This guide details the process of creating a JAR file from a Java source file. We'll cover each step with explanations and examples.
Step 1: Java Code Creation
Create a .java
file containing your Java code. For instance, a file named Main.java
might look like this:
public class Main { public static void main(String[] args) { System.out.println("Hello, World!"); } }
This is your source code, adhering to Java's syntax and rules.
Step 2: Compilation
Compile the .java
file using the Java compiler (javac
):
javac Main.java
This generates a .class
file (e.g., Main.class
) containing bytecode – machine-readable instructions for the Java Virtual Machine (JVM). Each .java
file produces a corresponding .class
file.
Step 3: Manifest File (Optional)
Create a MANIFEST.MF
file (optional but recommended) to define JAR metadata. For example:
<code>Main-Class: Main</code>
Main-Class
specifies the application's entry point (the class with the main
method). This simplifies JAR execution.
Step 4: JAR File Packaging
Use the jar
command to package the .class
file(s), resources, and (optionally) the manifest file into a JAR:
jar cvfm MyApplication.jar MANIFEST.MF Main.class
-
c
: Creates a new JAR. -
v
: Enables verbose output (shows the packaging process). -
f
: Specifies the output JAR filename (MyApplication.jar
). -
m
: Includes the manifest file (MANIFEST.MF
).
The jar
tool creates a single, portable archive (MyApplication.jar
) containing all compiled components.
Step 5: JAR File Testing
Run the JAR file to verify its functionality:
java -jar MyApplication.jar
Successful execution should produce the output:
<code>Hello, World!</code>
The JVM uses the MANIFEST.MF
(if present) to locate the Main-Class
and execute its main
method.
Step 6: JAR File Deployment
Deployment depends on the target environment:
-
Standalone: Copy the JAR to the target machine and run it using
java -jar
. -
Microservices (e.g., Spring Boot): Deploy the "fat JAR" (containing an embedded server) using
java -jar
. - Containerized (Docker): Use a Dockerfile:
FROM openjdk:17 COPY MyApplication.jar /app/MyApplication.jar WORKDIR /app CMD ["java", "-jar", "MyApplication.jar"]
Build (docker build -t my-java-app .
) and run (docker run -p 8080:8080 my-java-app
) the container.
- Cloud: Deploy to cloud platforms (AWS, GCP, Azure) via CI/CD or cloud-specific services.
Execution Summary:
- Write Java code (
.java
). - Compile to
.class
files usingjavac
. - (Optional) Create
MANIFEST.MF
. - Package into a JAR using
jar
. - Test the JAR using
java -jar
. - Deploy to the appropriate environment.
The above is the detailed content of Journey From Java file to a JAR file. For more information, please follow other related articles on the PHP Chinese website!
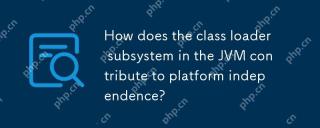
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
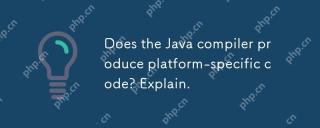
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
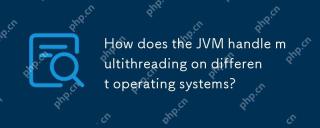
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
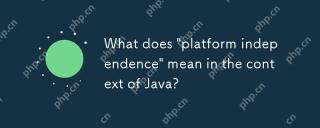
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
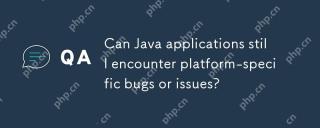
Javaapplicationscanindeedencounterplatform-specificissuesdespitetheJVM'sabstraction.Reasonsinclude:1)Nativecodeandlibraries,2)Operatingsystemdifferences,3)JVMimplementationvariations,and4)Hardwaredependencies.Tomitigatethese,developersshould:1)Conduc
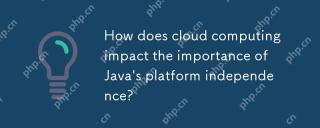
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
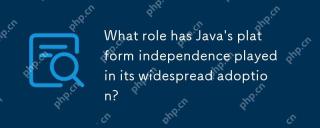
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
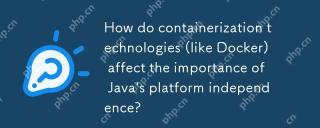
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
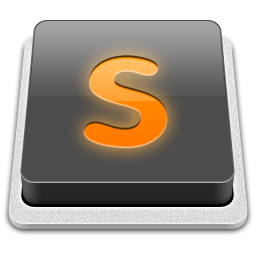
SublimeText3 Mac version
God-level code editing software (SublimeText3)