


How Can I Reliably Determine a File's MIME Type in .NET, Even Without a Valid Extension?
Determining a File's MIME Type in .NET: Bypassing Extension Issues
A common challenge in .NET development involves accurately identifying a file's MIME type, especially when dealing with files lacking proper extensions or having incorrect ones. This often arises with legacy systems or raw data streams.
This article presents a robust solution using the FindMimeFromData
method from urlmon.dll
. This method analyzes the file's signature (the first 256 bytes) to determine its MIME type, providing a reliable result even without a valid extension.
Implementation Steps:
-
Import
urlmon.dll
: UseSystem.Runtime.InteropServices
to import the necessary DLL. -
Declare
FindMimeFromData
: Define the external functionFindMimeFromData
. - Read File Signature: Create a function to read the initial 256 bytes of the file into a byte array. Handle files smaller than 256 bytes gracefully.
-
Call
FindMimeFromData
: Invoke the method, passing the byte array. Anull
MIME type proposal ensures the method relies solely on the file signature.
Code Example:
using System.Runtime.InteropServices; using System.IO; [DllImport("urlmon.dll", CharSet = CharSet.Auto)] static extern uint FindMimeFromData(uint pBC, [MarshalAs(UnmanagedType.LPStr)] string pwzUrl, [MarshalAs(UnmanagedType.LPArray)] byte[] pBuffer, uint cbSize, [MarshalAs(UnmanagedType.LPStr)] string pwzMimeProposed, uint dwMimeFlags, out uint ppwzMimeOut, uint dwReserved); public static string GetMimeType(string filePath) { if (!File.Exists(filePath)) throw new FileNotFoundException($"File not found: {filePath}"); byte[] buffer = new byte[256]; using (FileStream fs = new FileStream(filePath, FileMode.Open)) { int bytesRead = fs.Read(buffer, 0, Math.Min(256, (int)fs.Length)); //Handle files < 256 bytes } uint mimeTypePtr; FindMimeFromData(0, null, buffer, (uint)buffer.Length, null, 0, out mimeTypePtr, 0); IntPtr ptr = new IntPtr(mimeTypePtr); string mimeType = Marshal.PtrToStringUni(ptr); Marshal.FreeCoTaskMem(ptr); return mimeType ?? "unknown/unknown"; //Handle potential null return }
This improved code snippet efficiently handles files of any size and provides a default "unknown/unknown" MIME type if detection fails. This approach ensures reliable MIME type identification, regardless of file extension validity.
The above is the detailed content of How Can I Reliably Determine a File's MIME Type in .NET, Even Without a Valid Extension?. For more information, please follow other related articles on the PHP Chinese website!
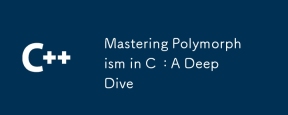
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
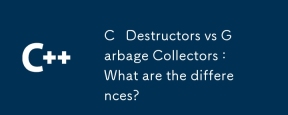
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
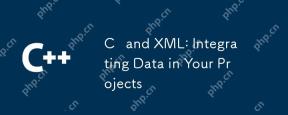
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
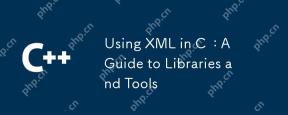
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
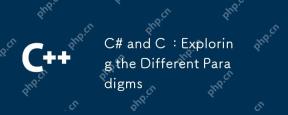
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
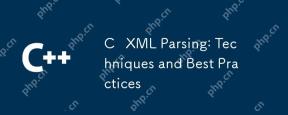
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
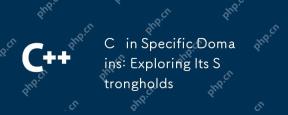
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
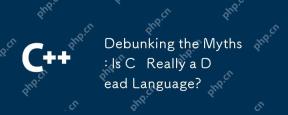
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
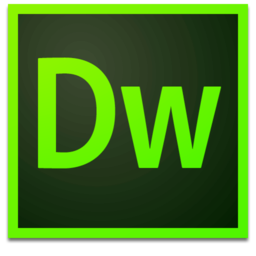
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
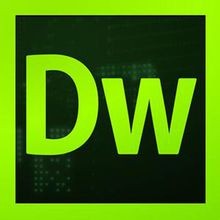
Dreamweaver CS6
Visual web development tools
