- Use field parameters
For example, ensure that the maximum length of the
field is 100 characters. Since it is first_name
, you can use CharField
Parameters: max_length
from django import forms class PersonalInfo(forms.Form): first_name = forms.CharField(max_length=100)This is one of the simplest and most commonly used verification methods.
- Use the built -in verification device
Assume that the minimum value of the
field is 18. Although the parameters can be used directly, how to use the age
: min_value
MinValueValidator
from django import forms from django.core.validators import MinValueValidator class PersonalInfo(forms.Form): first_name = forms.CharField(max_length=100) age = forms.IntegerField(validators=[MinValueValidator(18)])module provides multiple pre -constructed verifications for minimum/maximum value inspection, regular expression verification and other tasks.
validators
- Writing a custom verification device
-
The verification device is a callable object. It receives a value. If the value does not meet the conditions, it will cause
. For example, create a verification device to ensure the number of numbers:
ValidationError
from django import forms from django.core.exceptions import ValidationError from django.core.validators import MinValueValidator def validate_even(value): if value % 2 != 0: raise ValidationError(f"{value} 不是偶数") class PersonalInfo(forms.Form): first_name = forms.CharField(max_length=100) age = forms.IntegerField(validators=[MinValueValidator(18)]) even_field = forms.IntegerField(validators=[validate_even])In order to keep the code orderly, you can consider putting the custom verification device in a separate file, such as
. This depends on the scale of the project.
validators.py
-
clean_<fieldname>()</fieldname>
Another powerful field verification method is to rewrite the
field directly in the form: clean_<fieldname>()</fieldname>
This method can directly access the cleaning data of the field and allow more detailed control verification. even_field
from django import forms from django.core.exceptions import ValidationError from django.core.validators import MinValueValidator class PersonalInfo(forms.Form): first_name = forms.CharField(max_length=100) age = forms.IntegerField(validators=[MinValueValidator(18)]) even_field = forms.IntegerField() def clean_even_field(self): even_field_validated = self.cleaned_data.get("even_field") if even_field_validated % 2 != 0: raise ValidationError(f"{even_field_validated} 不是偶数") return even_field_validated
Method
-
Sometimes, verification needs to consider the relationship between multiple fields in the form. For example, if there are two fields, there are more characters in one field than another field. This type of verification is
, because it depends on the value of multiple fields, not just a single field.clean()
Form -level verification
method in the form class.
By rewriting the method, you can realize the custom logic logic of the entire form to ensure that the data meets more complicated requirements.
clean()
Summary
from django import forms from django.core.exceptions import ValidationError from django.core.validators import MinValueValidator class PersonalInfo(forms.Form): first_name = forms.CharField(max_length=100) age = forms.IntegerField(validators=[MinValueValidator(18)]) even_field = forms.IntegerField() field1 = forms.CharField() field2 = forms.CharField() def clean_even_field(self): even_field_validated = self.cleaned_data.get("even_field") if even_field_validated % 2 != 0: raise ValidationError(f"{even_field_validated} 不是偶数") return even_field_validated def clean(self): # 表单级验证 cleaned_data = super().clean() field1_value = cleaned_data.get("field1") field2_value = cleaned_data.get("field2") if field1_value and field2_value and len(field1_value) >= len(field2_value): raise ValidationError("field2 字符数必须多于 field1.") return cleaned_data
- Field parameters: Use
max_length
ormin_value
and other parameters for simple verification. - Built -in verification device: Use Django's predetermined verification device to handle common mode.
- Custom verification device: Written reusable callable objects for complex verification rules.
- Method: Rewrite this method for high -level specific field verification.
clean_<fieldname>()</fieldname>
Method: Use this method to perform form -level verification involving multiple fields. -
clean()
The above is the detailed content of Techniques for Field Validation in Django. For more information, please follow other related articles on the PHP Chinese website!
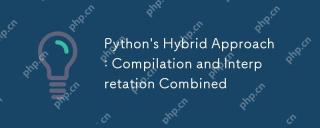
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
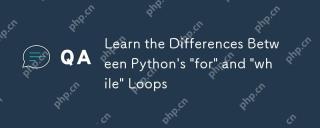
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
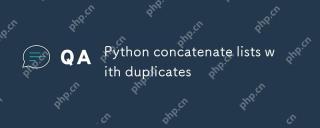
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.
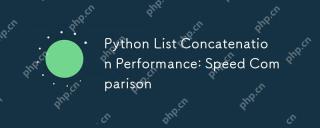
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
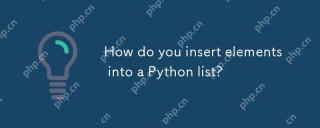
ToinsertelementsintoaPythonlist,useappend()toaddtotheend,insert()foraspecificposition,andextend()formultipleelements.1)Useappend()foraddingsingleitemstotheend.2)Useinsert()toaddataspecificindex,thoughit'sslowerforlargelists.3)Useextend()toaddmultiple
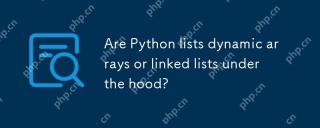
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
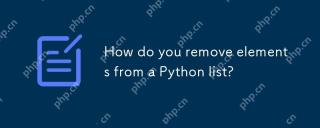
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
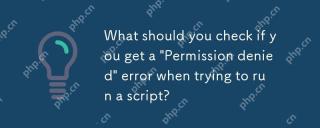
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
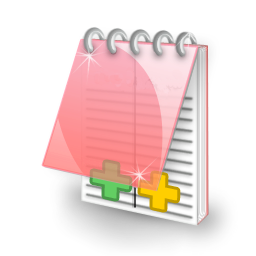
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
