Explore the strange corners of C# and the .NET framework
In software development, we often encounter some puzzling edge cases. This article will explore some of the most bizarre edge cases in C# and the .NET framework, revealing their anomalous behavior.
String resident exception
Consider the following code snippet:
string x = new string(new char[0]); string y = new string(new char[0]); Console.WriteLine(object.ReferenceEquals(x, y));
Intuitively, we would expect the output to be False since the "new" keyword usually allocates space for a new object. Surprisingly, this code outputs True in all framework versions tested. Although the specification states that new objects should always be created, this becomes a special case.
Nullable type exception
This code further illustrates the complexity of C#:
static void Foo<T>() where T : new() { T t = new T(); // ... (其他操作) // 此行引发NullReferenceException Console.WriteLine(t.GetType()); }
The mystery lies in type T. To uncover it, we have to examine the code carefully. The Nullable<t></t>
structure represents a nullable value type, which overrides most member methods, but does not override GetType()
. So when GetType()
is called, the nullable value is cast to object
(resulting in null), resulting in NullReferenceException
.
Class instantiation trick
Another puzzling edge case occurs in the following code, where T is restricted to a reference type:
private static void Main() { CanThisHappen<MyFunnyType>(); } public static void CanThisHappen<T>() where T : class, new() { var instance = new T(); Debug.Assert(instance != null, "我们是如何破坏CLR的?"); }
Through excellent engineering design, this code can be broken using indirect methods similar to remote calls:
class MyFunnyProxyAttribute : ProxyAttribute { // ... (重写) } [MyFunnyProxy] class MyFunnyType : ContextBoundObject { }
By defining a custom proxy attribute and redirecting the new()
call to return null, the assertion in CanThisHappen
is broken, demonstrating the tremendous flexibility and potential pitfalls in the C# language and .NET runtime.
The above is the detailed content of What Are Some Unexpected Behaviors and Corner Cases in C# and .NET?. For more information, please follow other related articles on the PHP Chinese website!
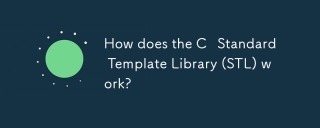
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
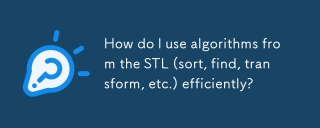
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
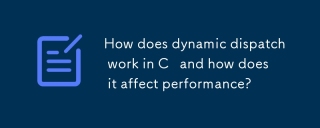
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
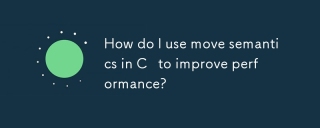
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
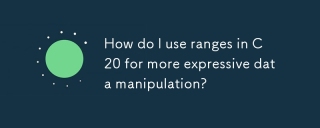
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
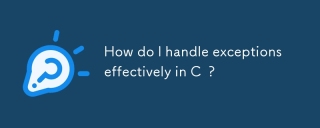
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
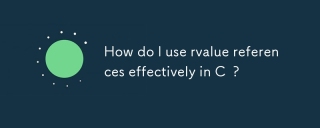
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
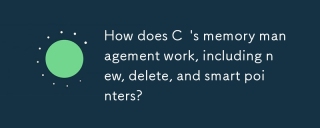
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
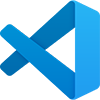
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
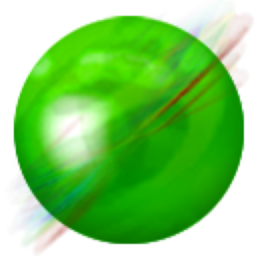
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
