jqGrid: Handling indirect data display
When dealing with tabular data, you often need to display data from different tables. For example, if you have a main table that contains student names and city IDs, and another table that maps city IDs to actual city names, you might want to display city names in the grid instead of city IDs.
Limitations of direct connection method
A common approach is to join these tables based on the city ID field. However, this approach becomes impractical if you are using a class structure that contains a city ID field instead of the actual city name.
Solution: Use lookup function to decode ID
To solve this problem, jqGrid provides the formatter: "select"
function. This function allows you to decode a city ID into the corresponding city name. However, it requires a value map to perform this decoding, and this map needs to be set before jqGrid processes the server response.
The recommended approach is to extend your server response with an additional editoptions.value
attribute data that will be used for the column using the "select" formatter. This extended response can be in the following format:
{ "cityMap": {"11": "Chennai", "12": "Mumbai", "13": "Delhi"}, "rows": [ { "SID": "1", "SNAME": "ABC", "CITY": "11" }, { "SID": "2", "SNAME": "XYZ", "CITY": "12" }, { "SID": "3", "SNAME": "ACX", "CITY": "13" }, { "SID": "4", "SNAME": "KHG", "CITY": "13" }, { "SID": "5", "SNAME": "ADF", "CITY": "12" }, { "SID": "6", "SNAME": "KKR", "CITY": "11" } ] }
In your jqGrid settings you can configure the columns as follows:
colModel: [ {name: "SNAME", width: 250}, {name: "CITY", width: 180, align: "center"} ], beforeProcessing: function (response) { var $self = $(this); $self.jqGrid("setColProp", "CITY", { formatter: "select", edittype: "select", editoptions: { value: $.isPlainObject(response.cityMap) ? response.cityMap : [] } }); }, jsonReader: { id: "SID"}
This method allows you to dynamically set column options based on data received from the server. It also gives you the flexibility to implement more complex scenarios, such as specifying language preferences or handling large numbers of items in a selection element.
The above is the detailed content of How Can I Display Indirect Data (e.g., City Names from IDs) in a jqGrid?. For more information, please follow other related articles on the PHP Chinese website!
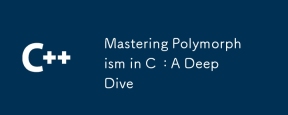
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
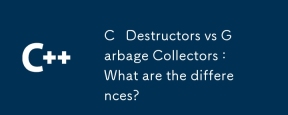
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
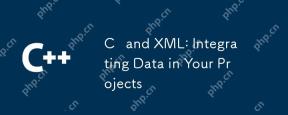
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
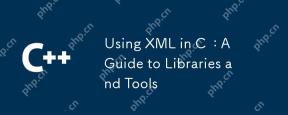
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
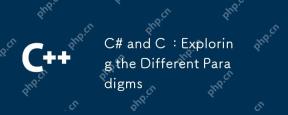
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
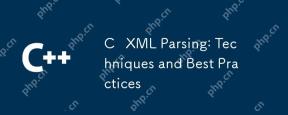
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
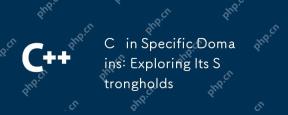
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
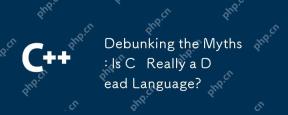
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
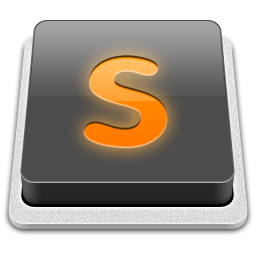
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
