interface in Java is a subinterface of the List
interface, representing an ordered set, allowing duplicate elements. Collection
is a commonly used implementation class for the ArrayList
interface. It is a dynamic array without pre-specifying the size. List
is a class in the Java collection framework, used to store key-value pairs. Keys cannot be repeated, and values can be repeated. HashMap<k v></k>
to ArrayList
: HashMap
- By iteration
-
ArrayList
Using Java 8 Stream API
<code>输入列表:[1="1", 2="2", 3="3"] 输出映射:{1=1, 2=2, 3=3} 输入列表:[1="Java", 2="for", 3="JavaScript"] 输出映射:{1=Java, 2=for, 3=JavaScript} 输入:Mercedes, Audi, BMW, Harley Davidson, Triumph 输出:{Car=[Mercedes, Audi, BMW], Bike=[Harley Davidson, Triumph]}</code>
Algorithm: Convert ArrayList to HashMap
This algorithm describes how to convert to ArrayList
. HashMap
- Step 1: Start.
- Step 2: Declare and import the necessary Java packages.
- Step 3: Create a public list.
- Step 4: Declare key-value pairs.
- Step 5: Create a constructor for the referenced value.
- Step 6: Assign values to the declared key.
- Step 7: Return the private variable id.
- Step 8: Declare a public class and method.
- Step 9: Declare the parameter string.
- Step 10: Create a .
ArrayList
- Step 11: Use data elements to fill the list value.
- Step 12: Create and declare a mapping value.
- Step 13: Declare the object method.
- Step 14: Create an object map value.
- Step 15: Add each data element to the map.
- Step 16: Print the map value and end.
Syntax: Convert ArrayList to HashMap
The following are some sample code snippets:
ArrayList<product> productList = new ArrayList<product>(); productList = getProducts(); Map<string, product> urMap = yourList.stream().collect(Collectors.toMap(Product::getField1, Function.identity())); HashMap<string, product> productMap = new HashMap<string, product>(); for (Product product : productList) { productMap.put(product.getProductCode(), product); } for (Product p: productList) { s.put(p.getName(), p); } for(Product p : productList){ s.put(p.getProductCode() , p); }These snippets show how to convert
to ArrayList
. HashMap
Method
We will introduce two methods:
Method 1: Use iteration and Collectors.toMap()
This method iterates directly
. ArrayList
HashMap
// 示例代码 (假设ArrayList包含自定义对象) class Color { private String name; private String code; // ... getters and setters ... } // ... List<Color> colors = new ArrayList<>(); // ... populate colors list ... Map<String, String> colorMap = colors.stream() .collect(Collectors.toMap(Color::getName, Color::getCode));Method 2: Use Java 8 Stream API and
Collectors.groupingBy()
If you need to group, you can use the method.
Collectors.groupingBy()
Through the above methods, you can choose the appropriate method to convert
// 示例代码 (假设ArrayList包含自定义对象,需要按类别分组) class Product { private String category; private String name; // ... getters and setters ... } // ... List<Product> products = new ArrayList<>(); // ... populate products list ... Map<String, List<Product>> productMap = products.stream() .collect(Collectors.groupingBy(Product::getCategory));according to actual needs. Remember to deal with potential duplicate keys, such as using the third parameter of
to specify the merge function. Select ArrayList
to maintain the insertion order. HashMap
The above is the detailed content of Convert ArrayList to HashMap in Java. For more information, please follow other related articles on the PHP Chinese website!
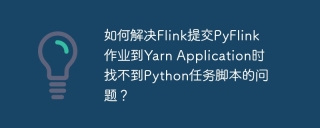
How to solve the problem that Flink cannot find Python task script when submitting PyFlink job to YarnApplication? When you are submitting PyFlink jobs to Yarn using Flink...
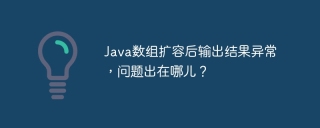
Java array expansion and strange output results This article will analyze a piece of Java code, which aims to achieve dynamic expansion of arrays, but during operation...
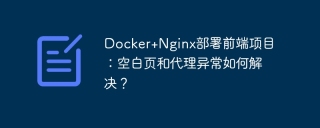
Blank pages and proxy exceptions encountered when deploying front-end projects with Docker Nginx. When using Docker and Nginx to deploy front-end and back-end projects, you often encounter some...
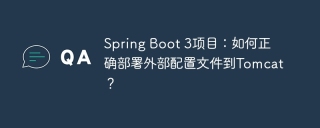
Deployment method of external configuration files of SpringBoot3 project In SpringBoot3 project development, we often need to configure the configuration file application.properties...
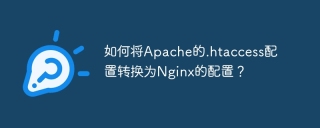
Configuration method for converting Apache's .htaccess configuration to Nginx In project development, you often encounter situations where you need to migrate your server from Apache to Nginx. Ap...
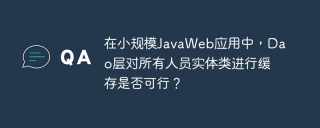
JavaWeb application performance optimization: An exploration of the feasibility of Dao-level entity-class caching In JavaWeb application development, performance optimization has always been the focus of developers. Either...
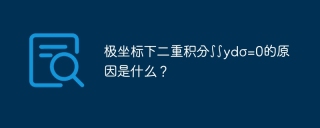
Solving double integrals under polar coordinate system This article will answer a question about double integrals under polar coordinates in detail. The question gives a point area and is incorporated...
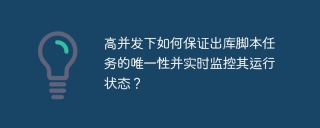
How to ensure the uniqueness of script tasks and monitor their operating status in a high concurrency environment? This article will explore how to ensure an outbound foot in a cluster environment...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
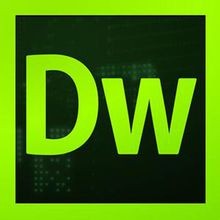
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use