


How Can We Effectively Manage Dependencies in a Factory Method Pattern with DI/IoC?
Factory Method Pattern: Addressing Dependency Management Challenges with DI/IoC
The Factory Method pattern, enhanced by Dependency Injection (DI) and Inversion of Control (IoC), offers an elegant abstraction for object creation. However, managing numerous dependencies within the factory's constructor can become problematic. This article explores solutions to this common challenge.
The Problem: Overly Complex Factory Constructors
A CarFactory
with a constructor requiring many dependencies exemplifies this issue. This design contradicts the Factory Method's goal of encapsulating creation logic and isolating it from dependency details. Manually injecting each dependency becomes unwieldy as the variety of car types grows.
Solutions: Refined Approaches
Two key approaches offer improved dependency management:
Approach 1: Container Injection
This simplifies the factory constructor by injecting a service container responsible for resolving dependencies dynamically. This reduces the factory's direct dependencies:
public class CarFactory { private readonly IContainer _container; public CarFactory(IContainer container) { _container = container; } public ICar CreateCar(Type type) { // Resolve dependencies via the container switch (type) { case Type a: return _container.Resolve<ICar1>(); case Type b: return _container.Resolve<ICar2>(); default: throw new ArgumentException("Unsupported car type."); } } }
While effective, this approach introduces a reliance on a service locator.
Approach 2: The Strategy Pattern – A More Elegant Solution
The Strategy pattern offers a superior solution by decoupling the factory's interface from its implementation. This enables registering multiple factories and dynamically selecting them based on the object type:
Interfaces:
public interface ICarFactory { ICar CreateCar(); bool AppliesTo(Type type); } public interface ICarStrategy { ICar CreateCar(Type type); }
Concrete Factories (Examples):
public class Car1Factory : ICarFactory { // Dependencies injected into the factory public Car1Factory(IDep1 dep1, IDep2 dep2, IDep3 dep3) { ... } public ICar CreateCar() { ... } public bool AppliesTo(Type type) { ... } } public class Car2Factory : ICarFactory { ... }
The Strategy:
public class CarStrategy : ICarStrategy { private readonly ICarFactory[] _carFactories; public CarStrategy(ICarFactory[] carFactories) { _carFactories = carFactories; } public ICar CreateCar(Type type) { var factory = _carFactories.FirstOrDefault(f => f.AppliesTo(type)); if (factory == null) throw new InvalidOperationException("No factory registered for type " + type); return factory.CreateCar(); } }
Usage:
var strategy = new CarStrategy(new ICarFactory[] { new Car1Factory(dep1, dep2, dep3), new Car2Factory(dep4, dep5, dep6) }); var car1 = strategy.CreateCar(typeof(Car1)); var car2 = strategy.CreateCar(typeof(Car2));
This approach provides flexibility and extensibility, allowing easy registration of new factories and streamlined object creation. It effectively separates creation logic from dependencies, simplifying development and maintenance of complex relationships.
The above is the detailed content of How Can We Effectively Manage Dependencies in a Factory Method Pattern with DI/IoC?. For more information, please follow other related articles on the PHP Chinese website!
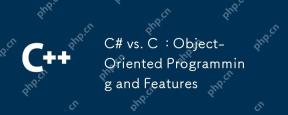
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
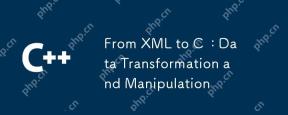
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
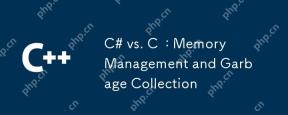
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
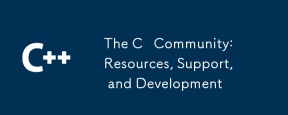
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
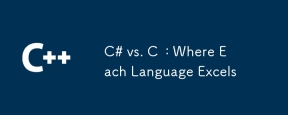
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
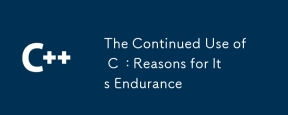
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
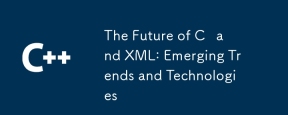
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
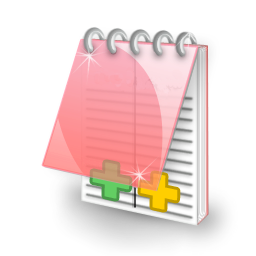
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.