The Java Singleton pattern is a widely used design pattern that guarantees a class has only one instance and provides a global access point to it. Think of it as a single manager overseeing a team – there's only one, and everyone interacts through that single point of contact. This article will break down the pattern's implementation and various approaches in Java.
Why Use the Singleton Pattern?
- Guaranteed Single Instance: Ensures only one instance of a class exists within your application. This is crucial for managing resources like database connections (avoiding excessive connection creation and destruction) or printer spoolers (preventing conflicts between users).
- Global Access Point: Provides a single, readily available access point to the instance.
- Resource Management: Efficiently manages shared resources such as configurations, logging systems, or thread pools.
How to Implement the Singleton Pattern in Java
Several approaches exist, each with its own trade-offs:
1. Lazy Initialization: Creates the instance only when it's first needed.
import java.io.Serializable; public class LazySingleton implements Serializable { private static LazySingleton instance; private LazySingleton() { if (instance != null) { throw new IllegalStateException("Instance already created"); } } public static LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } private Object readResolve() { return getInstance(); } }
2. Thread-Safe Singleton: Uses synchronized
to ensure thread safety during instance creation.
import java.io.*; public class ThreadSafeSingleton implements Serializable { private static final long serialVersionUID = 1L; private static ThreadSafeSingleton instance; private ThreadSafeSingleton() { if (instance != null) { throw new IllegalStateException("Instance already created"); } } public static synchronized ThreadSafeSingleton getInstance() { if (instance == null) { instance = new ThreadSafeSingleton(); } return instance; } private Object readResolve() { return getInstance(); } }
3. Double-Checked Locking: Optimizes thread safety by minimizing synchronization overhead.
import java.io.*; public class DoubleCheckedLockingSingleton implements Serializable { private static final long serialVersionUID = 1L; private static volatile DoubleCheckedLockingSingleton instance; private DoubleCheckedLockingSingleton() { if (instance != null) { throw new IllegalStateException("Instance already created"); } } public static DoubleCheckedLockingSingleton getInstance() { if (instance == null) { synchronized (DoubleCheckedLockingSingleton.class) { if (instance == null) { instance = new DoubleCheckedLockingSingleton(); } } } return instance; } private Object readResolve() { return getInstance(); } }
4. Bill Pugh Singleton (Recommended): Uses a static inner class to ensure lazy initialization and thread safety.
import java.io.*; public class BillPughSingleton implements Serializable { private static final long serialVersionUID = 1L; private BillPughSingleton() { if (SingletonHelper.INSTANCE != null) { throw new IllegalStateException("Instance already created"); } } private static class SingletonHelper { private static final BillPughSingleton INSTANCE = new BillPughSingleton(); } public static BillPughSingleton getInstance() { return SingletonHelper.INSTANCE; } private Object readResolve() { return getInstance(); } }
5. Enum Singleton (Modern Approach): Leverages the inherent thread safety and serialization handling of enums. This is often considered the best approach for its simplicity and robustness.
public enum EnumSingleton { INSTANCE; public void showMessage() { System.out.println("Hello from Enum Singleton!"); } }
Summary of Singleton Implementations
- Lazy Initialization: Simple, but requires additional handling for thread safety and serialization.
- Thread-Safe Singleton: Thread-safe, but can have performance overhead due to synchronization.
- Double-Checked Locking: Improves performance over the basic thread-safe approach.
- Bill Pugh Singleton: Elegant and efficient, often preferred for its simplicity and thread safety.
- Enum Singleton: The most concise and robust solution, leveraging Java's built-in features. Generally recommended for modern Java development.
The above is the detailed content of Understanding the Singleton Pattern in Java. For more information, please follow other related articles on the PHP Chinese website!
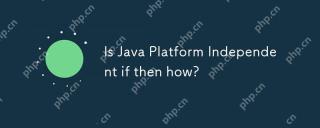
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
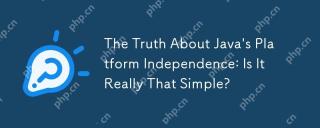
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
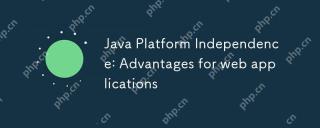
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
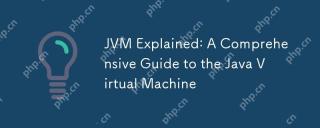
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
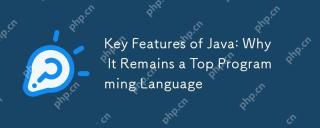
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
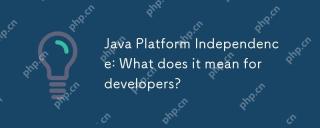
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
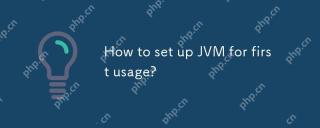
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
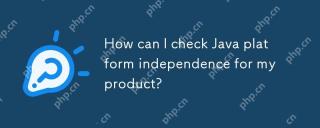
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
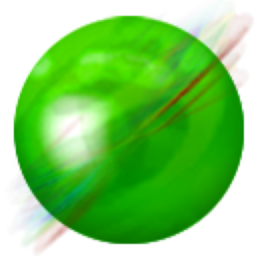
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
