Web scraping—the art of extracting online data—is a powerful technique for research, analysis, and automation. Python offers various libraries for this purpose, but cURL, accessed via PycURL, stands out for its speed and precision. This guide demonstrates how to leverage cURL's capabilities within Python for efficient web scraping. We'll also compare it to popular alternatives like Requests, HTTPX, and AIOHTTP.
Understanding cURL
cURL is a command-line tool for sending HTTP requests. Its speed, flexibility, and support for various protocols make it a valuable asset. Basic examples:
GET request: curl -X GET "https://httpbin.org/get"
POST request: curl -X POST "https://httpbin.org/post"
PycURL enhances cURL's power by providing fine-grained control within your Python scripts.
Step 1: Installing PycURL
Install PycURL using pip:
pip install pycurl
Step 2: GET Requests with PycURL
Here's how to perform a GET request using PycURL:
import pycurl import certifi from io import BytesIO buffer = BytesIO() c = pycurl.Curl() c.setopt(c.URL, 'https://httpbin.org/get') c.setopt(c.WRITEDATA, buffer) c.setopt(c.CAINFO, certifi.where()) c.perform() c.close() body = buffer.getvalue() print(body.decode('iso-8859-1'))
This code demonstrates PycURL's ability to manage HTTP requests, including setting headers and handling SSL certificates.
Step 3: POST Requests with PycURL
POST requests, crucial for form submissions and API interactions, are equally straightforward:
import pycurl import certifi from io import BytesIO buffer = BytesIO() c = pycurl.Curl() c.setopt(c.URL, 'https://httpbin.org/post') post_data = 'param1=python¶m2=pycurl' c.setopt(c.POSTFIELDS, post_data) c.setopt(c.WRITEDATA, buffer) c.setopt(c.CAINFO, certifi.where()) c.perform() c.close() body = buffer.getvalue() print(body.decode('iso-8859-1'))
This example showcases sending data with a POST request.
Step 4: Custom Headers and Authentication
PycURL allows you to add custom headers for authentication or user-agent simulation:
import pycurl import certifi from io import BytesIO buffer = BytesIO() c = pycurl.Curl() c.setopt(c.URL, 'https://httpbin.org/get') c.setopt(c.HTTPHEADER, ['User-Agent: MyApp', 'Accept: application/json']) c.setopt(c.WRITEDATA, buffer) c.setopt(c.CAINFO, certifi.where()) c.perform() c.close() body = buffer.getvalue() print(body.decode('iso-8859-1'))
This illustrates the use of custom headers.
Step 5: Handling XML Responses
PycURL efficiently handles XML responses:
import pycurl import certifi from io import BytesIO import xml.etree.ElementTree as ET buffer = BytesIO() c = pycurl.Curl() c.setopt(c.URL, 'https://www.google.com/sitemap.xml') c.setopt(c.WRITEDATA, buffer) c.setopt(c.CAINFO, certifi.where()) c.perform() c.close() body = buffer.getvalue() root = ET.fromstring(body.decode('utf-8')) print(root.tag, root.attrib)
This shows XML parsing directly within your workflow.
Step 6: Robust Error Handling
Error handling is crucial for reliable scraping:
import pycurl import certifi from io import BytesIO buffer = BytesIO() c = pycurl.Curl() c.setopt(c.URL, 'https://example.com') c.setopt(c.WRITEDATA, buffer) c.setopt(c.CAINFO, certifi.where()) try: c.perform() except pycurl.error as e: errno, errstr = e.args print(f"Error: {errstr} (errno {errno})") finally: c.close() body = buffer.getvalue() print(body.decode('iso-8859-1'))
This code ensures graceful error handling.
Step 7: Advanced Features: Cookies and Timeouts
PycURL supports advanced features like cookies and timeouts:
import pycurl import certifi from io import BytesIO buffer = BytesIO() c = pycurl.Curl() c.setopt(c.URL, 'http://httpbin.org/cookies') c.setopt(c.COOKIE, 'user_id=12345') c.setopt(c.TIMEOUT, 30) c.setopt(c.WRITEDATA, buffer) c.setopt(c.CAINFO, certifi.where()) c.perform() c.close() body = buffer.getvalue() print(body.decode('utf-8'))
This example demonstrates using cookies and setting timeouts.
Step 8: PycURL vs. Other Libraries
PycURL offers superior performance and flexibility, but has a steeper learning curve and lacks asynchronous support. Requests is user-friendly but less performant. HTTPX and AIOHTTP excel in asynchronous operations and modern protocol support. Choose the library that best suits your project's needs and complexity.
Conclusion
PycURL provides a powerful combination of speed and control for advanced web scraping tasks. While it requires a deeper understanding than simpler libraries, the performance benefits make it a worthwhile choice for demanding projects.
The above is the detailed content of Unlocking the Benefits of Using cURL with Python. For more information, please follow other related articles on the PHP Chinese website!
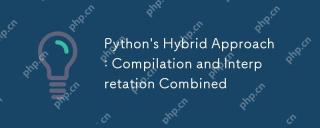
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
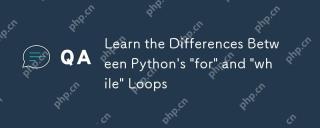
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
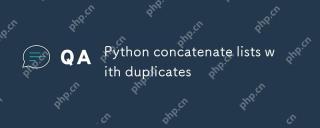
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.
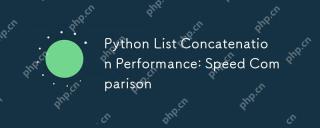
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
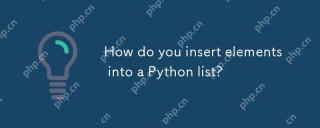
ToinsertelementsintoaPythonlist,useappend()toaddtotheend,insert()foraspecificposition,andextend()formultipleelements.1)Useappend()foraddingsingleitemstotheend.2)Useinsert()toaddataspecificindex,thoughit'sslowerforlargelists.3)Useextend()toaddmultiple
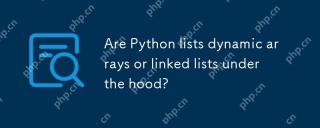
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
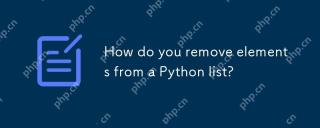
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
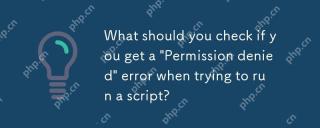
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
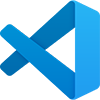
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
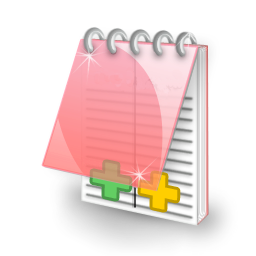
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
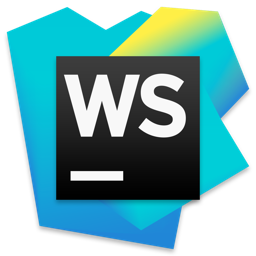
WebStorm Mac version
Useful JavaScript development tools
