Managing Concurrent Async I/O in .NET
Efficiently managing the number of concurrent asynchronous I/O operations is vital to prevent resource exhaustion. .NET offers several mechanisms to achieve this.
Leveraging SemaphoreSlim
for Asynchronous Concurrency Control
Since .NET 4.5, SemaphoreSlim
's WaitAsync()
method provides robust asynchronous semaphore synchronization. This allows precise control over the number of concurrent async operations.
Here's an example:
public async Task MyOuterMethod() { // Semaphore initialized to allow 20 concurrent operations var throttler = new SemaphoreSlim(20); var tasks = new List<Task>(); foreach (var url in urls) { // Wait for available semaphore slot await throttler.WaitAsync(); tasks.Add(Task.Run(async () => { try { using (var client = new HttpClient()) { var html = await client.GetStringAsync(url); // Process html... } } finally { // Release semaphore slot after completion (success or failure) throttler.Release(); } })); } await Task.WhenAll(tasks); }
TPL-Based Task Scheduling with Concurrency Limits
Alternatively, the Task Parallel Library (TPL) can be used in conjunction with a custom task scheduler to enforce concurrency limits. This involves creating tasks but delaying their execution until a slot becomes available.
Further Points to Consider
-
Custom
TaskScheduler
: MSDN provides examples of creating customTaskScheduler
implementations for fine-grained concurrency management. - Parallel Async Patterns: Employing parallel async patterns like TAP (Task Asynchronous Pattern) or async iterators with async streams can further optimize the execution of concurrent async tasks.
The above is the detailed content of How Can I Limit Concurrent Asynchronous I/O Operations in .NET?. For more information, please follow other related articles on the PHP Chinese website!
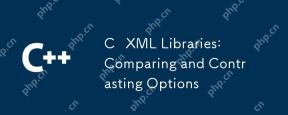
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
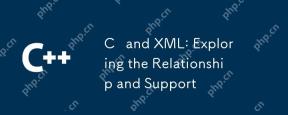
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
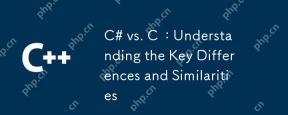
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
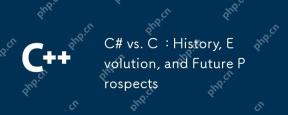
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
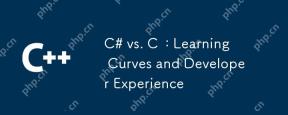
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
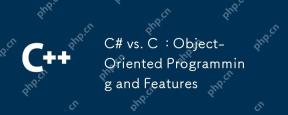
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
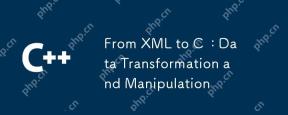
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
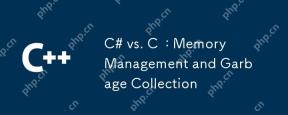
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
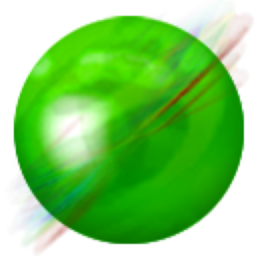
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
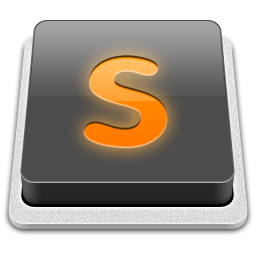
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
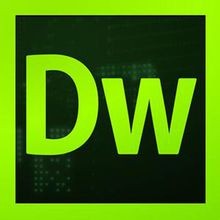
Dreamweaver CS6
Visual web development tools