Configuring Cross-Origin Resource Sharing (CORS) in ASP.NET Core Web API
This guide demonstrates two approaches to enable CORS in your ASP.NET Core Web API: using middleware and a manual header injection method.
Method 1: Middleware-Based CORS Configuration
The simplest and recommended approach is to leverage the Microsoft.AspNetCore.Cors
NuGet package.
-
Install the Package:
<code>Install-Package Microsoft.AspNetCore.Cors</code>
-
Configure CORS Services:
Within your
Startup.cs
file, register the CORS service:public void ConfigureServices(IServiceCollection services) { services.AddCors(); // ... other service configurations }
-
Use CORS Middleware:
In the
Configure
method, utilize theapp.UseCors
middleware to define allowed origins and HTTP methods. Replace"http://example.com"
with your actual allowed origin(s).public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ... ) { app.UseCors(options => options.WithOrigins("http://example.com").AllowAnyMethod()); // ... other middleware configurations }
Method 2: Manual Header Injection (Fallback Method)
If the middleware approach proves ineffective, you can directly add CORS headers to your HTTP responses. This is generally less preferred due to reduced maintainability.
app.Use(async (context, next) => { context.Response.Headers.Add("Access-Control-Allow-Origin", "http://example.com"); context.Response.Headers.Add("Access-Control-Allow-Methods", "GET, POST, PUT, PATCH, DELETE"); context.Response.Headers.Add("Access-Control-Allow-Headers", "X-PINGOTHER, Content-Type, Authorization"); await next.Invoke(); });
Remember to position this middleware before app.UseRouting()
or equivalent middleware handling routing.
Important Considerations:
- *Wildcard Origins (`
):** Avoid using the wildcard
"*"for
WithOrigins` in production environments. This opens your API to requests from any origin, posing a significant security risk. -
Specific Headers: Carefully define the allowed headers using
AddCustomHeader
or the equivalent in your chosen method. Always include"Content-Type"
. -
Advanced Configurations: For more granular control, explore the CORS policy model offered by the
Microsoft.AspNetCore.Cors
package. This allows for named policies and more complex scenarios.
This enhanced guide provides a clearer explanation and improved structure for implementing CORS in ASP.NET Core Web API. Choose the method that best suits your needs and prioritize security best practices.
The above is the detailed content of How to Enable CORS in ASP.NET Core Web API?. For more information, please follow other related articles on the PHP Chinese website!
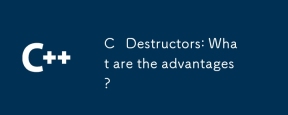
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
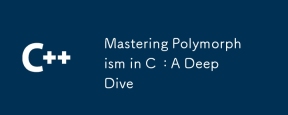
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
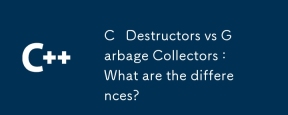
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
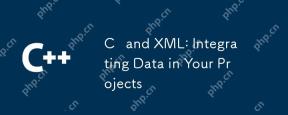
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
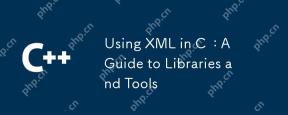
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
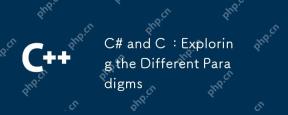
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
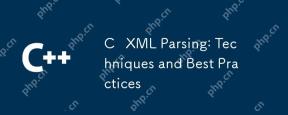
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
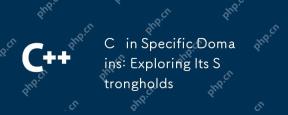
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
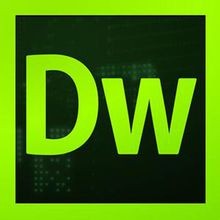
Dreamweaver CS6
Visual web development tools
