easy and we can implement using a simple loop.
Steps
Following are the steps to insert an element at the bottom of a stack using a temporary variable
- Initialize a Temporary Variable: Create a variable to temporarily hold the elements as you iterate through the stack.
- Transfer Elements: Then use a loop to pop elements from the stack and store those elements in the temporary variable.
- Insert New Element: Once our stack is empty, then we need to push the new element into the stack.
- Restore Elements: After inserting the element, push the elements from the temporary variable back into the stack.
Example
import java.util.Stack; public class InsertAtBottomUsingRecursion { public static void insertAtElementBottom(Stack<integer> st, int x) { // Base case: If the stack is empty, push the new element if (st.isEmpty()) { st.push(x); return; } // Recursive case: Pop the top element int top = st.pop(); // Call the function recursively insertAtElementBottom(st, x); // Restore the top element into the stack st.push(top); } public static void main(String[] args) { Stack<integer> st = new Stack(); st.push(1); st.push(2); st.push(3); st.push(4); System.out.println("Original Stack: " + st); insertAtElementBottom(st, 0); System.out.println("Stack after inserting 0 at the bottom: " + st); } } </integer></integer>
In this program, we used a temporary array to hold the elements while manipulating the stack. We then insert the new element into the stack and restore the original elements into the stack.
Using a Queue
In this approach, we will use a queue to hold the elements temporarily while inserting a new element at the bottom of the stack. This method is the better way to manage the order of elements. Using a Queue we can a new element to a stack without tampering with the existing elements.
Steps
Following are the steps to insert an element at the bottom of a stack using a queue −
- Initialize a Queue: Create a queue to hold the elements from the stack.
- Transfer Elements: Pop the elements from the stack and enqueue them into the queue.
- Insert New Element: Push the new element into the stack.
- Restore Elements: Dequeue the elements from the queue and push them back into the stack.
Example
import java.util.Stack; public class InsertAtBottomUsingTempVar { public static void insertAtElementBottom(Stack<integer> st, int x) { // Temporary variable to hold elements int[] temp = new int[st.size()]; int index = 0; // Transfer elements to temporary variable while (!st.isEmpty()) { temp[index++] = st.pop(); } // Push the new element into the stack st.push(x); // Restore elements from temporary variable for (int i = 0; i st = new Stack(); st.push(1); st.push(2); st.push(3); st.push(4); System.out.println("Original Stack: " + st); insertAtElementBottom(st, 0); System.out.println("Stack after inserting 0 at the bottom: " + st); } } </integer>
Output
Following is the output of the above code −
import java.util.Stack; public class InsertAtBottomUsingTwoStacks { public static void insertElementAtBottom(Stack<integer> mainStack, int x) { // Create an extra auxiliary stack Stack<integer> St2 = new Stack(); /* Step 1: Pop all elements from the main stack and push them into the auxiliary stack */ while (!mainStack.isEmpty()) { St2.push(mainStack.pop()); } // Step 2: Push the new element into the main stack mainStack.push(x); /* Step 3: Restore the original order by popping each element from the auxiliary stack and push back to main stack */ while (!St2.isEmpty()) { mainStack.push(St2.pop()); } } public static void main(String[] args) { Stack<integer> stack1 = new Stack(); stack1.push(1); stack1.push(2); stack1.push(3); stack1.push(4); System.out.println("Original Stack: " + stack1); insertElementAtBottom(stack1, 0); System.out.println("Stack after inserting 0 at the bottom: " + stack1); } } </integer></integer></integer>
In this implementation, we used a queue to hold the elements for a temporary time. We first transfer the existing elements from the stack to the queue. Then, we push the new element into the stack and restore the original elements from the queue back to the stack
Note: We can use other data structures such as Array, LinkedList, ArrayList, etc. instead of a queue.
The above is the detailed content of Java Program to insert an element at the Bottom of a Stack. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
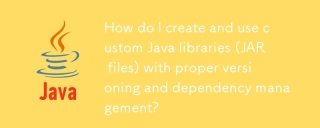
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
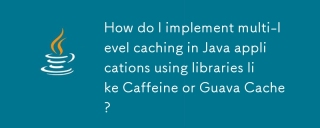
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
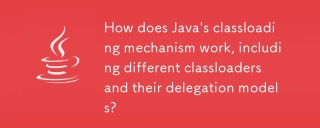
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
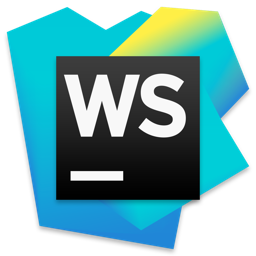
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
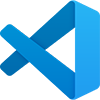
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft