


How Can I Deserialize JSON Objects with Non-Default Constructors Using Json.net?
Json.NET: Deserializing Objects with Non-Default Constructors
Json.NET typically uses the default constructor for deserialization. However, if your class has a non-default constructor that's necessary for other reasons, you can still use Json.NET to deserialize using your preferred constructor.
Using the [JsonConstructor]
Attribute
The simplest solution is to mark your desired constructor with the [JsonConstructor]
attribute. This tells Json.NET to use this constructor during deserialization. Make sure the constructor parameter names match the JSON property names (case-insensitive).
[JsonConstructor] public Result(int? code, string format, Dictionary<string, string> details = null) { // ... }
Creating a Custom JsonConverter
If you can't modify the source code directly, you can create a custom JsonConverter
.
class ResultConverter : JsonConverter { // ... implementation details ... }
Then, add the converter to your serializer settings:
JsonSerializerSettings settings = new JsonSerializerSettings(); settings.Converters.Add(new ResultConverter()); Result result = JsonConvert.DeserializeObject<Result>(jsonText, settings);
This approach provides more control over the deserialization process without altering the original class definition. The JsonConverter
allows for flexible object creation and property population.
The above is the detailed content of How Can I Deserialize JSON Objects with Non-Default Constructors Using Json.net?. For more information, please follow other related articles on the PHP Chinese website!
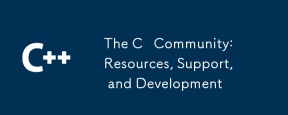
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
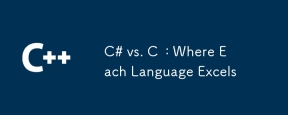
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
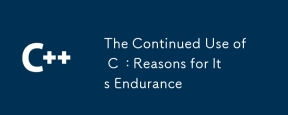
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
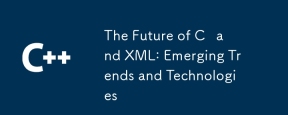
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
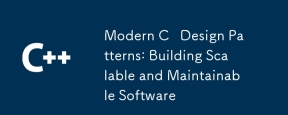
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
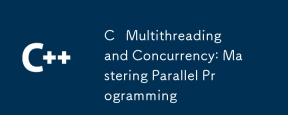
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
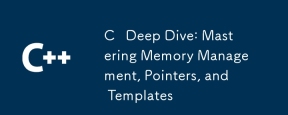
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
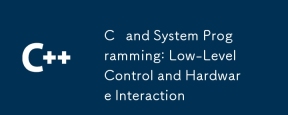
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
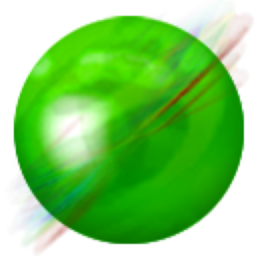
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
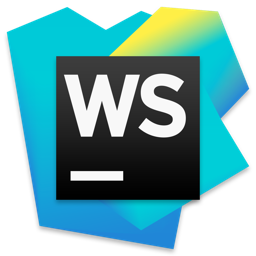
WebStorm Mac version
Useful JavaScript development tools
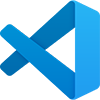
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft