It's been a while since my last IoP update. Let's catch up!
Significant enhancements have been added to the IoP command-line interface:
-
Name Change: The
grongier.pex
module has been renamed toiop
to align with the project's new branding. - Asynchronous Support: IoP now fully supports asynchronous functions and coroutines.
Project Renaming
The grongier.pex
module remains accessible for backward compatibility but will be removed in a future release. Use the iop
module for new development.
Asynchronous Functionalities
While IoP has long supported asynchronous calls, direct use of async functions and coroutines was previously unavailable. Before exploring this new feature, let's review how asynchronous calls function within InterSystems IRIS and examine two examples.
Legacy Asynchronous Calls
This illustrates the traditional approach:
from iop import BusinessProcess from msg import MyMessage class MyBP(BusinessProcess): def on_message(self, request): msg_one = MyMessage(message="Message1") msg_two = MyMessage(message="Message2") self.send_request_async("Python.MyBO", msg_one, completion_key="1") self.send_request_async("Python.MyBO", msg_two, completion_key="2") def on_response(self, request, response, call_request, call_response, completion_key): if completion_key == "1": self.response_one = call_response elif completion_key == "2": self.response_two = call_response def on_complete(self, request, response): self.log_info(f"Received response one: {self.response_one.message}") self.log_info(f"Received response two: {self.response_two.message}")
This mirrors the asynchronous call behavior in IRIS. send_request_async
sends a request to a Business Operation, and on_response
handles the received response. completion_key
differentiates responses.
Synchronous Multi-Request Functionality
While not entirely new, the ability to send multiple synchronous requests concurrently is noteworthy:
from iop import BusinessProcess from msg import MyMessage class MyMultiBP(BusinessProcess): def on_message(self, request): msg_one = MyMessage(message="Message1") msg_two = MyMessage(message="Message2") tuple_responses = self.send_multi_request_sync([("Python.MyMultiBO", msg_one), ("Python.MyMultiBO", msg_two)]) self.log_info("All requests have been processed") for target, request, response, status in tuple_responses: self.log_info(f"Received response: {response.message}")
This example concurrently sends two requests to the same Business Operation. The response is a tuple containing target, request, response, and status for each call. This is particularly useful when request order is unimportant.
Asynchronous Functions and Coroutines
Here's how to leverage async functions and coroutines in IoP:
import asyncio from iop import BusinessProcess from msg import MyMessage class MyAsyncNGBP(BusinessProcess): def on_message(self, request): results = asyncio.run(self.await_response(request)) for result in results: print(f"Received response: {result.message}") async def await_response(self, request): msg_one = MyMessage(message="Message1") msg_two = MyMessage(message="Message2") tasks = [self.send_request_async_ng("Python.MyAsyncNGBO", msg_one), self.send_request_async_ng("Python.MyAsyncNGBO", msg_two)] return await asyncio.gather(*tasks)
This concurrently sends multiple requests using send_request_async_ng
. asyncio.gather
ensures all responses are awaited concurrently.
If you've followed along this far, please comment "Boomerang"! It would mean a lot. Thanks!
await_response
is a coroutine that sends multiple requests and waits for all responses.
The advantages of using async functions and coroutines include improved performance through parallel requests, enhanced readability and maintainability, increased flexibility using the asyncio
module, and better exception and timeout handling.
Comparison of Asynchronous Methods
What are the key differences between send_request_async
, send_multi_request_sync
, and send_request_async_ng
?
-
send_request_async
: Sends a request and waits for a response only ifon_response
is implemented andcompletion_key
is used. Simple but less scalable for parallel requests. -
send_multi_request_sync
: Sends multiple requests concurrently and waits for all responses. Easy to use, but response order isn't guaranteed. -
send_request_async_ng
: Sends multiple requests concurrently and waits for all responses, maintaining response order. Requires async functions and coroutines.
Happy multithreading!
The above is the detailed content of Interoperability On Python update async support. For more information, please follow other related articles on the PHP Chinese website!
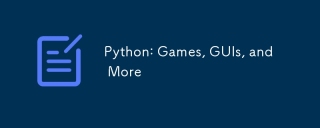
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
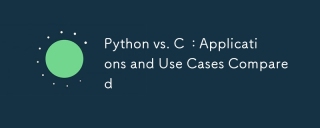
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
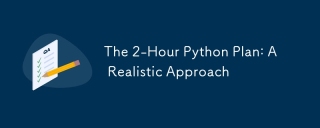
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
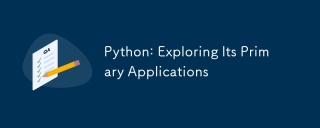
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
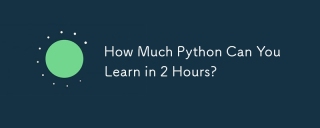
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
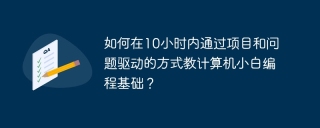
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
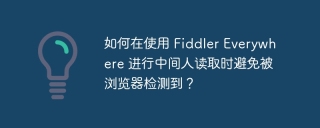
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
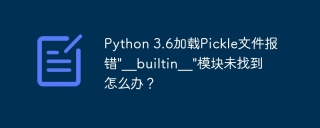
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
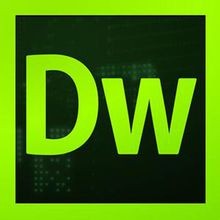
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
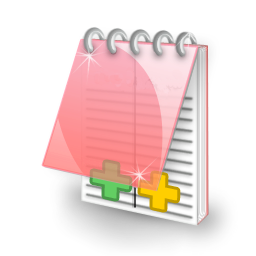
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment
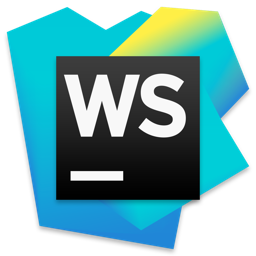
WebStorm Mac version
Useful JavaScript development tools