Modeling Many-to-Many Relationships in PostgreSQL Databases
PostgreSQL, like other relational database systems, allows for complex relationships between database tables. A common scenario is the many-to-many relationship, where multiple records in one table can be associated with multiple records in another.
Establishing the Table Structure
Implementing a many-to-many relationship in PostgreSQL typically requires a third, intermediary table. This "junction table" or "bridge table" connects the two original tables. It contains foreign keys referencing the primary keys of both tables, forming a composite primary key (or a unique constraint).
Let's illustrate with an example:
CREATE TABLE product ( product_id SERIAL PRIMARY KEY, product_name TEXT NOT NULL, price NUMERIC NOT NULL DEFAULT 0 ); CREATE TABLE invoice ( invoice_id SERIAL PRIMARY KEY, invoice_number TEXT NOT NULL, invoice_date DATE NOT NULL DEFAULT CURRENT_DATE ); CREATE TABLE invoice_product ( invoice_id INTEGER REFERENCES invoice (invoice_id) ON UPDATE CASCADE ON DELETE CASCADE, product_id INTEGER REFERENCES product (product_id) ON UPDATE CASCADE, quantity NUMERIC NOT NULL DEFAULT 1, CONSTRAINT invoice_product_pkey PRIMARY KEY (invoice_id, product_id) );
The Junction Table and Foreign Key Constraints
The invoice_product
table acts as the junction table, linking invoice
and product
. invoice_id
and product_id
are foreign keys, ensuring referential integrity. The ON UPDATE CASCADE
and ON DELETE CASCADE
clauses maintain data consistency when records are modified or deleted in the main tables.
Managing Relationships
When inserting or deleting rows from the invoice
or product
tables, the cascading actions on the foreign keys automatically update or remove related entries in the invoice_product
table, simplifying data management.
Retrieving Related Data
To query data across this many-to-many relationship, use JOIN
operations. For example, to find all products associated with a specific invoice:
SELECT p.product_name, ip.quantity FROM invoice i JOIN invoice_product ip ON i.invoice_id = ip.invoice_id JOIN product p ON ip.product_id = p.product_id WHERE i.invoice_id = 123;
In summary, effectively managing many-to-many relationships in PostgreSQL involves creating a junction table with appropriate foreign key constraints. This approach facilitates clear data modeling and efficient data retrieval.
The above is the detailed content of How Do I Implement Many-to-Many Relationships in PostgreSQL?. For more information, please follow other related articles on the PHP Chinese website!
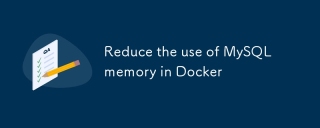
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
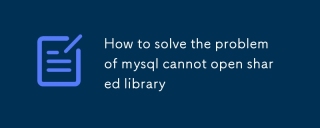
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
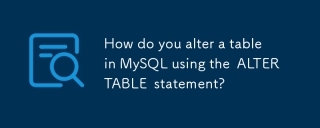
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
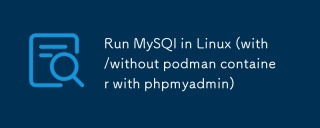
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
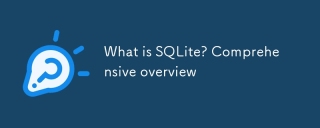
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
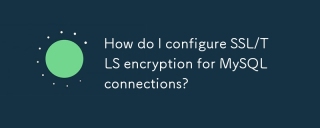
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
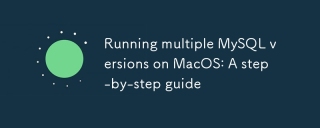
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
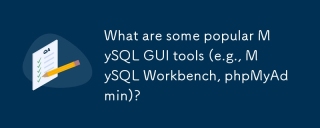
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
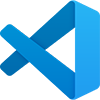
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
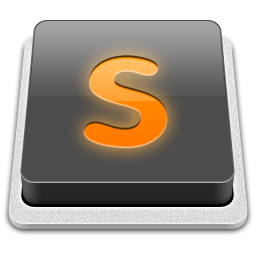
SublimeText3 Mac version
God-level code editing software (SublimeText3)
