Introduction
TypeScript is rapidly gaining popularity among JavaScript developers seeking robust, scalable application solutions. Its strengths lie in features like strong typing, advanced tooling, and improved error handling, simplifying complex app development. A core concept is understanding data types, enabling precise variable definition and preventing common runtime errors.
This guide delves into TypeScript's essential data types, explaining their use in writing cleaner, more maintainable code. Whether you're a novice or an experienced developer, this resource provides a solid foundation.
Why Choose TypeScript?
Before exploring data types, let's examine TypeScript's advantages. It extends JavaScript with optional static typing, enabling early error detection. Powerful tooling, such as autocompletion and type-checking, boosts developer productivity and code quality. TypeScript's compiler proactively identifies errors often missed by JavaScript, saving time and frustration. This makes it suitable for projects of all sizes.
Essential TypeScript Data Types
Mastering TypeScript data types is crucial for writing effective, bug-free code. Let's examine the most common types:
1. Number: Precise Numeric Handling
TypeScript's number
type handles both integers and floating-point numbers. Unlike JavaScript, it doesn't differentiate between int
and float
, simplifying numeric data manipulation.
let age: number = 30; let price: number = 99.99;
2. String: Efficient Text Manipulation
The string
type stores text data. Define strings using single, double quotes, or backticks (for template literals and interpolation).
let username: string = "Alice"; let greeting: string = `Hello, ${username}!`;
String interpolation simplifies dynamic text handling.
3. Boolean: Representing True/False Values
The boolean
type represents logical values (true
or false
), essential for conditional statements and application flow control.
let isAuthenticated: boolean = true; let isActive: boolean = false;
4. Array: Typed Data Collections
TypeScript arrays are strongly typed, specifying the data type they hold. This ensures consistent data type usage throughout your application.
let numbers: number[] = [1, 2, 3, 4, 5]; let names: Array<string> = ["Alice", "Bob", "Charlie"];
5. Tuple: Fixed-Size, Heterogeneous Arrays
Tuples resemble arrays but hold a fixed number of elements, each with a potentially different type. They're useful for storing data with mixed types in a predefined structure.
let person: [string, number] = ["Alice", 30];
6. Enum: Defining Named Constants
Enums define sets of named constants. Values are numeric by default, but custom values can be assigned.
let age: number = 30; let price: number = 99.99;
Enums enhance code readability and maintainability.
7. Any: A Wildcard Type (Use Sparingly)
The any
type bypasses type checking. While offering flexibility, overuse undermines TypeScript's type safety.
let username: string = "Alice"; let greeting: string = `Hello, ${username}!`;
8. Void: For Functions Without Return Values
The void
type is for functions without return values, indicating their focus on side effects rather than result computation.
let isAuthenticated: boolean = true; let isActive: boolean = false;
9. Null & Undefined: Representing Absence of Values
null
and undefined
are distinct types. null
indicates an intentional absence of a value, while undefined
signifies a declared but uninitialized variable.
let numbers: number[] = [1, 2, 3, 4, 5]; let names: Array<string> = ["Alice", "Bob", "Charlie"];
Both are subtypes of any
but used differently to represent "empty" or "missing" data.
10. Never: For Unreachable Code
The never
type applies to functions that never return a value (due to errors or infinite loops).
let person: [string, number] = ["Alice", 30];
11. Object: Defining Non-Primitive Types
The object
type defines non-primitive data structures (functions, arrays, objects). It's a base type for any non-primitive value.
enum Direction { Up = 1, Down, Left, Right } let move: Direction = Direction.Up;
TypeScript Type Assertions: Overriding Type Inference
Type assertions explicitly tell the compiler the expected type when automatic inference fails.
let data: any = 42; data = "Now I am a string"; data = [1, 2, 3];
Or using angle bracket syntax:
function logMessage(message: string): void { console.log(message); }
Conclusion: Why Understanding Data Types Matters
TypeScript offers more than just typed JavaScript; it's a powerful tool for writing safer, more efficient, and maintainable code. Utilizing its data types prevents bugs, improves collaboration, and ensures scalability. This guide covered primitive and advanced types; understanding them unlocks TypeScript's full potential for building robust applications.
Key Takeaways:
- TypeScript enhances JavaScript with type safety.
- Master basic data types (
number
,string
,boolean
,any
). - Leverage advanced types (
tuple
,enum
,never
) for complex data structures. - Use type assertions when type inference is insufficient.
FAQs: (Similar to the original, rephrased for better flow)
- What is TypeScript? A statically-typed superset of JavaScript adding optional type annotations and features to improve code quality and developer productivity.
- Why use TypeScript? It enhances JavaScript with static typing, interfaces, and classes, enabling early error detection, improved refactoring, and better tooling.
- How does TypeScript handle arrays and tuples? Arrays are strongly typed, specifying element types. Tuples are fixed-size arrays with potentially different types per element.
- Can TypeScript be used with React? Absolutely! It integrates seamlessly with React, providing type safety for components and related elements.
- Can TypeScript be converted to JavaScript? Yes, the TypeScript compiler converts it to plain JavaScript, ensuring compatibility with any JavaScript environment.
Related Blog Posts: (Same as original)
- Understanding TypeScript Features, Benefits & Use Cases
- Converting TypeScript to JavaScript: A Comprehensive Guide with Real-World Examples
- Learn How to Use TypeScript with React: Benefits and Best Practices
The above is the detailed content of Mastering TypeScript Data Types: A Beginners Guide for �. For more information, please follow other related articles on the PHP Chinese website!
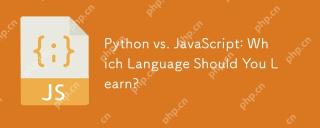
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
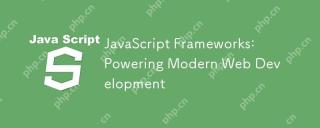
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
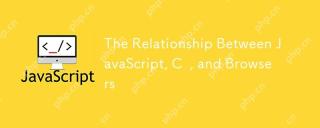
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
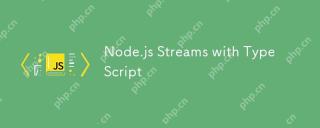
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
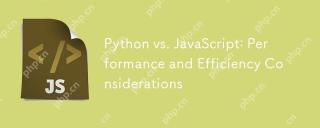
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
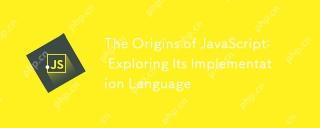
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
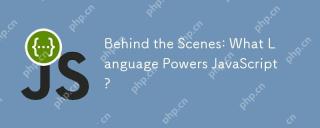
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
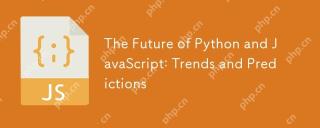
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
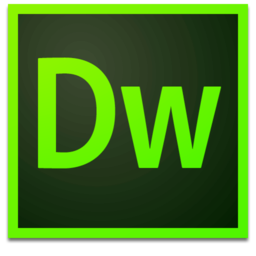
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
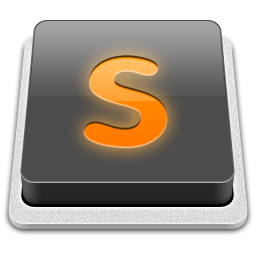
SublimeText3 Mac version
God-level code editing software (SublimeText3)
