I recently tackled a Frontend Mentor challenge: crafting a responsive "Coming Soon" landing page for a fashion retailer. The brief called for an email subscription form, a captivating background image, and seamless responsiveness across desktop and mobile. This post details my approach, key decisions, and problem-solving strategies.
Phase 1: Project Deconstruction
Before coding, I meticulously analyzed the project requirements:
- A hero section showcasing the logo and "Coming Soon" message.
- An email subscription form for user sign-ups.
- A responsive layout with a background image dynamically adapting to screen size.
- Basic email validation to ensure accurate email input.
Phase 2: HTML Structure
I prioritized a clean, semantic HTML structure with minimal divs. The layout comprised two key sections:
- Details Section: Housing the logo, headline, description, and email input form.
- Image Section: Displaying the background image.
My HTML structure:
<div class="coming-soon-container"> <!-- Details Section --> <div class="details"> <!-- Logo, Heading, Description, Form --> </div> <!-- Image Section --> <div class="image-container"> <img src="/static/imghwm/default1.png" data-src="..." class="lazy" alt="Coming Soon Image"> </div> </div>
Phase 3: CSS Styling and Responsiveness
Flexbox was instrumental in achieving a visually appealing and responsive layout. It streamlined the arrangement of elements both horizontally (desktop) and vertically (mobile).
Initially, the .coming-soon-container
used display: flex
for a side-by-side desktop layout. A media query (@media (max-width: 768px)
) switched the flex-direction
to column-reverse
for mobile, placing the image below the details.
Flexbox CSS:
.coming-soon-container { display: flex; justify-content: space-between; align-items: center; } @media (max-width: 768px) { .coming-soon-container { flex-direction: column-reverse; } }
Phase 4: Email Input and Arrow Icon Design
The email form was designed for a clean, modern aesthetic. The input field and submit button (an arrow icon) were styled for visual appeal.
CSS for Input and Icon:
.input-container { position: relative; width: 385px; } /* ... (input and span styles) ... */
Phase 5: Responsive Hero Image
The object-fit
property ensured the hero image scaled responsively without distortion.
CSS for Image Container:
.image-container { width: 40%; height: 100vh; overflow: hidden; } .image-container img { width: 100%; height: 100%; object-fit: cover; object-position: top; }
Phase 6: JavaScript Email Validation
Client-side email validation was implemented using JavaScript to verify email format. A regular expression checked the input against a standard email pattern.
JavaScript Validation Function:
function validateEmail() { // ... (validation logic) ... }
Conclusion
This "Coming Soon" page project honed my responsive design and form validation skills. Utilizing Flexbox for layout, custom styling, and mobile-first principles resulted in a clean and functional design. I highly recommend Frontend Mentor's challenges for sharpening frontend development skills. The complete code is available on GitHub: GitHub Link
The above is the detailed content of Building a Responsive 'Coming Soon' Page. For more information, please follow other related articles on the PHP Chinese website!
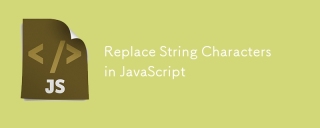
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
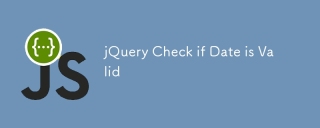
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
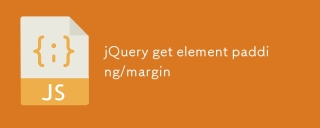
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
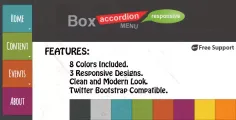
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
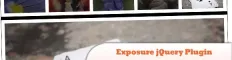
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
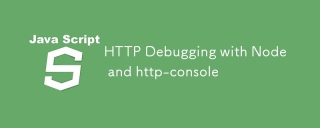
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
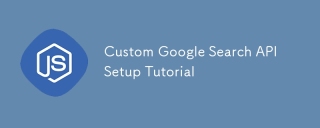
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
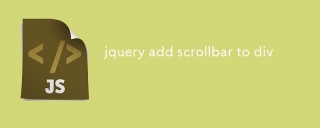
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
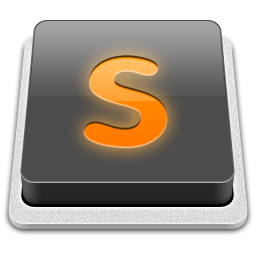
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
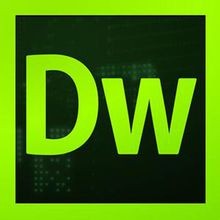
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
