Delete array elements in C#
When working with C# arrays, you may encounter situations where you need to delete specific elements. This article will dive into how to do this effectively.
Determine the elements to be deleted
To remove an element from an array, you must first identify it exactly. Unfortunately, arrays don't natively support retrieving elements by "name". However, you can use other techniques to pinpoint the desired value.
Using LINQ and Loops
If your target framework is .NET Framework 3.5 or higher, LINQ (Language Integrated Query) provides a comprehensive way to filter and modify arrays. Here's how to delete elements using LINQ:
int[] numbers = { 1, 3, 4, 9, 2 }; int numToRemove = 4; numbers = numbers.Where(val => val != numToRemove).ToArray();
Alternatively, for .NET Framework 2.0, you can use a non-LINQ loop:
static bool isNotFour(int n) { return n != 4; } int[] numbers = { 1, 3, 4, 9, 2 }; numbers = Array.FindAll(numbers, isNotFour).ToArray();
Delete first instance
Sometimes, you may only need to remove the first instance of a specific element. In this case, you can modify the LINQ or non-LINQ code as follows:
// LINQ int numToRemove = 4; int numIndex = Array.IndexOf(numbers, numToRemove); numbers = numbers.Where((val, idx) => idx != numIndex).ToArray(); // 非LINQ int numToRemove = 4; int numIdx = Array.IndexOf(numbers, numToRemove); List<int> tmp = new List<int>(numbers); tmp.RemoveAt(numIdx); numbers = tmp.ToArray();
Alternatives to deletion
In some cases, removing elements from an array may not be the most appropriate option. Here are some alternatives to consider:
- Overwrite Element: You can assign a new value to a specific array element, effectively overwriting the existing value.
- Using Linked Lists: Linked lists provide a more flexible structure for deleting elements as it allows you to iterate through the list and find the required value efficiently.
- Create a new array : If the number of elements to be deleted is large, it may be more efficient to create a new array containing the required elements than to modify the existing array.
The above is the detailed content of How Do I Delete an Element from an Array in C#?. For more information, please follow other related articles on the PHP Chinese website!

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
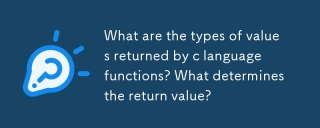
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing
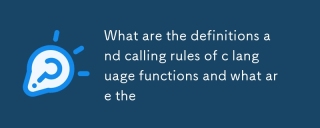
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
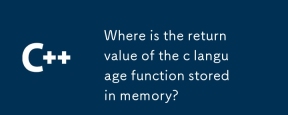
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
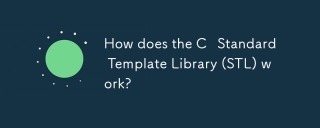
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
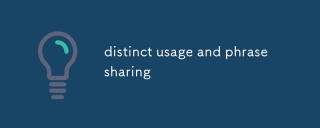
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
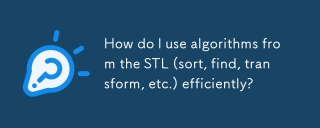
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
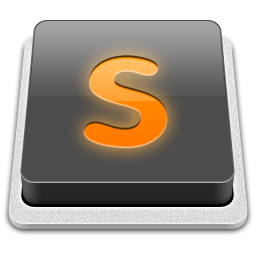
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
