Random sorting of generic lists (such as digital lists) is a common task in programming. In C#, there are multiple methods that can use the built -in method, extension method, and even third -party libraries to achieve this purpose. Understanding the best methods and limitations is essential for gaining the best performance and correctness.
Fisher-Yates shuffle expansion method
One of the most commonly used methods is to use Fisher-Yates to shuffle algorithm. This method randomly disrupts the element of iList
by repeating the elements with random indexes. The following code block demonstrates an extension method to implement the Fisher-Yates algorithm:
private static Random rng = new Random(); public static void Shuffle<T>(this IList<T> list) { int n = list.Count; while (n > 1) { n--; int k = rng.Next(n + 1); T value = list[k]; list[k] = list[n]; list[n] = value; } }To use this expansion method, just call the shuffle () for iList
. For example:
List<Product> products = GetProducts(); products.Shuffle();Use System.Security.Cryptography to improve randomness
Although System.random is convenient, it does not always provide enough randomness. If you need higher -quality randomness, System.Security.Cryptography Library provides a safer random number generator:
using System.Security.Cryptography; ... public static void Shuffle<T>(this IList<T> list) { using (RNGCryptoServiceProvider provider = new RNGCryptoServiceProvider()) { int n = list.Count; while (n > 1) { byte[] box = new byte[1]; do provider.GetBytes(box); while (!(box[0] < (byte)((double)uint.MaxValue / uint.MaxValue * n))); int k = (int)(box[0] % n); T value = list[k]; list[k] = list[n - 1]; list[n - 1] = value; } } }The importance of performance considerations and thread security
The SYSTEM.RANDOM class used in the first example is not thread -safe. This means that if multiple threads try to access the same system.random instance at the same time, incorrect results may occur. To solve this problem, the ThreadSAFRANDOM class provides a thread security solution by using a local random number generator. The modified SHuffle () expansion method of the Threadsaferantom to ensure the correctness in the multi -threaded environment.
Conclusion
In random generic lists, developers can choose between using System.RANDOM (for convenience) or System.Security.Cryptography (for better randomness) Fisher-Yates shuffling methods. In addition, the ThreadsafeRANDOM class helps ensure that thread security in multi -threaded applications. The specific method depends on the required randomness and performance considerations.
The above is the detailed content of How Can I Randomly Shuffle a Generic List in C# Efficiently and Safely?. For more information, please follow other related articles on the PHP Chinese website!
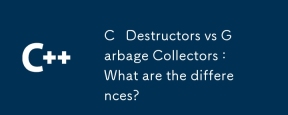
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
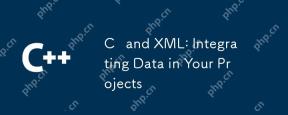
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
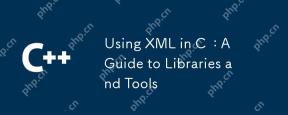
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
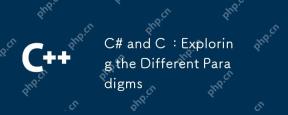
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
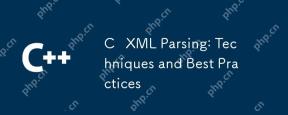
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
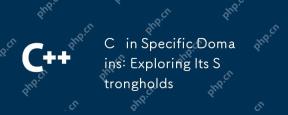
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
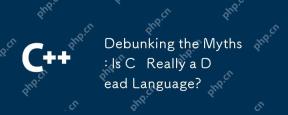
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
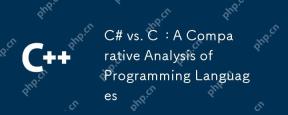
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
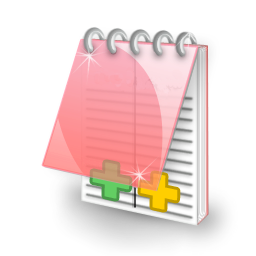
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
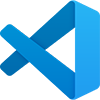
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
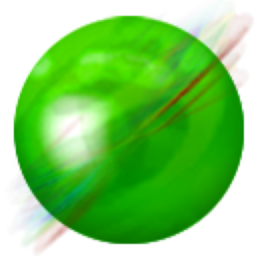
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
