


It is crucial to build a well-structured Flask RESTful API that is readable, maintainable, extensible, and easy for other developers to use. This article will introduce some best practices to help developers improve their API design and provide a complete guide to building a Flask REST API.
Project Structure
A typical and efficient Flask REST API project structure is as follows:
project/ │ ├── app/ │ ├── init.py │ ├── config.py │ ├── models/ │ │ ├── init.py │ │ └── user.py │ ├── routes/ │ │ ├── init.py │ │ └── user_routes.py │ ├── schemas/ │ │ ├── init.py │ │ └── user_schema.py │ ├── services/ │ │ ├── init.py │ │ └── user_service.py │ └── tests/ │ ├── init.py │ └── test_user.py ├── run.py └── requirements.txt
Key components:
- app/init.py: Initialize the Flask application and register the blueprint.
- app/config.py: Contains the application’s configuration settings.
- models/: stores database models.
- routes/: Defines API endpoints.
- schemas/: manages data serialization and validation.
- services/: Contains business logic and interacts with the model.
- tests/: stores the unit tests of the application.
- Using Blueprints: Flask’s blueprint feature allows you to organize your application into different components. Each blueprint can handle its routes, models, and services, making it easier to manage large applications. For example, you can create a user blueprint that specifically handles user-related functionality.
Blueprint initialization example:
# app/routes/user_routes.py from flask import Blueprint user_bp = Blueprint('user', __name__) @user_bp.route('/users', methods=['GET']) def get_users(): # 获取用户逻辑 pass @user_bp.route('/users', methods=['POST']) def create_user(): # 创建新用户逻辑 pass
Implement CRUD operations
Most Flask REST APIs include CRUD operations. Here's how to define these actions in your routes:
CRUD operation example:
# app/routes/user_routes.py @user_bp.route('/users/<user_id>', methods=['GET']) def get_user(user_id): # 根据 ID 获取用户逻辑 pass @user_bp.route('/users/<user_id>', methods=['PUT']) def update_user(user_id): # 更新现有用户逻辑 pass @user_bp.route('/users/<user_id>', methods=['DELETE']) def delete_user(user_id): # 根据 ID 删除用户逻辑 pass
Use Marshmallow for data validation
Data validation and serialization can be greatly simplified using libraries like Marshmallow. Create a schema that represents a data structure:
Pattern definition example:
# app/schemas/user_schema.py from marshmallow import Schema, fields class UserSchema(Schema): id = fields.Int(required=True) username = fields.Str(required=True) email = fields.Email(required=True)
API Test
Testing is critical to ensuring your API works correctly. Unit tests can be written using tools such as pytest.
Test case example:
# app/tests/test_user.py def test_get_users(client): response = client.get('/users') assert response.status_code == 200
Conclusion
You can follow this structured approach to develop a robust and easy-to-maintain Flask REST API. Using blueprints, efficient CRUD operations, data validation through schemas, and documentation with Swagger are best practices that can help you jumpstart your development efforts.
The above is the detailed content of How To Structure a Large Flask Application-Best Practices for 5. For more information, please follow other related articles on the PHP Chinese website!
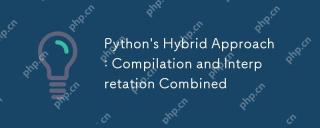
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
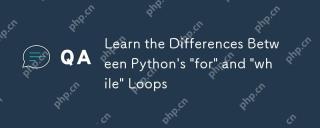
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
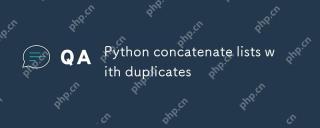
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.
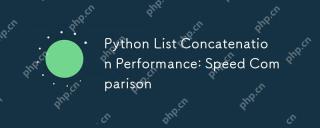
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
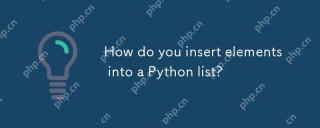
ToinsertelementsintoaPythonlist,useappend()toaddtotheend,insert()foraspecificposition,andextend()formultipleelements.1)Useappend()foraddingsingleitemstotheend.2)Useinsert()toaddataspecificindex,thoughit'sslowerforlargelists.3)Useextend()toaddmultiple
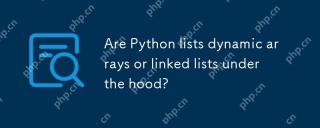
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
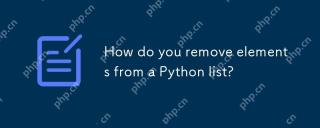
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
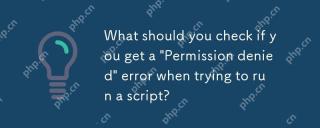
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
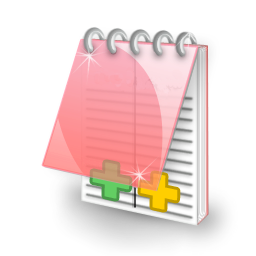
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
