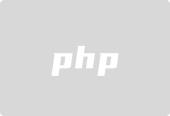
Comparison of Join and GroupJoin in LINQ to Entities
Understanding GroupJoin
GroupJoin, like Join, is a LINQ method for combining multiple data sources. However, GroupJoin produces results with a different structure than Join.
Join:
- Perform an inner join and only generate rows where there are matches in both joined tables.
- Result: A merged table containing columns from both tables.
GroupJoin:
- Perform an outer join to match rows in the first table with rows in the second table.
- Result: A collection of groups, where each group contains a row from the first table and its matching row from the second table (or an empty list if no match exists).
Grammar
Query syntax:
Join:
<code>from p in Parent
join c in Child on p.Id equals c.Id
select new { p.Value, c.ChildValue }</code>
GroupJoin:
<code>from p in Parent
join c in Child on p.Id equals c.Id into g
select new { Parent = p, Children = g }</code>
Method syntax (less commonly used):
Join:
<code>Parent.Join(Child, p => p.Id, c => c.Id, (p, c) => new { p.Value, c.ChildValue })</code>
GroupJoin:
<code>Parent.GroupJoin(Child, p => p.Id, c => c.Id, (p, c) => new { Parent = p, Children = c })</code>
Use cases
1. Generate flat outer connection:
- GroupJoin can be used to create a flattened outer join that contains every row in the first table, even if there are no matching rows in the second table. This is achieved by flattening the group:
<code>from p in Parent
join c in Child on p.Id equals c.Id into g
from c in g.DefaultIfEmpty()
select new { Parent = p.Value, Child = c?.ChildValue }</code>
2. Keep order:
- Join can be used to filter a list while maintaining the order of elements. By using an external sequence as the first list, you can maintain the order of elements from the second list:
<code>from id in ids
join p in parents on id equals p.Id
select p</code>
The above is the detailed content of What's the Difference Between LINQ's Join and GroupJoin Methods?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn