Safely Accessing Unity's API from Secondary Threads
The Challenge:
Directly manipulating Unity's API (like updating UI elements) from a background thread often results in errors, such as "GetComponentFastPath can only be called from the main thread." This article outlines a solution for safely executing code on a secondary thread while updating Unity components.
The Solution: Thread-Safe Queuing
Unity's API isn't thread-safe. To avoid crashes, use a queue to marshal operations back to the main thread.
-
The Queue: Create a thread-safe queue (e.g., a
Queue<action></action>
) to store actions intended for the main thread.Action
delegates represent the code to execute. -
Enqueueing from Background Thread: From your secondary thread, add actions to the queue. Use a
lock
statement to protect the queue from race conditions. -
Processing the Queue on the Main Thread: In your main thread's
Update
method (or a similar loop):- Check if the queue contains any actions.
- If so, dequeue and execute them. To avoid blocking the main thread, process a limited number of actions per frame or use a separate coroutine.
C# Code Example:
// Thread-safe queue private readonly Queue<Action> _actionQueue = new Queue<Action>(); // In your secondary thread: lock (_actionQueue) { _actionQueue.Enqueue(() => { // Code to update Unity API (e.g., textComponent.text = "Updated Text"); }); } // In your main thread's Update method: while (_actionQueue.Count > 0) { Action action; lock (_actionQueue) { action = _actionQueue.Dequeue(); } action.Invoke(); }
Best Practices:
-
Error Handling: Wrap your actions in
try-catch
blocks to handle potential exceptions. -
Coroutine for Main Thread Processing: For smoother performance, consider using a coroutine to process the queue instead of doing it all within
Update
. - Alternative Architectures: For complex multithreading scenarios, explore Unity's job system or a dedicated messaging system.
By using this queuing mechanism, you can efficiently perform background tasks and safely update Unity's UI and other components without encountering thread-safety issues.
The above is the detailed content of How Can I Safely Access Unity's API from a Secondary Thread?. For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
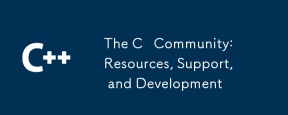
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
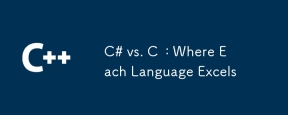
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
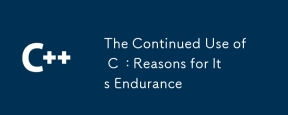
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
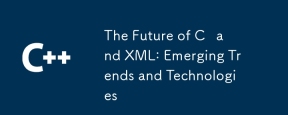
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
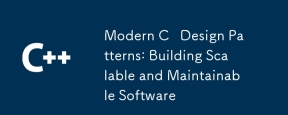
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
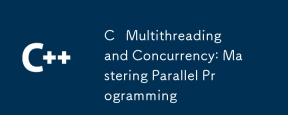
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
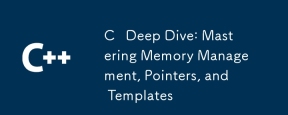
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
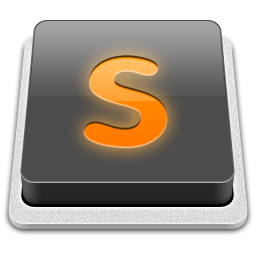
SublimeText3 Mac version
God-level code editing software (SublimeText3)