Fast bitmap processing in C#
When working with large bitmaps, accessing and performing operations on a pixel-by-pixel basis may impact performance. C#'s built-in Bitmap.GetPixel()
and Bitmap.SetPixel()
methods are convenient but slow. This article explores alternative ways to quickly convert a bitmap to a byte array and back to a bitmap, allowing for efficient pixel operations.
Convert bitmap to byte array
- LockBits method: This method allows direct access to the bitmap's internal data buffer. By using unsafe code, you can access the raw pixel data as pointers, allowing for fast operations. However, this approach requires careful memory management.
-
Marshaling: Marshaling involves copying data between managed and unmanaged memory. Using the
Marshal.Copy()
method you can copy pixel data from a bitmap buffer into a byte array. Although marshaling does not require unsafe code, it may be slightly slower than the LockBits method.
Convert byte array to bitmap
- LockBits method: After modifying the pixel data in the byte array, it can be copied back to the bitmap through the LockBits method. This is similar to converting a bitmap into a byte array.
-
Marshaling: You can use the
Marshal.Copy()
method to copy modified pixel data from a byte array back to a bitmap buffer.
Performance comparison
- LockBits: faster, requires unsafe code
- Marshaling: Slower, but no need for unsafe code
LockBits method example
using System; using System.Drawing; using System.Runtime.InteropServices; public unsafe class FastBitmap { public static Image ThresholdUA(float thresh) { Bitmap b = new Bitmap(_image); BitmapData bData = b.LockBits(new Rectangle(0, 0, _image.Width, _image.Height), ImageLockMode.ReadWrite, b.PixelFormat); byte bitsPerPixel = GetBitsPerPixel(bData.PixelFormat); byte* scan0 = (byte*)bData.Scan0.ToPointer(); for (int i = 0; i < ... }
Example of marshaling method
using System; using System.Drawing; using System.Runtime.InteropServices; public class FastBitmap { public static Image ThresholdMA(float thresh) { Bitmap b = new Bitmap(_image); BitmapData bData = b.LockBits(new Rectangle(0, 0, _image.Width, _image.Height), ImageLockMode.ReadWrite, b.PixelFormat); byte bitsPerPixel = GetBitsPerPixel(bData.PixelFormat); int size = bData.Stride * bData.Height; byte[] data = new byte[size]; Marshal.Copy(bData.Scan0, data, 0, size); for (int i = 0; i < ... }
The above is the detailed content of How Can I Efficiently Manipulate Bitmaps in C#?. For more information, please follow other related articles on the PHP Chinese website!
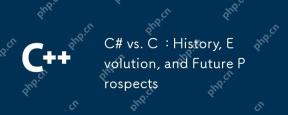
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
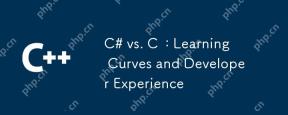
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
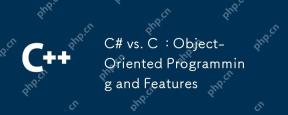
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
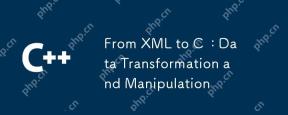
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
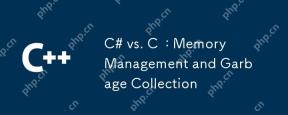
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
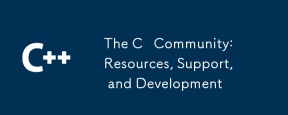
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
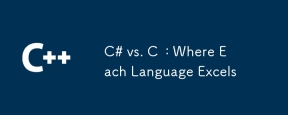
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
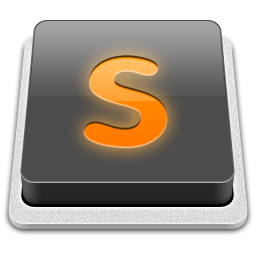
SublimeText3 Mac version
God-level code editing software (SublimeText3)
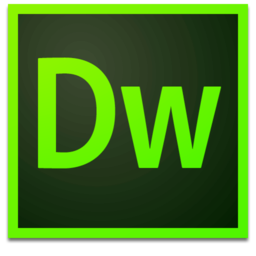
Dreamweaver Mac version
Visual web development tools
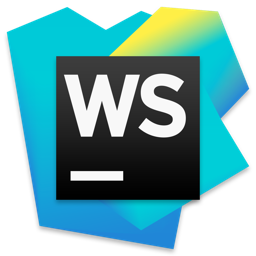
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment