Precise Relative Time Display in C#
Calculating the time elapsed between two dates is a frequent programming task. This article demonstrates accurate relative time representation in C#.
Calculating Time Differences
To determine the difference between two DateTime
values, subtract the earlier date from the later date, resulting in a TimeSpan
object. This object represents the duration in days, hours, minutes, and seconds.
Formatting Relative Time
Several methods exist for formatting the calculated time difference as relative time:
- Constant-Based Approach: Define constants for each time unit (seconds, minutes, hours, days, months, etc.) and use conditional statements to construct the relative time string.
-
String Builder Method: Employ a
StringBuilder
to dynamically create the relative time string based on theTimeSpan
values.
Implementation Example (Constant Approach)
Here's an example using the constant approach:
const int SECOND = 1; const int MINUTE = 60 * SECOND; const int HOUR = 60 * MINUTE; const int DAY = 24 * HOUR; const int MONTH = 30 * DAY; TimeSpan ts = DateTime.UtcNow - yourDate; // Assuming yourDate is the earlier date double delta = Math.Abs(ts.TotalSeconds); string relativeTime; if (delta < MINUTE) { relativeTime = $"{Math.Round(delta)} seconds ago"; } else if (delta < HOUR) { relativeTime = $"{Math.Round(delta / MINUTE)} minutes ago"; } else if (delta < DAY) { relativeTime = $"{Math.Round(delta / HOUR)} hours ago"; } else if (delta < MONTH) { relativeTime = $"{Math.Round(delta / DAY)} days ago"; } else { relativeTime = $"{Math.Round(delta / MONTH)} months ago"; } Console.WriteLine(relativeTime);
This method offers clear, maintainable code, easily extensible to include additional time units or adjust the output format.
The above is the detailed content of How to Accurately Calculate and Display Relative Time in C#?. For more information, please follow other related articles on the PHP Chinese website!
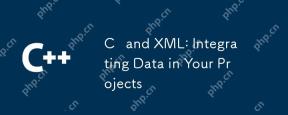
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
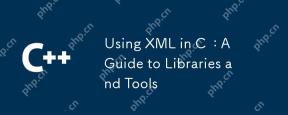
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
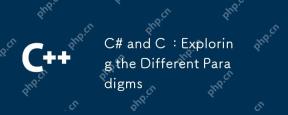
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
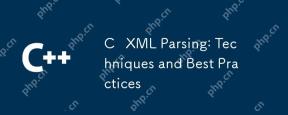
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
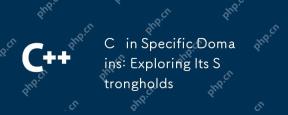
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
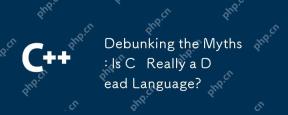
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
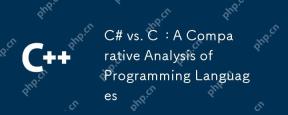
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
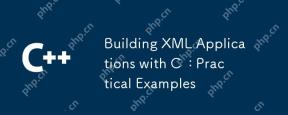
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
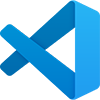
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
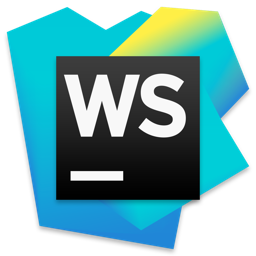
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
