JSON (JavaScript Object Notation) has become the universal standard for data exchange, powering APIs, web services, and more. However, dealing with escaped characters in JSON strings can be tricky, especially when dealing with large data sets or complex APIs. JSON decoding simplifies this process by decoding escape characters, making the data easier to read and use.
What is JSON decoding?
JSON decoding is the process of converting escaped characters in a JSON string back to its original, human-readable form. These escape characters are encoded to ensure that special characters (such as quotes or newlines) do not interfere with the JSON syntax. For example, the string ""HellonWorld"" contains escape sequences (" and n), representing double quotes and newlines respectively. JSON decoding converts these sequences back to their expected representation.
Why is JSON decoding important?
Escape characters can hinder readability and usability when processing JSON data. For example, a raw API response or log file may contain many escape sequences that obscure the actual content. Unescaping these characters ensures clean data, making it easier to debug, analyze, and process.
Additionally, decoding is critical for correct data presentation. Failure to accurately decode JSON data can cause display issues or unexpected errors, especially in front-end applications.
Common escape characters in JSON
To better understand JSON decoding, let’s look at some of the most common escape characters in JSON:
- Newline character (n): Represents the newline character in the string.
- Tab (t): Represents tab space.
- Backslash (): Escapes the backslash character itself.
- Double quotes ("): Used to include quotes in a JSON string.
- Unicode Characters (uXXXX): Encodes special characters or symbols in hexadecimal format (for example, u00A9 represents the © symbol).
How JSON decoding works
The decoding process involves parsing the JSON string and converting escape sequences to their original form. For example, a JSON string containing ""HellonWorld"" would be decoded as:
<code>"Hello World"</code>
This decoding ensures that the data appears in its intended format, whether for display, processing or storage.
JSON decoding tools and libraries
There are a variety of tools and programming libraries that help you easily decode JSON data. These include:
- Online Tools: Platforms like JSONLint and FreeFormatter allow users to paste JSON data and decode it with a single click.
- JavaScript: The JSON.parse() method automatically handles decoding when parsing a JSON string.
- Python: The json module and functions like html.unescape() can decode escaped JSON data.
- Java: Libraries such as org.json provide built-in methods for parsing and decoding JSON.
Use JSON decoding in popular programming languages
Here’s how to implement JSON decoding in some of the most widely used programming languages:
- JavaScript
JavaScript’s JSON.parse() automatically decodes characters:
<code>"Hello World"</code>
- Python
Python’s json library makes decoding seamless:
const jsonString = '{"message": "Hello\nWorld"}'; const parsed = JSON.parse(jsonString); console.log(parsed.message); // Output: Hello // World
- Java
Java’s org.json library can efficiently decode JSON data:
import json json_string = '{"message": "Hello\nWorld"}' data = json.loads(json_string) print(data['message']) # Output: Hello # World
Best Practices for JSON Decoding
To ensure efficient handling of escaped characters, follow these best practices:
- Validate JSON input: Always verify that JSON data is well-formed before decoding it.
- Use built-in libraries: Use standard libraries and methods for decoding whenever possible to avoid manual errors.
- Handling edge cases: Be prepared for situations where the escape sequence may be incomplete or invalid.
- Integrate with workflows: Automate JSON validation and decoding in your development pipeline to save time.
Challenges in JSON decoding
While JSON decoding is a simple process, it does come with some challenges:
- Invalid JSON format: Malformed JSON data can cause parsing errors. Always validate your JSON before trying to decode it.
- Encoding Conflict: Mismatched character encodings (e.g., UTF-8 vs. ASCII) can cause problems during decoding.
- Performance bottleneck: Processing large data sets containing a large number of escape sequences may slow down processing.
These challenges can be mitigated by using reliable tools and adhering to best practices.
When to use JSON decoding
JSON decoding is particularly useful when:
- Render user data: Decode the front-end application’s API response.
- Debug Log: Makes JSON log files easier to read.
- Data Transformation: Prepare JSON data for migration or further processing.
Conclusion
JSON decoding is an important tool for developers working with JSON data, ensuring clean and easy-to-read output. Whether you're debugging, processing API responses, or transforming data, knowing how to decode JSON can save time and prevent errors. By leveraging built-in libraries, tools, and best practices, you can seamlessly handle escaped characters and enhance your workflow.
The above is the detailed content of JSON Unescape: Understanding and Using It Effectively. For more information, please follow other related articles on the PHP Chinese website!
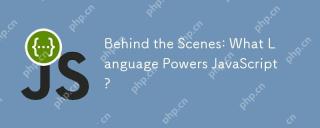
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
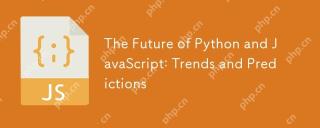
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
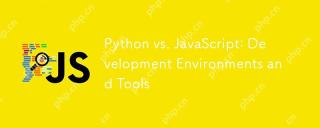
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
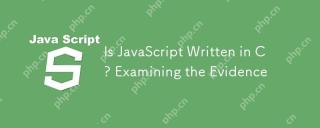
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
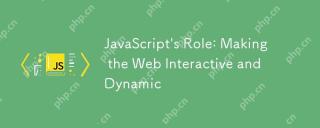
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
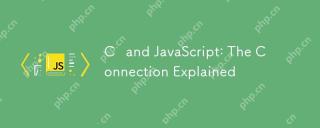
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
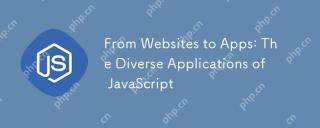
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
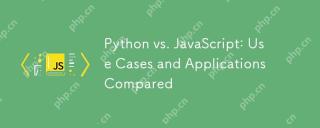
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
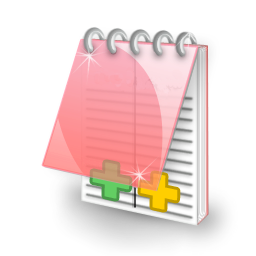
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
