


JavaScript data type:
In JavaScript, data types define the types of values that a variable can hold. The type determines what operations can be performed on the value. JavaScript has both primitive data types (simple values) and reference data types (complex objects).
1. Original data type:
These are the most basic data types in JavaScript, they hold a single value. They are immutable, which means that once their values are set, they cannot be changed.
a) Number:
represents integers and floating point numbers.
let x = 42;
b) String:
represents a series of characters.
let name = "Alice";
c) Boolean:
means true or false.
let isActive = true;
d) Null:
means intentionally not having any object value.
let person = null;
e) Undefined:
Indicates a variable that has been declared but not assigned a value.
let value;
f) Symbol:
A unique identifier.
const uniqueID = Symbol();
g) BigInt:
is used to represent large integers.
let bigNum = 123n;
2. Reference data type:
These data types are more complex, storing collections of values or more structured data. They are mutable, which means their contents can change even if the reference remains the same.
a) Object:
A collection of key-value pairs.
let person = {name: "John"};
b) Array:
A sorted collection of values.
let arr = [1, 2, 3];
c) Function:
Code block that performs tasks.
function greet() {}
JavaScript variables:
In JavaScript, variables are named containers for storing data that can be referenced and manipulated throughout your code.
Variables allow you to store values such as numbers, strings, objects, and functions.
1. Declare variables:
In JavaScript, variables are declared using one of three keywords:
var (old method, less used now) let (block scope, introduced in ES6) const (block scope, for constants, also introduced in ES6)
a) var (old method):
The var keyword is traditionally used to declare variables in JavaScript.
var name = "Alice";
var age = 25;
b) let (modern method):
Block scope means that a variable can only be accessed within the block in which it is defined (for example, within an if statement or loop).
let name = "Bob";
let age = 30;
c) const (constant variable):
The const keyword is used to declare variables that should not be reassigned after initial assignment.
const country = "USA";
Redeclare variables:
Use var: You can redeclare variables within the same scope and JavaScript will not throw an error.
var name = "Alice"; var name = "Bob"; // 没有错误 console.log(name); // 输出:Bob
Use let and const: You cannot redeclare a variable within the same scope. Doing so will cause a SyntaxError.
let name = "Alice"; let name = "Bob"; // SyntaxError: Identifier 'name' has already been declared
const country = "USA"; const country = "Canada"; // SyntaxError: Identifier 'country' has already been declared
JavaScript operators:
In JavaScript, operators are symbols or keywords that perform operations on operands.
1. Arithmetic operators:
These operators are used to perform mathematical operations on numerical values.
<code>+ 加法 5 + 3 → 8 - 减法 5 - 3 → 2 * 乘法 5 * 3 → 15 / 除法 5 / 3 → 1.6667 % 取模(除法的余数) 5 % 3 → 2 ** 幂运算(乘方) 2 ** 3 → 8 ++ 递增(加 1) let x = 5; x++ → 6 -- 递减(减 1) let x = 5; x-- → 4</code>
2. Assignment operator:
These operators are used to assign values to variables.
<code>= 简单赋值 let x = 10; += 加法赋值 x += 5; → x = x + 5; -= 减法赋值 x -= 5; → x = x - 5; *= 乘法赋值 x *= 5; → x = x * 5; /= 除法赋值 x /= 5; → x = x / 5; %= 取模赋值 x %= 5; → x = x % 5; **= 幂赋值 x **= 2; → x = x ** 2;</code>
3. Comparison operators:
These operators compare two values and return a Boolean value (true or false).
<code>== 等于 5 == "5" → true === 全等 5 === "5" → false != 不等于 5 != "5" → false !== 不全等 5 !== "5" → true > 大于 5 > 3 → true >= 大于等于 5 >= 3 → true </code>
4. Logical operators:
These operators are used to perform logical operations, usually using Boolean values.
<code>&& 逻辑与 true && false → false || 逻辑或 true || false → true ! 逻辑非 !true → false</code>
JavaScript conditional statement:
In JavaScript, conditional statements are used to perform different actions based on whether a specific condition is true or false.
1. if statement
The if statement is the most basic conditional statement. If the specified condition evaluates to true, the code block is executed.
Grammar:
if (condition) { // 如果条件为真,则执行的代码块 }
2. else statement
Theelse statement follows the if statement and defines a block of code that is executed if the condition in the if statement is false.
Grammar:
if (condition) { // 如果条件为真,则执行的代码块 } else { // 如果条件为假,则执行的代码块 }
3. else if statement
The else if statement allows you to test multiple conditions. Use it when you need to check multiple conditions.
Grammar:
if (condition1) { // 如果 condition1 为真,则执行的代码块 } else if (condition2) { // 如果 condition2 为真,则执行的代码块 } else { // 如果 condition1 和 condition2 都为假,则执行的代码块 }
4. Nested if statements
You can nest if statements within other if statements to create more complex conditions.
5. switch statement
The switch statement is a more efficient way to test a value with multiple conditions, especially when you have many different possible outcomes.
Grammar:
switch (expression) { case value1: // 如果 expression === value1,则运行的代码 break; case value2: // 如果 expression === value2,则运行的代码 break; default: // 如果没有 case 匹配,则运行的代码 }
JavaScript loop:
In JavaScript, a loop is used to repeatedly execute a block of code as long as a specific condition is met. This is useful when you want to perform repetitive tasks, such as iterating over an array or executing a block of code multiple times.
1. for loop
The for loop is the most common loop in JavaScript. This is typically used when you know how many times you want to iterate a block of code.
Grammar:
for (initialization; condition; increment/decrement) { // 每次迭代中要执行的代码 }
2. while loop
The while loop executes a block of code as long as the specified condition evaluates to true. The condition is checked before each iteration and the loop stops once the condition becomes false.
Grammar:
while (condition) { // 只要条件为真就执行的代码 }
The above is the detailed content of Day Datatypes, Variables,Operators, Conditional Statements,Looping in Javascript. For more information, please follow other related articles on the PHP Chinese website!
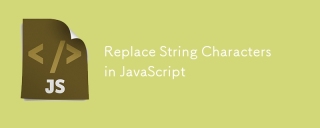
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
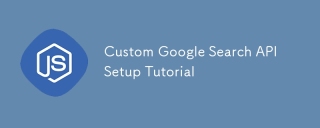
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
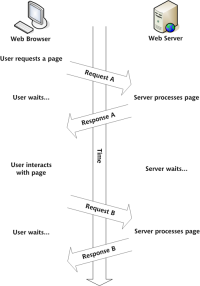
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
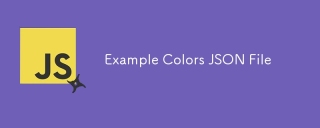
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
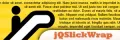
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
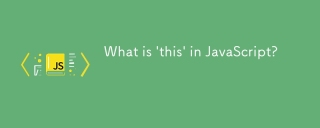
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
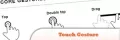
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
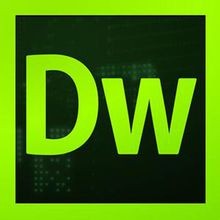
Dreamweaver CS6
Visual web development tools
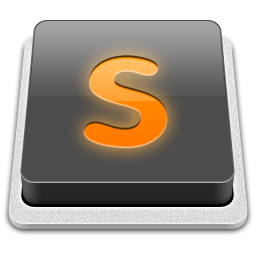
SublimeText3 Mac version
God-level code editing software (SublimeText3)
