


How to Fix SQL Syntax Errors When Using `bindValue` with LIMIT Clause in PHP?
Problem: SQL Syntax Errors Using bindValue
with LIMIT in PHP
A common issue arises when using PHP's bindValue
method with a MySQL LIMIT
clause. The problem stems from PHP potentially quoting the LIMIT parameters, leading to incorrect SQL syntax.
Solution: Explicit Integer Casting
The solution is straightforward: explicitly cast the LIMIT
parameters to integers before binding them. This prevents PHP from adding unwanted quotes.
Corrected Code:
Here's the improved code snippet:
$fetchPictures = $PDO->prepare("SELECT * FROM pictures WHERE album = :albumId ORDER BY id ASC LIMIT :skip, :max"); $fetchPictures->bindValue(':albumId', (int)$_GET['albumid'], PDO::PARAM_INT); // Cast to int for safety $skip = isset($_GET['skip']) ? (int)trim($_GET['skip']) : 0; // Cleaner skip handling $fetchPictures->bindValue(':skip', $skip, PDO::PARAM_INT); $fetchPictures->bindValue(':max', (int)$max, PDO::PARAM_INT); // Cast to int $fetchPictures->execute() or die(print_r($fetchPictures->errorInfo(), true)); //Improved error handling $pictures = $fetchPictures->fetchAll(PDO::FETCH_ASSOC);
Explanation:
-
Integer Casting: The
(int)
cast ensures the:skip
and:max
values are treated as integers, avoiding quoting issues. We also cast:albumId
for added security. -
Improved
$skip
Handling: The ternary operator provides a more concise way to handle the optional$_GET['skip']
parameter. -
Error Handling: The
print_r()
output is now wrapped intrue
to produce a more readable string for debugging.
This revised code effectively addresses the SQL syntax error by ensuring the LIMIT
clause receives correctly formatted integer values. Remember to always sanitize and validate user inputs to prevent SQL injection vulnerabilities.
The above is the detailed content of How to Fix SQL Syntax Errors When Using `bindValue` with LIMIT Clause in PHP?. For more information, please follow other related articles on the PHP Chinese website!
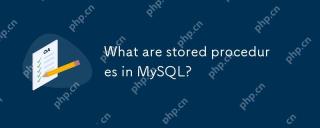
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
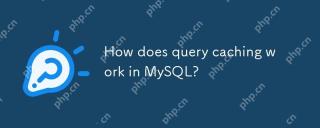
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
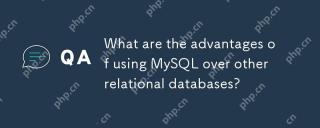
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
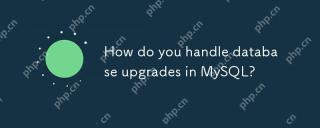
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.
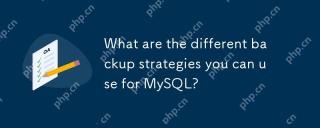
MySQL backup policies include logical backup, physical backup, incremental backup, replication-based backup, and cloud backup. 1. Logical backup uses mysqldump to export database structure and data, which is suitable for small databases and version migrations. 2. Physical backups are fast and comprehensive by copying data files, but require database consistency. 3. Incremental backup uses binary logging to record changes, which is suitable for large databases. 4. Replication-based backup reduces the impact on the production system by backing up from the server. 5. Cloud backups such as AmazonRDS provide automation solutions, but costs and control need to be considered. When selecting a policy, database size, downtime tolerance, recovery time, and recovery point goals should be considered.
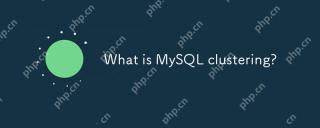
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
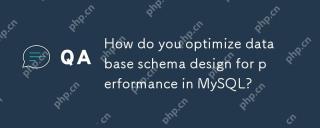
Optimizing database schema design in MySQL can improve performance through the following steps: 1. Index optimization: Create indexes on common query columns, balancing the overhead of query and inserting updates. 2. Table structure optimization: Reduce data redundancy through normalization or anti-normalization and improve access efficiency. 3. Data type selection: Use appropriate data types, such as INT instead of VARCHAR, to reduce storage space. 4. Partitioning and sub-table: For large data volumes, use partitioning and sub-table to disperse data to improve query and maintenance efficiency.
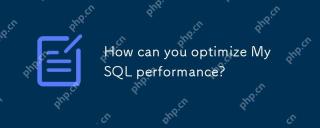
TooptimizeMySQLperformance,followthesesteps:1)Implementproperindexingtospeedupqueries,2)UseEXPLAINtoanalyzeandoptimizequeryperformance,3)Adjustserverconfigurationsettingslikeinnodb_buffer_pool_sizeandmax_connections,4)Usepartitioningforlargetablestoi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
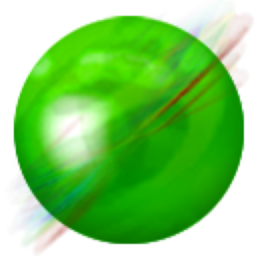
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
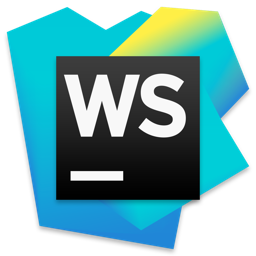
WebStorm Mac version
Useful JavaScript development tools
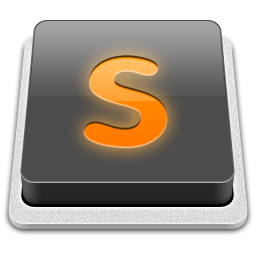
SublimeText3 Mac version
God-level code editing software (SublimeText3)
