Python code performance optimization full strategy
Python as a dynamic type interpretation language, the running speed may be slower than the static type compilation language such as C. But through specific techniques and strategies, Python code performance can be significantly improved. This article will discuss how to optimize the Python code to make it run faster and more efficient, and use the timeit
module of Python to accurately measure the code to perform the time.
<:> Note: By default, The module will repeat the code one million times to ensure the accuracy and stability of the measurement result. timeit
measurement timeit
function execution time): print_hi
import timeit def print_hi(name): print(f'Hi, {name}') if __name__ == '__main__': t = timeit.Timer(setup='from __main__ import print_hi', stmt='print_hi("leapcell")') print(t.timeit())
The
provides a high -precision timer in the module, which is suitable for measuring a short time interval. For example:
time
time.perf_counter()
I. I/O -dense operation optimization
import time start_time = time.perf_counter() # ...你的代码逻辑... end_time = time.perf_counter() run_time = end_time - start_time print(f"程序运行时间: {run_time} 秒")
I/O -dense operation refers to the programs or tasks that are spent most of the procedures for the completion of the I/O operation. I/O operations include reading data from disk, data to disk, network communication, etc. These operations usually involve hardware equipment, so its execution speed is limited to hardware performance and I/O bandwidth. The characteristics are as follows:
Waiting time:
When the program executes I/O operation, it is usually necessary to wait for data to transmit data from external devices to memory or transmission from memory to external devices, which may cause program execution blocking.
- Due to the waiting time of I/O operation, the CPU may be in a free state during this period, resulting in low CPU utilization rate. Performance bottleneck: I/O operation speed often becomes a bottleneck of program performance, especially when the data volume is large or the transmission speed is slow.
- For example, perform a million I/O -intensive operations :
- The running result is about 3 seconds. And if you call the empty method of , the program speed will be significantly improved:
CPU utilization:
print
> I/O -dense operation optimization method:
import time import timeit def print_hi(name): print(f'Hi, {name}') return if __name__ == '__main__': start_time = time.perf_counter() t = timeit.Timer(setup='from __main__ import print_hi', stmt='print_hi("leapcell")') t.timeit() end_time = time.perf_counter() run_time = end_time - start_time print(f"程序运行时间: {run_time} 秒")
If necessary (such as file read and write), you can use the following methods to improve efficiency: print
print_hi('xxxx')
def print_hi(name): returnUse
and other asynchronous programming models to allow programs to continue performing other tasks while waiting for the I/O operation to complete, thereby increasing the CPU utilization rate. Cushion:
Use the buffer to temporarily store data to reduce the frequency of I/O operations.Parallel processing:
- Perform multiple I/O operations in parallel to improve the speed processing speed of the overall data.
-
Optimized data structure: Select the appropriate data structure to reduce the number of data read and write times.
asyncio
- 2. Use the generator to generate a list and dictionary In the Python 2.7 and subsequent versions, the list, dictionary, and collector generators have been improved to make the construction process of the data structure more concise and efficient.
- <.> 1. Traditional method:
import timeit def print_hi(name): print(f'Hi, {name}') if __name__ == '__main__': t = timeit.Timer(setup='from __main__ import print_hi', stmt='print_hi("leapcell")') print(t.timeit())
<.> 2. Use the generator optimization:
import time start_time = time.perf_counter() # ...你的代码逻辑... end_time = time.perf_counter() run_time = end_time - start_time print(f"程序运行时间: {run_time} 秒")The method of using the generator is simpler and faster.
Three, avoid string stitching, use
join()
Methods connect the string efficiently, especially when dealing with a large number of string, which is faster than
formatting faster and saving memory. join()
For example:
%
import time import timeit def print_hi(name): print(f'Hi, {name}') return if __name__ == '__main__': start_time = time.perf_counter() t = timeit.Timer(setup='from __main__ import print_hi', stmt='print_hi("leapcell")') t.timeit() end_time = time.perf_counter() run_time = end_time - start_time print(f"程序运行时间: {run_time} 秒")4. Use
instead of the cycle join()
def print_hi(name): returnFunctions are usually more efficient than traditional
cycle.
map()
Traditional cycle method:
map()
Use for
Function:
5. Choose the right data structure
def fun1(): list_ = [] for i in range(100): list_.append(i)
Choosing the appropriate data structure is essential to improve Python code execution efficiency. Dictionary search efficiency is higher than the list (especially under large data volume), but when the amount of small data is the opposite. When frequent and deleted a lot of elements, consider using . When searching frequently, consider using the map()
two -point search.
def fun1(): list_ = [i for i in range(100)]
Reduce unnecessary function calls, merge multiple operations, and improve efficiency.
Seven, avoid unnecessary introduction collections.deque
bisect
Reduce unnecessary module import and reduce expenses.
8. Avoid using global variables
Put the code inside the function, which can usually increase the speed.Nine, avoid module and function attribute access
Use to avoid the expenses of attribute access.
Ten, reduce the calculation in the inner cycle
Calculate the values that can be calculated in advance in the loop in advance to reduce duplicate calculations.
(here is a omitted introduction to the Leapcell platform, because it has nothing to do with Python code performance optimization)
Please note that the above optimization methods are not always applicable, and the appropriate optimization strategy needs to be selected according to the specific situation. Performance and testing the code can find the most effective optimization solution.
The above is the detailed content of Python Performance Tips You Must Know. For more information, please follow other related articles on the PHP Chinese website!
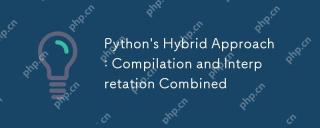
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
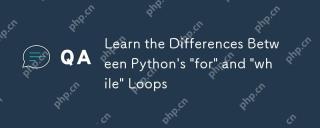
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
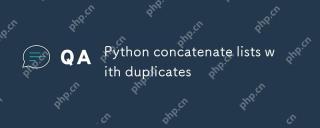
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.
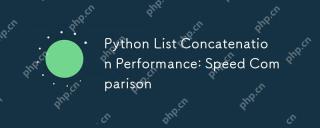
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
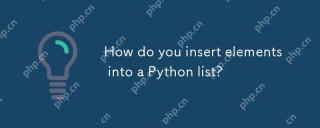
ToinsertelementsintoaPythonlist,useappend()toaddtotheend,insert()foraspecificposition,andextend()formultipleelements.1)Useappend()foraddingsingleitemstotheend.2)Useinsert()toaddataspecificindex,thoughit'sslowerforlargelists.3)Useextend()toaddmultiple
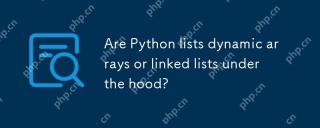
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
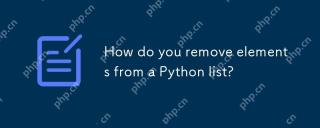
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
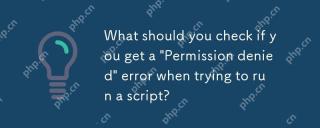
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
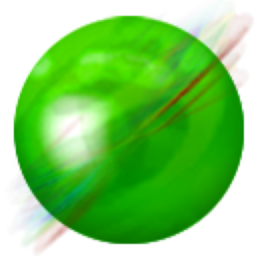
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
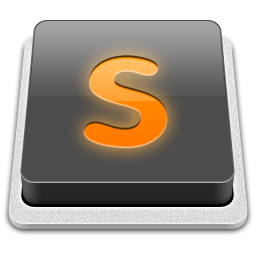
SublimeText3 Mac version
God-level code editing software (SublimeText3)
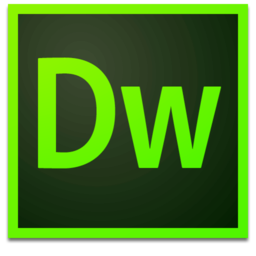
Dreamweaver Mac version
Visual web development tools
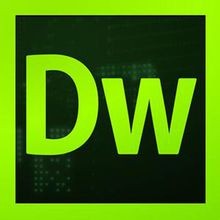
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
