


MVC form cannot submit object list
In an MVC application, users encountered a problem: a form containing a list of objects could not submit data to the controller, resulting in an empty list being passed. The form uses a loop and a partial view to render the items, but upon submission the controller receives an empty enum.
Problem Analysis
To understand why the data is not submitted correctly, let's examine the provided code:
Parent view:
@foreach (var planVM in Model) { @Html.Partial("_partialView", planVM) }
Partial View (_partialView):
@Html.HiddenFor(p => p.PlanID) @Html.HiddenFor(p => p.CurrentPlan) @Html.CheckBoxFor(p => p.ShouldCompare)
PlanCompareViewModel class:
public class PlansCompareViewModel { public int PlanID { get; set; } public Plan CurrentPlan { get; set; } public bool ShouldCompare { get; set; } }
Solution
The root cause of the problem lies in the way the form elements are named. Without an index, the model binder cannot distinguish between the elements of the list. To fix this, we need to assign the correct index to the name of the form element:
Improved partial view (_partialView):
@for (int i = 0; i < Model.Count(); i++) { @Html.HiddenFor(p => p[i].PlanID) @Html.HiddenFor(p => p[i].CurrentPlan) @Html.CheckBoxFor(p => p[i].ShouldCompare) }
This modification allows the model binder to associate individual data elements with the correct list items.
Improve form generation using editor templates
In order to be more concise and efficient, it is recommended to use the editor template. Part of the view can be replaced with the following content by creating PlanCompareViewModel.cshtml in the EditorTemplates folder:
<div> @Html.HiddenFor(p => p.PlanID) @Html.HiddenFor(p => p.CurrentPlan) @Html.CheckBoxFor(p => p.ShouldCompare) </div>
Finally, the parent view can be simplified to:
@Html.EditorForModel()
The editor template automatically handles indexing to ensure form elements are named correctly for model binding.
The above is the detailed content of Why is my MVC form failing to post a list of objects, resulting in a null list in the controller?. For more information, please follow other related articles on the PHP Chinese website!
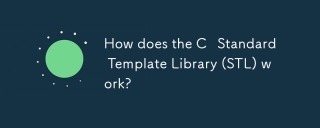
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
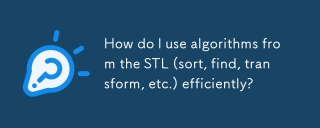
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
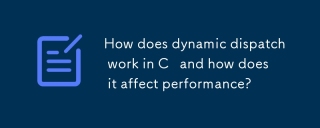
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
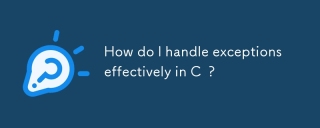
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
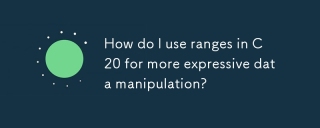
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
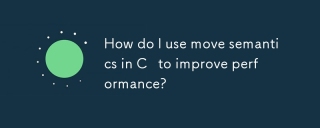
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
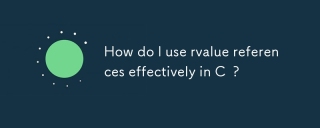
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
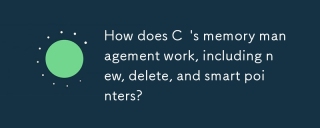
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
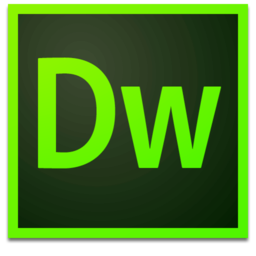
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
