Real-time Clipboard Monitoring with C#
This article demonstrates how to create a C# application that efficiently monitors clipboard changes. The challenge lies in detecting when the clipboard's contents are updated.
Solution:
Utilizing Windows message handling provides a robust solution. This involves:
-
Custom Control: A custom control, inheriting from
Control
(notComponent
), is crucial for obtaining a window handle. This handle is necessary for interacting with the Windows message queue. -
WndProc
Override: TheWndProc
method intercepts Windows messages. We specifically targetWM_DRAWCLIPBOARD
. -
WM_DRAWCLIPBOARD
Handling: This message signals a clipboard update. Within theWndProc
method, whenWM_DRAWCLIPBOARD
is received, theOnClipboardChanged
method is invoked. -
Data Retrieval:
OnClipboardChanged
usesClipboard.GetDataObject()
to retrieve the updated clipboard data. -
Event Trigger: Finally, a custom
ClipboardChanged
event is raised, passing the new data.
Here's a code example showcasing the clipboard monitoring control:
using System; using System.ComponentModel; using System.Runtime.InteropServices; using System.Windows.Forms; using System.Drawing; namespace ClipboardMonitorApp { [DefaultEvent("ClipboardChanged")] public class ClipboardMonitor : Control { private IntPtr nextClipboardViewer; public ClipboardMonitor() { this.BackColor = Color.Red; this.Visible = false; nextClipboardViewer = SetClipboardViewer((int)this.Handle); } public event EventHandler<ClipboardChangedEventArgs> ClipboardChanged; protected override void Dispose(bool disposing) { ChangeClipboardChain(this.Handle, nextClipboardViewer); base.Dispose(disposing); } [DllImport("User32.dll")] private static extern int SetClipboardViewer(int hWndNewViewer); [DllImport("User32.dll", CharSet = CharSet.Auto)] private static extern bool ChangeClipboardChain(IntPtr hWndRemove, IntPtr hWndNewNext); [DllImport("user32.dll", CharSet = CharSet.Auto)] private static extern int SendMessage(IntPtr hwnd, int wMsg, IntPtr wParam, IntPtr lParam); protected override void WndProc(ref Message m) { const int WM_DRAWCLIPBOARD = 0x308; const int WM_CHANGECBCHAIN = 0x030D; switch (m.Msg) { case WM_DRAWCLIPBOARD: OnClipboardChanged(); SendMessage(nextClipboardViewer, m.Msg, m.WParam, m.LParam); break; case WM_CHANGECBCHAIN: if (m.WParam == nextClipboardViewer) nextClipboardViewer = m.LParam; else SendMessage(nextClipboardViewer, m.Msg, m.WParam, m.LParam); break; default: base.WndProc(ref m); break; } } private void OnClipboardChanged() { try { IDataObject data = Clipboard.GetDataObject(); ClipboardChanged?.Invoke(this, new ClipboardChangedEventArgs(data)); } catch (Exception ex) { // Handle exceptions appropriately (e.g., log the error) MessageBox.Show(ex.ToString()); } } } public class ClipboardChangedEventArgs : EventArgs { public readonly IDataObject DataObject; public ClipboardChangedEventArgs(IDataObject dataObject) { DataObject = dataObject; } } }
This refined code provides a more robust and error-handled solution for monitoring clipboard changes in your C# applications. Remember to handle potential exceptions appropriately in a production environment.
The above is the detailed content of How Can I Detect Clipboard Changes in C#?. For more information, please follow other related articles on the PHP Chinese website!
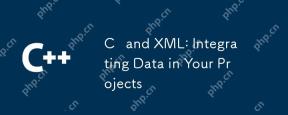
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
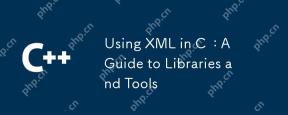
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
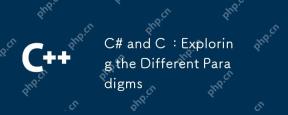
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
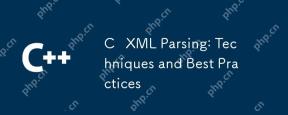
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
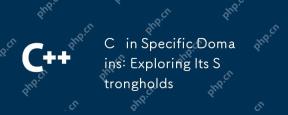
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
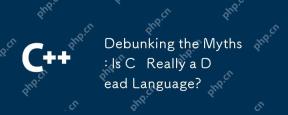
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
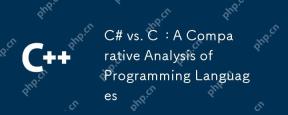
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
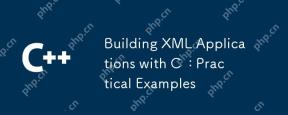
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
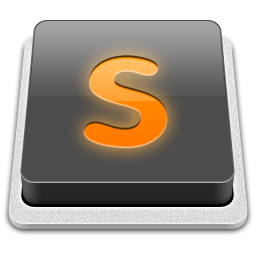
SublimeText3 Mac version
God-level code editing software (SublimeText3)
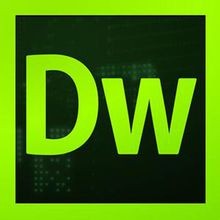
Dreamweaver CS6
Visual web development tools
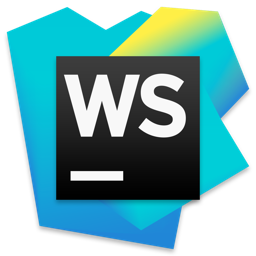
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
