Understanding Regular Expression Syntax in C#
When working with regular expressions in C#, you might encounter issues if you try to use delimiters like forward slashes (/
), which are common in languages such as PHP or JavaScript. Let's examine why this doesn't work in C# and how to correctly implement regular expressions.
The problem arises from attempting to use a regular expression like this (incorrect):
"name = dr-det-fb.ydp.eu/ebook/trunk/annotations/ctrl.php/api1751-4060-1193-0487\nname = Regex.Replace(name, @"/\W/g", "")"
This code attempts to remove "/", "-", and "." using delimiters. However, C# handles regular expressions differently. Delimiters aren't required and, in fact, will cause errors.
The Correct Approach in C#
To define a regular expression in C#, use the @
symbol to create a verbatim string literal. This prevents C# from interpreting escape sequences within the string. You also need to correctly escape special characters within the regex itself.
The corrected code to remove non-alphanumeric characters (W
) would be:
name = Regex.Replace(name, @"\W", "");
This single line of code effectively replaces all non-alphanumeric characters in the name
string with an empty string, achieving the desired result without the need for delimiters. Remember that W
is the regex character class matching any non-alphanumeric character.
The above is the detailed content of Why Aren't My Regular Expression Delimiters Working in C#?. For more information, please follow other related articles on the PHP Chinese website!
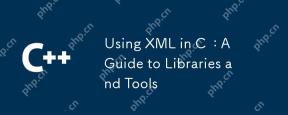
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
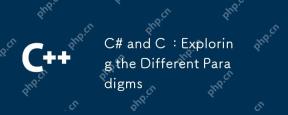
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
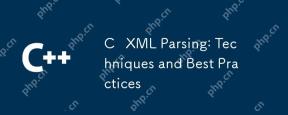
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
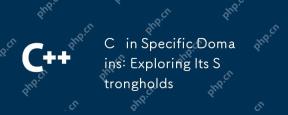
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
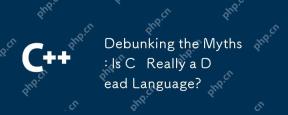
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
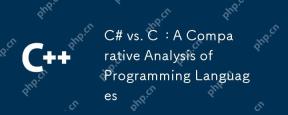
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
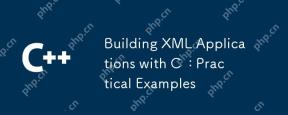
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
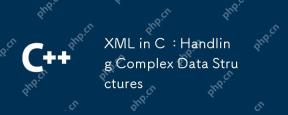
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
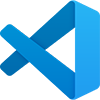
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
