Detailed explanation and common methods of JavaScript arrays:
What is an array?
In JavaScript, an array is a special object used to store a series of values (elements) under a variable name. The values can be of different data types (numbers, strings, booleans, objects, or even other arrays).
Main features:
- Ordered: The elements in the array have a specific order, with their positions indexed starting from 0.
- Mutable: Once an array is created, its elements can be changed.
- Dynamic: Arrays can grow or shrink in size as needed.
Create array:
- Literal representation:
const myArray = [1, "hello", true, null];
- Use Array constructor:
const anotherArray = new Array(5); // 创建一个包含5个空槽的数组 const yetAnotherArray = new Array(1, 2, 3);
Access array elements:
Use square bracket notation and indexing:
const fruits = ["apple", "banana", "orange"]; console.log(fruits[0]); // 输出: "apple" (第一个元素) console.log(fruits[2]); // 输出: "orange" (第三个元素)
Modify array elements:
Assign the new value to the desired index:
fruits[1] = "grape"; console.log(fruits); // 输出: ["apple", "grape", "orange"]
Commonly used array methods:
- push(): Add one or more elements to the end of the array.
fruits.push("mango");
- pop(): Removes the last element of the array and returns it.
const removedFruit = fruits.pop();
- unshift(): Adds one or more elements to the beginning of the array.
fruits.unshift("kiwi");
- shift(): Deletes the first element of the array and returns it.
const firstFruit = fruits.shift();
- slice(): Creates a shallow copy of a portion of the array.
const citrusFruits = fruits.slice(1, 3); // 从索引1到2(不包括2)的元素
- splice(): Add/remove array elements at the specified position.
fruits.splice(1, 0, "pear"); // 在索引1处插入"pear" fruits.splice(2, 1); // 从索引2处删除1个元素
- concat(): Creates a new array by concatenating existing arrays.
const combinedFruits = fruits.concat(["pineapple", "strawberry"]);
- join(): Concatenates all array elements into a string, separated by the specified delimiter.
const fruitString = fruits.join(", ");
- indexOf(): Returns the first index of the given element.
const index = fruits.indexOf("apple");
- includes(): Checks whether the array contains an element.
const hasBanana = fruits.includes("banana");
- forEach(): Execute the provided function once for each array element.
fruits.forEach(fruit => console.log(fruit));
- map(): Creates a new array by applying a function to each element of the original array.
const fruitLengths = fruits.map(fruit => fruit.length);
- filter(): Creates a new array containing only elements that pass the test provided by the function.
const longFruits = fruits.filter(fruit => fruit.length > 5);
This is a basic overview of JavaScript arrays and their methods. There are many other methods available, each with its own specific purpose. Hope this helps!
The above is the detailed content of Explanation on Arrays and Arrays method. For more information, please follow other related articles on the PHP Chinese website!
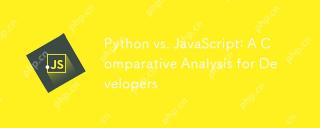
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
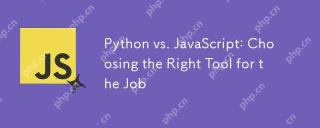
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
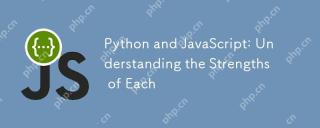
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
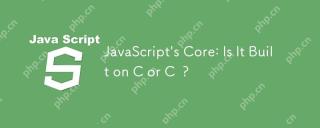
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
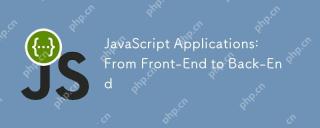
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
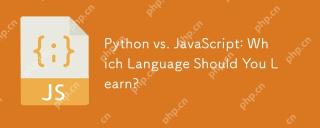
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
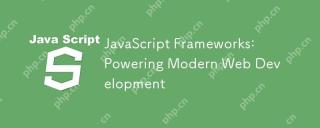
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
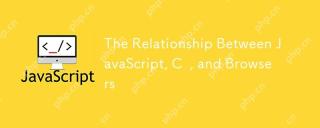
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
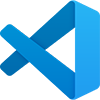
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
