


How Can a Generic Retry Function Improve Code Maintainability and Resilience?
Robust and Reusable Retry Logic
Handling intermittent failures in operations requires a reliable retry mechanism. Instead of repetitive, manual retry loops, a generic retry function offers a superior solution for improved code maintainability and resilience.
A Universal Retry Function
This approach introduces a versatile retry function, TryThreeTimes()
(or a more descriptive name), that simplifies retry logic for any method. The function allows specification of retry parameters: the number of attempts, the interval between retries, and the method to execute.
Implementing the Retry Function
The core retry function can be implemented as follows:
public static class RetryHelper { public static void Execute( Action action, TimeSpan retryInterval, int maxAttemptCount = 3) { // ... implementation details ... } public static T Execute<T>( Func<T> action, TimeSpan retryInterval, int maxAttemptCount = 3) { // ... implementation details ... } }
The Execute
method iterates through the specified retry attempts, pausing between each. Any exceptions encountered are collected. After all attempts, if failures occurred, an AggregateException
is thrown, providing details on all exceptions.
Utilizing the Retry Function
Using the retry function is straightforward:
RetryHelper.Execute(() => SomeFunctionThatMightFail(), TimeSpan.FromSeconds(1));
RetryHelper.Execute(SomeFunctionThatMightFail, TimeSpan.FromSeconds(1));
int result = RetryHelper.Execute(SomeFunctionReturningInt, TimeSpan.FromSeconds(1), 4);
Key Advantages
This generic retry approach provides several benefits:
- Enhanced Maintainability: Centralizes retry logic, improving code organization and reducing redundancy.
- Reduced Code Complexity: Eliminates the need for repeated, manual retry code blocks.
- Flexibility and Extensibility: Allows easy customization of retry parameters to suit various scenarios.
By employing a generic retry function, developers can create more robust, maintainable, and resilient applications. This approach promotes cleaner code and simplifies the handling of transient errors.
The above is the detailed content of How Can a Generic Retry Function Improve Code Maintainability and Resilience?. For more information, please follow other related articles on the PHP Chinese website!
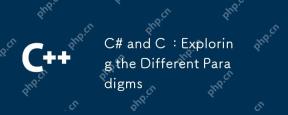
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
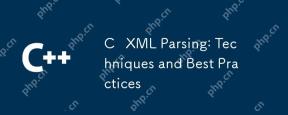
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
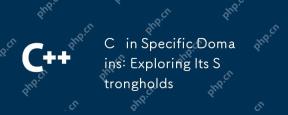
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
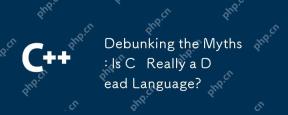
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
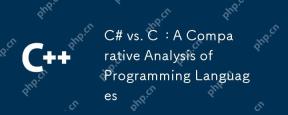
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
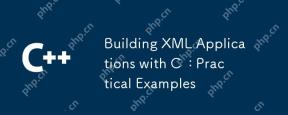
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
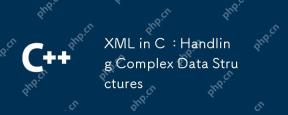
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
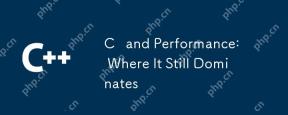
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
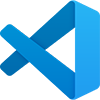
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
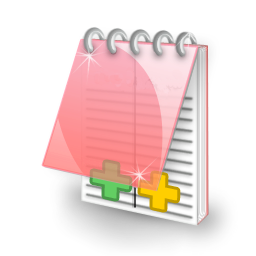
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
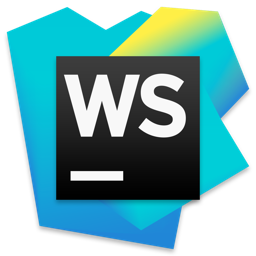
WebStorm Mac version
Useful JavaScript development tools
